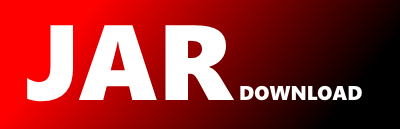
software.amazon.awssdk.services.workdocs.model.Activity Maven / Gradle / Ivy
Show all versions of workdocs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workdocs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the activity information.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Activity implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Activity::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField TIME_STAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(Activity::timeStamp)).setter(setter(Builder::timeStamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeStamp").build()).build();
private static final SdkField IS_INDIRECT_ACTIVITY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(Activity::isIndirectActivity)).setter(setter(Builder::isIndirectActivity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsIndirectActivity").build())
.build();
private static final SdkField ORGANIZATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Activity::organizationId)).setter(setter(Builder::organizationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationId").build()).build();
private static final SdkField INITIATOR_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(Activity::initiator)).setter(setter(Builder::initiator)).constructor(UserMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Initiator").build()).build();
private static final SdkField PARTICIPANTS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(Activity::participants)).setter(setter(Builder::participants)).constructor(Participants::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Participants").build()).build();
private static final SdkField RESOURCE_METADATA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Activity::resourceMetadata))
.setter(setter(Builder::resourceMetadata)).constructor(ResourceMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceMetadata").build()).build();
private static final SdkField ORIGINAL_PARENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Activity::originalParent))
.setter(setter(Builder::originalParent)).constructor(ResourceMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OriginalParent").build()).build();
private static final SdkField COMMENT_METADATA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Activity::commentMetadata))
.setter(setter(Builder::commentMetadata)).constructor(CommentMetadata::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CommentMetadata").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD, TIME_STAMP_FIELD,
IS_INDIRECT_ACTIVITY_FIELD, ORGANIZATION_ID_FIELD, INITIATOR_FIELD, PARTICIPANTS_FIELD, RESOURCE_METADATA_FIELD,
ORIGINAL_PARENT_FIELD, COMMENT_METADATA_FIELD));
private static final long serialVersionUID = 1L;
private final String type;
private final Instant timeStamp;
private final Boolean isIndirectActivity;
private final String organizationId;
private final UserMetadata initiator;
private final Participants participants;
private final ResourceMetadata resourceMetadata;
private final ResourceMetadata originalParent;
private final CommentMetadata commentMetadata;
private Activity(BuilderImpl builder) {
this.type = builder.type;
this.timeStamp = builder.timeStamp;
this.isIndirectActivity = builder.isIndirectActivity;
this.organizationId = builder.organizationId;
this.initiator = builder.initiator;
this.participants = builder.participants;
this.resourceMetadata = builder.resourceMetadata;
this.originalParent = builder.originalParent;
this.commentMetadata = builder.commentMetadata;
}
/**
*
* The activity type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ActivityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The activity type.
* @see ActivityType
*/
public ActivityType type() {
return ActivityType.fromValue(type);
}
/**
*
* The activity type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ActivityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The activity type.
* @see ActivityType
*/
public String typeAsString() {
return type;
}
/**
*
* The timestamp when the action was performed.
*
*
* @return The timestamp when the action was performed.
*/
public Instant timeStamp() {
return timeStamp;
}
/**
*
* Indicates whether an activity is indirect or direct. An indirect activity results from a direct activity
* performed on a parent resource. For example, sharing a parent folder (the direct activity) shares all of the
* subfolders and documents within the parent folder (the indirect activity).
*
*
* @return Indicates whether an activity is indirect or direct. An indirect activity results from a direct activity
* performed on a parent resource. For example, sharing a parent folder (the direct activity) shares all of
* the subfolders and documents within the parent folder (the indirect activity).
*/
public Boolean isIndirectActivity() {
return isIndirectActivity;
}
/**
*
* The ID of the organization.
*
*
* @return The ID of the organization.
*/
public String organizationId() {
return organizationId;
}
/**
*
* The user who performed the action.
*
*
* @return The user who performed the action.
*/
public UserMetadata initiator() {
return initiator;
}
/**
*
* The list of users or groups impacted by this action. This is an optional field and is filled for the following
* sharing activities: DOCUMENT_SHARED, DOCUMENT_SHARED, DOCUMENT_UNSHARED, FOLDER_SHARED, FOLDER_UNSHARED.
*
*
* @return The list of users or groups impacted by this action. This is an optional field and is filled for the
* following sharing activities: DOCUMENT_SHARED, DOCUMENT_SHARED, DOCUMENT_UNSHARED, FOLDER_SHARED,
* FOLDER_UNSHARED.
*/
public Participants participants() {
return participants;
}
/**
*
* The metadata of the resource involved in the user action.
*
*
* @return The metadata of the resource involved in the user action.
*/
public ResourceMetadata resourceMetadata() {
return resourceMetadata;
}
/**
*
* The original parent of the resource. This is an optional field and is filled for move activities.
*
*
* @return The original parent of the resource. This is an optional field and is filled for move activities.
*/
public ResourceMetadata originalParent() {
return originalParent;
}
/**
*
* Metadata of the commenting activity. This is an optional field and is filled for commenting activities.
*
*
* @return Metadata of the commenting activity. This is an optional field and is filled for commenting activities.
*/
public CommentMetadata commentMetadata() {
return commentMetadata;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(timeStamp());
hashCode = 31 * hashCode + Objects.hashCode(isIndirectActivity());
hashCode = 31 * hashCode + Objects.hashCode(organizationId());
hashCode = 31 * hashCode + Objects.hashCode(initiator());
hashCode = 31 * hashCode + Objects.hashCode(participants());
hashCode = 31 * hashCode + Objects.hashCode(resourceMetadata());
hashCode = 31 * hashCode + Objects.hashCode(originalParent());
hashCode = 31 * hashCode + Objects.hashCode(commentMetadata());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Activity)) {
return false;
}
Activity other = (Activity) obj;
return Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(timeStamp(), other.timeStamp())
&& Objects.equals(isIndirectActivity(), other.isIndirectActivity())
&& Objects.equals(organizationId(), other.organizationId()) && Objects.equals(initiator(), other.initiator())
&& Objects.equals(participants(), other.participants())
&& Objects.equals(resourceMetadata(), other.resourceMetadata())
&& Objects.equals(originalParent(), other.originalParent())
&& Objects.equals(commentMetadata(), other.commentMetadata());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Activity").add("Type", typeAsString()).add("TimeStamp", timeStamp())
.add("IsIndirectActivity", isIndirectActivity()).add("OrganizationId", organizationId())
.add("Initiator", initiator()).add("Participants", participants()).add("ResourceMetadata", resourceMetadata())
.add("OriginalParent", originalParent()).add("CommentMetadata", commentMetadata()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "TimeStamp":
return Optional.ofNullable(clazz.cast(timeStamp()));
case "IsIndirectActivity":
return Optional.ofNullable(clazz.cast(isIndirectActivity()));
case "OrganizationId":
return Optional.ofNullable(clazz.cast(organizationId()));
case "Initiator":
return Optional.ofNullable(clazz.cast(initiator()));
case "Participants":
return Optional.ofNullable(clazz.cast(participants()));
case "ResourceMetadata":
return Optional.ofNullable(clazz.cast(resourceMetadata()));
case "OriginalParent":
return Optional.ofNullable(clazz.cast(originalParent()));
case "CommentMetadata":
return Optional.ofNullable(clazz.cast(commentMetadata()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function