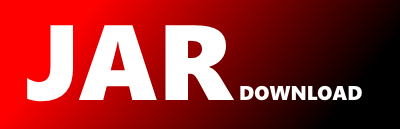
software.amazon.awssdk.services.workmail.model.CreateMobileDeviceAccessRuleRequest Maven / Gradle / Ivy
Show all versions of workmail Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workmail.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateMobileDeviceAccessRuleRequest extends WorkMailRequest implements
ToCopyableBuilder {
private static final SdkField ORGANIZATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OrganizationId").getter(getter(CreateMobileDeviceAccessRuleRequest::organizationId))
.setter(setter(Builder::organizationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationId").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientToken")
.getter(getter(CreateMobileDeviceAccessRuleRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(CreateMobileDeviceAccessRuleRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateMobileDeviceAccessRuleRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField EFFECT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Effect")
.getter(getter(CreateMobileDeviceAccessRuleRequest::effectAsString)).setter(setter(Builder::effect))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Effect").build()).build();
private static final SdkField> DEVICE_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DeviceTypes")
.getter(getter(CreateMobileDeviceAccessRuleRequest::deviceTypes))
.setter(setter(Builder::deviceTypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeviceTypes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NOT_DEVICE_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotDeviceTypes")
.getter(getter(CreateMobileDeviceAccessRuleRequest::notDeviceTypes))
.setter(setter(Builder::notDeviceTypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotDeviceTypes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DEVICE_MODELS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DeviceModels")
.getter(getter(CreateMobileDeviceAccessRuleRequest::deviceModels))
.setter(setter(Builder::deviceModels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeviceModels").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NOT_DEVICE_MODELS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotDeviceModels")
.getter(getter(CreateMobileDeviceAccessRuleRequest::notDeviceModels))
.setter(setter(Builder::notDeviceModels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotDeviceModels").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DEVICE_OPERATING_SYSTEMS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DeviceOperatingSystems")
.getter(getter(CreateMobileDeviceAccessRuleRequest::deviceOperatingSystems))
.setter(setter(Builder::deviceOperatingSystems))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeviceOperatingSystems").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NOT_DEVICE_OPERATING_SYSTEMS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotDeviceOperatingSystems")
.getter(getter(CreateMobileDeviceAccessRuleRequest::notDeviceOperatingSystems))
.setter(setter(Builder::notDeviceOperatingSystems))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotDeviceOperatingSystems").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DEVICE_USER_AGENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DeviceUserAgents")
.getter(getter(CreateMobileDeviceAccessRuleRequest::deviceUserAgents))
.setter(setter(Builder::deviceUserAgents))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeviceUserAgents").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NOT_DEVICE_USER_AGENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NotDeviceUserAgents")
.getter(getter(CreateMobileDeviceAccessRuleRequest::notDeviceUserAgents))
.setter(setter(Builder::notDeviceUserAgents))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotDeviceUserAgents").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ORGANIZATION_ID_FIELD,
CLIENT_TOKEN_FIELD, NAME_FIELD, DESCRIPTION_FIELD, EFFECT_FIELD, DEVICE_TYPES_FIELD, NOT_DEVICE_TYPES_FIELD,
DEVICE_MODELS_FIELD, NOT_DEVICE_MODELS_FIELD, DEVICE_OPERATING_SYSTEMS_FIELD, NOT_DEVICE_OPERATING_SYSTEMS_FIELD,
DEVICE_USER_AGENTS_FIELD, NOT_DEVICE_USER_AGENTS_FIELD));
private final String organizationId;
private final String clientToken;
private final String name;
private final String description;
private final String effect;
private final List deviceTypes;
private final List notDeviceTypes;
private final List deviceModels;
private final List notDeviceModels;
private final List deviceOperatingSystems;
private final List notDeviceOperatingSystems;
private final List deviceUserAgents;
private final List notDeviceUserAgents;
private CreateMobileDeviceAccessRuleRequest(BuilderImpl builder) {
super(builder);
this.organizationId = builder.organizationId;
this.clientToken = builder.clientToken;
this.name = builder.name;
this.description = builder.description;
this.effect = builder.effect;
this.deviceTypes = builder.deviceTypes;
this.notDeviceTypes = builder.notDeviceTypes;
this.deviceModels = builder.deviceModels;
this.notDeviceModels = builder.notDeviceModels;
this.deviceOperatingSystems = builder.deviceOperatingSystems;
this.notDeviceOperatingSystems = builder.notDeviceOperatingSystems;
this.deviceUserAgents = builder.deviceUserAgents;
this.notDeviceUserAgents = builder.notDeviceUserAgents;
}
/**
*
* The WorkMail organization under which the rule will be created.
*
*
* @return The WorkMail organization under which the rule will be created.
*/
public final String organizationId() {
return organizationId;
}
/**
*
* The idempotency token for the client request.
*
*
* @return The idempotency token for the client request.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* The rule name.
*
*
* @return The rule name.
*/
public final String name() {
return name;
}
/**
*
* The rule description.
*
*
* @return The rule description.
*/
public final String description() {
return description;
}
/**
*
* The effect of the rule when it matches. Allowed values are ALLOW
or DENY
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #effect} will
* return {@link MobileDeviceAccessRuleEffect#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #effectAsString}.
*
*
* @return The effect of the rule when it matches. Allowed values are ALLOW
or DENY
.
* @see MobileDeviceAccessRuleEffect
*/
public final MobileDeviceAccessRuleEffect effect() {
return MobileDeviceAccessRuleEffect.fromValue(effect);
}
/**
*
* The effect of the rule when it matches. Allowed values are ALLOW
or DENY
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #effect} will
* return {@link MobileDeviceAccessRuleEffect#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #effectAsString}.
*
*
* @return The effect of the rule when it matches. Allowed values are ALLOW
or DENY
.
* @see MobileDeviceAccessRuleEffect
*/
public final String effectAsString() {
return effect;
}
/**
* For responses, this returns true if the service returned a value for the DeviceTypes property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDeviceTypes() {
return deviceTypes != null && !(deviceTypes instanceof SdkAutoConstructList);
}
/**
*
* Device types that the rule will match.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDeviceTypes} method.
*
*
* @return Device types that the rule will match.
*/
public final List deviceTypes() {
return deviceTypes;
}
/**
* For responses, this returns true if the service returned a value for the NotDeviceTypes property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNotDeviceTypes() {
return notDeviceTypes != null && !(notDeviceTypes instanceof SdkAutoConstructList);
}
/**
*
* Device types that the rule will not match. All other device types will match.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotDeviceTypes} method.
*
*
* @return Device types that the rule will not match. All other device types will match.
*/
public final List notDeviceTypes() {
return notDeviceTypes;
}
/**
* For responses, this returns true if the service returned a value for the DeviceModels property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDeviceModels() {
return deviceModels != null && !(deviceModels instanceof SdkAutoConstructList);
}
/**
*
* Device models that the rule will match.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDeviceModels} method.
*
*
* @return Device models that the rule will match.
*/
public final List deviceModels() {
return deviceModels;
}
/**
* For responses, this returns true if the service returned a value for the NotDeviceModels property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNotDeviceModels() {
return notDeviceModels != null && !(notDeviceModels instanceof SdkAutoConstructList);
}
/**
*
* Device models that the rule will not match. All other device models will match.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotDeviceModels} method.
*
*
* @return Device models that the rule will not match. All other device models will match.
*/
public final List notDeviceModels() {
return notDeviceModels;
}
/**
* For responses, this returns true if the service returned a value for the DeviceOperatingSystems property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasDeviceOperatingSystems() {
return deviceOperatingSystems != null && !(deviceOperatingSystems instanceof SdkAutoConstructList);
}
/**
*
* Device operating systems that the rule will match.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDeviceOperatingSystems} method.
*
*
* @return Device operating systems that the rule will match.
*/
public final List deviceOperatingSystems() {
return deviceOperatingSystems;
}
/**
* For responses, this returns true if the service returned a value for the NotDeviceOperatingSystems property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasNotDeviceOperatingSystems() {
return notDeviceOperatingSystems != null && !(notDeviceOperatingSystems instanceof SdkAutoConstructList);
}
/**
*
* Device operating systems that the rule will not match. All other device operating systems will match.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotDeviceOperatingSystems} method.
*
*
* @return Device operating systems that the rule will not match. All other device operating systems will
* match.
*/
public final List notDeviceOperatingSystems() {
return notDeviceOperatingSystems;
}
/**
* For responses, this returns true if the service returned a value for the DeviceUserAgents property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDeviceUserAgents() {
return deviceUserAgents != null && !(deviceUserAgents instanceof SdkAutoConstructList);
}
/**
*
* Device user agents that the rule will match.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDeviceUserAgents} method.
*
*
* @return Device user agents that the rule will match.
*/
public final List deviceUserAgents() {
return deviceUserAgents;
}
/**
* For responses, this returns true if the service returned a value for the NotDeviceUserAgents property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNotDeviceUserAgents() {
return notDeviceUserAgents != null && !(notDeviceUserAgents instanceof SdkAutoConstructList);
}
/**
*
* Device user agents that the rule will not match. All other device user agents will match.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNotDeviceUserAgents} method.
*
*
* @return Device user agents that the rule will not match. All other device user agents will match.
*/
public final List notDeviceUserAgents() {
return notDeviceUserAgents;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(organizationId());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(effectAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasDeviceTypes() ? deviceTypes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNotDeviceTypes() ? notDeviceTypes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDeviceModels() ? deviceModels() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNotDeviceModels() ? notDeviceModels() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDeviceOperatingSystems() ? deviceOperatingSystems() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNotDeviceOperatingSystems() ? notDeviceOperatingSystems() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDeviceUserAgents() ? deviceUserAgents() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNotDeviceUserAgents() ? notDeviceUserAgents() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateMobileDeviceAccessRuleRequest)) {
return false;
}
CreateMobileDeviceAccessRuleRequest other = (CreateMobileDeviceAccessRuleRequest) obj;
return Objects.equals(organizationId(), other.organizationId()) && Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(name(), other.name()) && Objects.equals(description(), other.description())
&& Objects.equals(effectAsString(), other.effectAsString()) && hasDeviceTypes() == other.hasDeviceTypes()
&& Objects.equals(deviceTypes(), other.deviceTypes()) && hasNotDeviceTypes() == other.hasNotDeviceTypes()
&& Objects.equals(notDeviceTypes(), other.notDeviceTypes()) && hasDeviceModels() == other.hasDeviceModels()
&& Objects.equals(deviceModels(), other.deviceModels()) && hasNotDeviceModels() == other.hasNotDeviceModels()
&& Objects.equals(notDeviceModels(), other.notDeviceModels())
&& hasDeviceOperatingSystems() == other.hasDeviceOperatingSystems()
&& Objects.equals(deviceOperatingSystems(), other.deviceOperatingSystems())
&& hasNotDeviceOperatingSystems() == other.hasNotDeviceOperatingSystems()
&& Objects.equals(notDeviceOperatingSystems(), other.notDeviceOperatingSystems())
&& hasDeviceUserAgents() == other.hasDeviceUserAgents()
&& Objects.equals(deviceUserAgents(), other.deviceUserAgents())
&& hasNotDeviceUserAgents() == other.hasNotDeviceUserAgents()
&& Objects.equals(notDeviceUserAgents(), other.notDeviceUserAgents());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateMobileDeviceAccessRuleRequest").add("OrganizationId", organizationId())
.add("ClientToken", clientToken()).add("Name", name()).add("Description", description())
.add("Effect", effectAsString()).add("DeviceTypes", hasDeviceTypes() ? deviceTypes() : null)
.add("NotDeviceTypes", hasNotDeviceTypes() ? notDeviceTypes() : null)
.add("DeviceModels", hasDeviceModels() ? deviceModels() : null)
.add("NotDeviceModels", hasNotDeviceModels() ? notDeviceModels() : null)
.add("DeviceOperatingSystems", hasDeviceOperatingSystems() ? deviceOperatingSystems() : null)
.add("NotDeviceOperatingSystems", hasNotDeviceOperatingSystems() ? notDeviceOperatingSystems() : null)
.add("DeviceUserAgents", hasDeviceUserAgents() ? deviceUserAgents() : null)
.add("NotDeviceUserAgents", hasNotDeviceUserAgents() ? notDeviceUserAgents() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "OrganizationId":
return Optional.ofNullable(clazz.cast(organizationId()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Effect":
return Optional.ofNullable(clazz.cast(effectAsString()));
case "DeviceTypes":
return Optional.ofNullable(clazz.cast(deviceTypes()));
case "NotDeviceTypes":
return Optional.ofNullable(clazz.cast(notDeviceTypes()));
case "DeviceModels":
return Optional.ofNullable(clazz.cast(deviceModels()));
case "NotDeviceModels":
return Optional.ofNullable(clazz.cast(notDeviceModels()));
case "DeviceOperatingSystems":
return Optional.ofNullable(clazz.cast(deviceOperatingSystems()));
case "NotDeviceOperatingSystems":
return Optional.ofNullable(clazz.cast(notDeviceOperatingSystems()));
case "DeviceUserAgents":
return Optional.ofNullable(clazz.cast(deviceUserAgents()));
case "NotDeviceUserAgents":
return Optional.ofNullable(clazz.cast(notDeviceUserAgents()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function