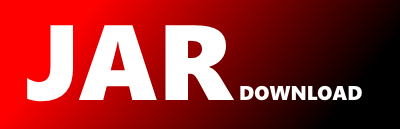
software.amazon.awssdk.services.workmail.model.AccessControlRule Maven / Gradle / Ivy
Show all versions of workmail Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workmail.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A rule that controls access to an WorkMail organization.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AccessControlRule implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(AccessControlRule::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField EFFECT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Effect")
.getter(getter(AccessControlRule::effectAsString)).setter(setter(Builder::effect))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Effect").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(AccessControlRule::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField> IP_RANGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("IpRanges")
.getter(getter(AccessControlRule::ipRanges))
.setter(setter(Builder::ipRanges))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpRanges").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NOT_IP_RANGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotIpRanges")
.getter(getter(AccessControlRule::notIpRanges))
.setter(setter(Builder::notIpRanges))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotIpRanges").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Actions")
.getter(getter(AccessControlRule::actions))
.setter(setter(Builder::actions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Actions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NOT_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotActions")
.getter(getter(AccessControlRule::notActions))
.setter(setter(Builder::notActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> USER_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("UserIds")
.getter(getter(AccessControlRule::userIds))
.setter(setter(Builder::userIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UserIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NOT_USER_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotUserIds")
.getter(getter(AccessControlRule::notUserIds))
.setter(setter(Builder::notUserIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotUserIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DATE_CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("DateCreated").getter(getter(AccessControlRule::dateCreated)).setter(setter(Builder::dateCreated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DateCreated").build()).build();
private static final SdkField DATE_MODIFIED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("DateModified").getter(getter(AccessControlRule::dateModified)).setter(setter(Builder::dateModified))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DateModified").build()).build();
private static final SdkField> IMPERSONATION_ROLE_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ImpersonationRoleIds")
.getter(getter(AccessControlRule::impersonationRoleIds))
.setter(setter(Builder::impersonationRoleIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ImpersonationRoleIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NOT_IMPERSONATION_ROLE_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotImpersonationRoleIds")
.getter(getter(AccessControlRule::notImpersonationRoleIds))
.setter(setter(Builder::notImpersonationRoleIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotImpersonationRoleIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, EFFECT_FIELD,
DESCRIPTION_FIELD, IP_RANGES_FIELD, NOT_IP_RANGES_FIELD, ACTIONS_FIELD, NOT_ACTIONS_FIELD, USER_IDS_FIELD,
NOT_USER_IDS_FIELD, DATE_CREATED_FIELD, DATE_MODIFIED_FIELD, IMPERSONATION_ROLE_IDS_FIELD,
NOT_IMPERSONATION_ROLE_IDS_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final String effect;
private final String description;
private final List ipRanges;
private final List notIpRanges;
private final List actions;
private final List notActions;
private final List userIds;
private final List notUserIds;
private final Instant dateCreated;
private final Instant dateModified;
private final List impersonationRoleIds;
private final List notImpersonationRoleIds;
private AccessControlRule(BuilderImpl builder) {
this.name = builder.name;
this.effect = builder.effect;
this.description = builder.description;
this.ipRanges = builder.ipRanges;
this.notIpRanges = builder.notIpRanges;
this.actions = builder.actions;
this.notActions = builder.notActions;
this.userIds = builder.userIds;
this.notUserIds = builder.notUserIds;
this.dateCreated = builder.dateCreated;
this.dateModified = builder.dateModified;
this.impersonationRoleIds = builder.impersonationRoleIds;
this.notImpersonationRoleIds = builder.notImpersonationRoleIds;
}
/**
*
* The rule name.
*
*
* @return The rule name.
*/
public final String name() {
return name;
}
/**
*
* The rule effect.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #effect} will
* return {@link AccessControlRuleEffect#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #effectAsString}.
*
*
* @return The rule effect.
* @see AccessControlRuleEffect
*/
public final AccessControlRuleEffect effect() {
return AccessControlRuleEffect.fromValue(effect);
}
/**
*
* The rule effect.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #effect} will
* return {@link AccessControlRuleEffect#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #effectAsString}.
*
*
* @return The rule effect.
* @see AccessControlRuleEffect
*/
public final String effectAsString() {
return effect;
}
/**
*
* The rule description.
*
*
* @return The rule description.
*/
public final String description() {
return description;
}
/**
* For responses, this returns true if the service returned a value for the IpRanges property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasIpRanges() {
return ipRanges != null && !(ipRanges instanceof SdkAutoConstructList);
}
/**
*
* IPv4 CIDR ranges to include in the rule.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIpRanges} method.
*
*
* @return IPv4 CIDR ranges to include in the rule.
*/
public final List ipRanges() {
return ipRanges;
}
/**
* For responses, this returns true if the service returned a value for the NotIpRanges property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNotIpRanges() {
return notIpRanges != null && !(notIpRanges instanceof SdkAutoConstructList);
}
/**
*
* IPv4 CIDR ranges to exclude from the rule.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotIpRanges} method.
*
*
* @return IPv4 CIDR ranges to exclude from the rule.
*/
public final List notIpRanges() {
return notIpRanges;
}
/**
* For responses, this returns true if the service returned a value for the Actions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasActions() {
return actions != null && !(actions instanceof SdkAutoConstructList);
}
/**
*
* Access protocol actions to include in the rule. Valid values include ActiveSync
,
* AutoDiscover
, EWS
, IMAP
, SMTP
, WindowsOutlook
,
* and WebMail
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasActions} method.
*
*
* @return Access protocol actions to include in the rule. Valid values include ActiveSync
,
* AutoDiscover
, EWS
, IMAP
, SMTP
,
* WindowsOutlook
, and WebMail
.
*/
public final List actions() {
return actions;
}
/**
* For responses, this returns true if the service returned a value for the NotActions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasNotActions() {
return notActions != null && !(notActions instanceof SdkAutoConstructList);
}
/**
*
* Access protocol actions to exclude from the rule. Valid values include ActiveSync
,
* AutoDiscover
, EWS
, IMAP
, SMTP
, WindowsOutlook
,
* and WebMail
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotActions} method.
*
*
* @return Access protocol actions to exclude from the rule. Valid values include ActiveSync
,
* AutoDiscover
, EWS
, IMAP
, SMTP
,
* WindowsOutlook
, and WebMail
.
*/
public final List notActions() {
return notActions;
}
/**
* For responses, this returns true if the service returned a value for the UserIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasUserIds() {
return userIds != null && !(userIds instanceof SdkAutoConstructList);
}
/**
*
* User IDs to include in the rule.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasUserIds} method.
*
*
* @return User IDs to include in the rule.
*/
public final List userIds() {
return userIds;
}
/**
* For responses, this returns true if the service returned a value for the NotUserIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasNotUserIds() {
return notUserIds != null && !(notUserIds instanceof SdkAutoConstructList);
}
/**
*
* User IDs to exclude from the rule.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotUserIds} method.
*
*
* @return User IDs to exclude from the rule.
*/
public final List notUserIds() {
return notUserIds;
}
/**
*
* The date that the rule was created.
*
*
* @return The date that the rule was created.
*/
public final Instant dateCreated() {
return dateCreated;
}
/**
*
* The date that the rule was modified.
*
*
* @return The date that the rule was modified.
*/
public final Instant dateModified() {
return dateModified;
}
/**
* For responses, this returns true if the service returned a value for the ImpersonationRoleIds property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasImpersonationRoleIds() {
return impersonationRoleIds != null && !(impersonationRoleIds instanceof SdkAutoConstructList);
}
/**
*
* Impersonation role IDs to include in the rule.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasImpersonationRoleIds} method.
*
*
* @return Impersonation role IDs to include in the rule.
*/
public final List impersonationRoleIds() {
return impersonationRoleIds;
}
/**
* For responses, this returns true if the service returned a value for the NotImpersonationRoleIds property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasNotImpersonationRoleIds() {
return notImpersonationRoleIds != null && !(notImpersonationRoleIds instanceof SdkAutoConstructList);
}
/**
*
* Impersonation role IDs to exclude from the rule.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotImpersonationRoleIds} method.
*
*
* @return Impersonation role IDs to exclude from the rule.
*/
public final List notImpersonationRoleIds() {
return notImpersonationRoleIds;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(effectAsString());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(hasIpRanges() ? ipRanges() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNotIpRanges() ? notIpRanges() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasActions() ? actions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNotActions() ? notActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasUserIds() ? userIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNotUserIds() ? notUserIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(dateCreated());
hashCode = 31 * hashCode + Objects.hashCode(dateModified());
hashCode = 31 * hashCode + Objects.hashCode(hasImpersonationRoleIds() ? impersonationRoleIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNotImpersonationRoleIds() ? notImpersonationRoleIds() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AccessControlRule)) {
return false;
}
AccessControlRule other = (AccessControlRule) obj;
return Objects.equals(name(), other.name()) && Objects.equals(effectAsString(), other.effectAsString())
&& Objects.equals(description(), other.description()) && hasIpRanges() == other.hasIpRanges()
&& Objects.equals(ipRanges(), other.ipRanges()) && hasNotIpRanges() == other.hasNotIpRanges()
&& Objects.equals(notIpRanges(), other.notIpRanges()) && hasActions() == other.hasActions()
&& Objects.equals(actions(), other.actions()) && hasNotActions() == other.hasNotActions()
&& Objects.equals(notActions(), other.notActions()) && hasUserIds() == other.hasUserIds()
&& Objects.equals(userIds(), other.userIds()) && hasNotUserIds() == other.hasNotUserIds()
&& Objects.equals(notUserIds(), other.notUserIds()) && Objects.equals(dateCreated(), other.dateCreated())
&& Objects.equals(dateModified(), other.dateModified())
&& hasImpersonationRoleIds() == other.hasImpersonationRoleIds()
&& Objects.equals(impersonationRoleIds(), other.impersonationRoleIds())
&& hasNotImpersonationRoleIds() == other.hasNotImpersonationRoleIds()
&& Objects.equals(notImpersonationRoleIds(), other.notImpersonationRoleIds());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AccessControlRule").add("Name", name()).add("Effect", effectAsString())
.add("Description", description()).add("IpRanges", hasIpRanges() ? ipRanges() : null)
.add("NotIpRanges", hasNotIpRanges() ? notIpRanges() : null).add("Actions", hasActions() ? actions() : null)
.add("NotActions", hasNotActions() ? notActions() : null).add("UserIds", hasUserIds() ? userIds() : null)
.add("NotUserIds", hasNotUserIds() ? notUserIds() : null).add("DateCreated", dateCreated())
.add("DateModified", dateModified())
.add("ImpersonationRoleIds", hasImpersonationRoleIds() ? impersonationRoleIds() : null)
.add("NotImpersonationRoleIds", hasNotImpersonationRoleIds() ? notImpersonationRoleIds() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Effect":
return Optional.ofNullable(clazz.cast(effectAsString()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "IpRanges":
return Optional.ofNullable(clazz.cast(ipRanges()));
case "NotIpRanges":
return Optional.ofNullable(clazz.cast(notIpRanges()));
case "Actions":
return Optional.ofNullable(clazz.cast(actions()));
case "NotActions":
return Optional.ofNullable(clazz.cast(notActions()));
case "UserIds":
return Optional.ofNullable(clazz.cast(userIds()));
case "NotUserIds":
return Optional.ofNullable(clazz.cast(notUserIds()));
case "DateCreated":
return Optional.ofNullable(clazz.cast(dateCreated()));
case "DateModified":
return Optional.ofNullable(clazz.cast(dateModified()));
case "ImpersonationRoleIds":
return Optional.ofNullable(clazz.cast(impersonationRoleIds()));
case "NotImpersonationRoleIds":
return Optional.ofNullable(clazz.cast(notImpersonationRoleIds()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function