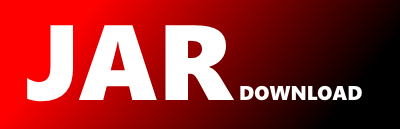
software.amazon.awssdk.services.workspaces.model.ApplicationResourceAssociation Maven / Gradle / Ivy
Show all versions of workspaces Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workspaces.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the association between an application and an application resource.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ApplicationResourceAssociation implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField APPLICATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationId").getter(getter(ApplicationResourceAssociation::applicationId))
.setter(setter(Builder::applicationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationId").build()).build();
private static final SdkField ASSOCIATED_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AssociatedResourceId").getter(getter(ApplicationResourceAssociation::associatedResourceId))
.setter(setter(Builder::associatedResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssociatedResourceId").build())
.build();
private static final SdkField ASSOCIATED_RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AssociatedResourceType").getter(getter(ApplicationResourceAssociation::associatedResourceTypeAsString))
.setter(setter(Builder::associatedResourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssociatedResourceType").build())
.build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("Created").getter(getter(ApplicationResourceAssociation::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Created").build()).build();
private static final SdkField LAST_UPDATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastUpdatedTime").getter(getter(ApplicationResourceAssociation::lastUpdatedTime))
.setter(setter(Builder::lastUpdatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdatedTime").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("State")
.getter(getter(ApplicationResourceAssociation::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField STATE_REASON_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StateReason")
.getter(getter(ApplicationResourceAssociation::stateReason)).setter(setter(Builder::stateReason))
.constructor(AssociationStateReason::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StateReason").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_ID_FIELD,
ASSOCIATED_RESOURCE_ID_FIELD, ASSOCIATED_RESOURCE_TYPE_FIELD, CREATED_FIELD, LAST_UPDATED_TIME_FIELD, STATE_FIELD,
STATE_REASON_FIELD));
private static final long serialVersionUID = 1L;
private final String applicationId;
private final String associatedResourceId;
private final String associatedResourceType;
private final Instant created;
private final Instant lastUpdatedTime;
private final String state;
private final AssociationStateReason stateReason;
private ApplicationResourceAssociation(BuilderImpl builder) {
this.applicationId = builder.applicationId;
this.associatedResourceId = builder.associatedResourceId;
this.associatedResourceType = builder.associatedResourceType;
this.created = builder.created;
this.lastUpdatedTime = builder.lastUpdatedTime;
this.state = builder.state;
this.stateReason = builder.stateReason;
}
/**
*
* The identifier of the application.
*
*
* @return The identifier of the application.
*/
public final String applicationId() {
return applicationId;
}
/**
*
* The identifier of the associated resource.
*
*
* @return The identifier of the associated resource.
*/
public final String associatedResourceId() {
return associatedResourceId;
}
/**
*
* The resource type of the associated resource.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #associatedResourceType} will return {@link ApplicationAssociatedResourceType#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #associatedResourceTypeAsString}.
*
*
* @return The resource type of the associated resource.
* @see ApplicationAssociatedResourceType
*/
public final ApplicationAssociatedResourceType associatedResourceType() {
return ApplicationAssociatedResourceType.fromValue(associatedResourceType);
}
/**
*
* The resource type of the associated resource.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #associatedResourceType} will return {@link ApplicationAssociatedResourceType#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #associatedResourceTypeAsString}.
*
*
* @return The resource type of the associated resource.
* @see ApplicationAssociatedResourceType
*/
public final String associatedResourceTypeAsString() {
return associatedResourceType;
}
/**
*
* The time the association was created.
*
*
* @return The time the association was created.
*/
public final Instant created() {
return created;
}
/**
*
* The time the association status was last updated.
*
*
* @return The time the association status was last updated.
*/
public final Instant lastUpdatedTime() {
return lastUpdatedTime;
}
/**
*
* The status of the application resource association.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link AssociationState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The status of the application resource association.
* @see AssociationState
*/
public final AssociationState state() {
return AssociationState.fromValue(state);
}
/**
*
* The status of the application resource association.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link AssociationState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The status of the application resource association.
* @see AssociationState
*/
public final String stateAsString() {
return state;
}
/**
*
* The reason the association deployment failed.
*
*
* @return The reason the association deployment failed.
*/
public final AssociationStateReason stateReason() {
return stateReason;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(applicationId());
hashCode = 31 * hashCode + Objects.hashCode(associatedResourceId());
hashCode = 31 * hashCode + Objects.hashCode(associatedResourceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedTime());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateReason());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ApplicationResourceAssociation)) {
return false;
}
ApplicationResourceAssociation other = (ApplicationResourceAssociation) obj;
return Objects.equals(applicationId(), other.applicationId())
&& Objects.equals(associatedResourceId(), other.associatedResourceId())
&& Objects.equals(associatedResourceTypeAsString(), other.associatedResourceTypeAsString())
&& Objects.equals(created(), other.created()) && Objects.equals(lastUpdatedTime(), other.lastUpdatedTime())
&& Objects.equals(stateAsString(), other.stateAsString()) && Objects.equals(stateReason(), other.stateReason());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ApplicationResourceAssociation").add("ApplicationId", applicationId())
.add("AssociatedResourceId", associatedResourceId())
.add("AssociatedResourceType", associatedResourceTypeAsString()).add("Created", created())
.add("LastUpdatedTime", lastUpdatedTime()).add("State", stateAsString()).add("StateReason", stateReason())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationId":
return Optional.ofNullable(clazz.cast(applicationId()));
case "AssociatedResourceId":
return Optional.ofNullable(clazz.cast(associatedResourceId()));
case "AssociatedResourceType":
return Optional.ofNullable(clazz.cast(associatedResourceTypeAsString()));
case "Created":
return Optional.ofNullable(clazz.cast(created()));
case "LastUpdatedTime":
return Optional.ofNullable(clazz.cast(lastUpdatedTime()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "StateReason":
return Optional.ofNullable(clazz.cast(stateReason()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function