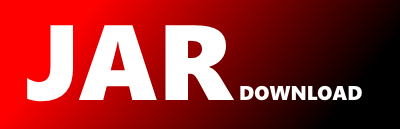
software.amazon.awssdk.services.workspacesthinclient.WorkSpacesThinClientAsyncClient Maven / Gradle / Ivy
Show all versions of workspacesthinclient Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workspacesthinclient;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.workspacesthinclient.model.CreateEnvironmentRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.CreateEnvironmentResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.DeleteDeviceRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.DeleteDeviceResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.DeleteEnvironmentRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.DeleteEnvironmentResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.DeregisterDeviceRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.DeregisterDeviceResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.GetDeviceRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.GetDeviceResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.GetEnvironmentRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.GetEnvironmentResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.GetSoftwareSetRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.GetSoftwareSetResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.TagResourceRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.TagResourceResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.UntagResourceRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.UntagResourceResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.UpdateDeviceRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.UpdateDeviceResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.UpdateEnvironmentRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.UpdateEnvironmentResponse;
import software.amazon.awssdk.services.workspacesthinclient.model.UpdateSoftwareSetRequest;
import software.amazon.awssdk.services.workspacesthinclient.model.UpdateSoftwareSetResponse;
import software.amazon.awssdk.services.workspacesthinclient.paginators.ListDevicesPublisher;
import software.amazon.awssdk.services.workspacesthinclient.paginators.ListEnvironmentsPublisher;
import software.amazon.awssdk.services.workspacesthinclient.paginators.ListSoftwareSetsPublisher;
/**
* Service client for accessing Amazon WorkSpaces Thin Client asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
*
* Amazon WorkSpaces Thin Client is an affordable device built to work with Amazon Web Services End User Computing (EUC)
* virtual desktops to provide users with a complete cloud desktop solution. WorkSpaces Thin Client is a compact device
* designed to connect up to two monitors and USB devices like a keyboard, mouse, headset, and webcam. To maximize
* endpoint security, WorkSpaces Thin Client devices do not allow local data storage or installation of unapproved
* applications. The WorkSpaces Thin Client device ships preloaded with device management software.
*
*
* You can use these APIs to complete WorkSpaces Thin Client tasks, such as creating environments or viewing devices.
* For more information about WorkSpaces Thin Client, including the required permissions to use the service, see the Amazon WorkSpaces Thin Client Administrator
* Guide. For more information about using the Command Line Interface (CLI) to manage your WorkSpaces Thin Client
* resources, see the WorkSpaces Thin Client
* section of the CLI Reference.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface WorkSpacesThinClientAsyncClient extends AwsClient {
String SERVICE_NAME = "thinclient";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "thinclient";
/**
*
* Creates an environment for your thin client devices.
*
*
* @param createEnvironmentRequest
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException Your request exceeds a service quota.
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.CreateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture createEnvironment(CreateEnvironmentRequest createEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an environment for your thin client devices.
*
*
*
* This is a convenience which creates an instance of the {@link CreateEnvironmentRequest.Builder} avoiding the need
* to create one manually via {@link CreateEnvironmentRequest#builder()}
*
*
* @param createEnvironmentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.CreateEnvironmentRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ServiceQuotaExceededException Your request exceeds a service quota.
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.CreateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture createEnvironment(
Consumer createEnvironmentRequest) {
return createEnvironment(CreateEnvironmentRequest.builder().applyMutation(createEnvironmentRequest).build());
}
/**
*
* Deletes a thin client device.
*
*
* @param deleteDeviceRequest
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.DeleteDevice
* @see AWS API Documentation
*/
default CompletableFuture deleteDevice(DeleteDeviceRequest deleteDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a thin client device.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDeviceRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDeviceRequest#builder()}
*
*
* @param deleteDeviceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.DeleteDeviceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.DeleteDevice
* @see AWS API Documentation
*/
default CompletableFuture deleteDevice(Consumer deleteDeviceRequest) {
return deleteDevice(DeleteDeviceRequest.builder().applyMutation(deleteDeviceRequest).build());
}
/**
*
* Deletes an environment.
*
*
* @param deleteEnvironmentRequest
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.DeleteEnvironment
* @see AWS API Documentation
*/
default CompletableFuture deleteEnvironment(DeleteEnvironmentRequest deleteEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an environment.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEnvironmentRequest.Builder} avoiding the need
* to create one manually via {@link DeleteEnvironmentRequest#builder()}
*
*
* @param deleteEnvironmentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.DeleteEnvironmentRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.DeleteEnvironment
* @see AWS API Documentation
*/
default CompletableFuture deleteEnvironment(
Consumer deleteEnvironmentRequest) {
return deleteEnvironment(DeleteEnvironmentRequest.builder().applyMutation(deleteEnvironmentRequest).build());
}
/**
*
* Deregisters a thin client device.
*
*
* @param deregisterDeviceRequest
* @return A Java Future containing the result of the DeregisterDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.DeregisterDevice
* @see AWS API Documentation
*/
default CompletableFuture deregisterDevice(DeregisterDeviceRequest deregisterDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters a thin client device.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterDeviceRequest.Builder} avoiding the need
* to create one manually via {@link DeregisterDeviceRequest#builder()}
*
*
* @param deregisterDeviceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.DeregisterDeviceRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeregisterDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.DeregisterDevice
* @see AWS API Documentation
*/
default CompletableFuture deregisterDevice(
Consumer deregisterDeviceRequest) {
return deregisterDevice(DeregisterDeviceRequest.builder().applyMutation(deregisterDeviceRequest).build());
}
/**
*
* Returns information for a thin client device.
*
*
* @param getDeviceRequest
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.GetDevice
* @see AWS API Documentation
*/
default CompletableFuture getDevice(GetDeviceRequest getDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information for a thin client device.
*
*
*
* This is a convenience which creates an instance of the {@link GetDeviceRequest.Builder} avoiding the need to
* create one manually via {@link GetDeviceRequest#builder()}
*
*
* @param getDeviceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.GetDeviceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.GetDevice
* @see AWS API Documentation
*/
default CompletableFuture getDevice(Consumer getDeviceRequest) {
return getDevice(GetDeviceRequest.builder().applyMutation(getDeviceRequest).build());
}
/**
*
* Returns information for an environment.
*
*
* @param getEnvironmentRequest
* @return A Java Future containing the result of the GetEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.GetEnvironment
* @see AWS API Documentation
*/
default CompletableFuture getEnvironment(GetEnvironmentRequest getEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information for an environment.
*
*
*
* This is a convenience which creates an instance of the {@link GetEnvironmentRequest.Builder} avoiding the need to
* create one manually via {@link GetEnvironmentRequest#builder()}
*
*
* @param getEnvironmentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.GetEnvironmentRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.GetEnvironment
* @see AWS API Documentation
*/
default CompletableFuture getEnvironment(Consumer getEnvironmentRequest) {
return getEnvironment(GetEnvironmentRequest.builder().applyMutation(getEnvironmentRequest).build());
}
/**
*
* Returns information for a software set.
*
*
* @param getSoftwareSetRequest
* @return A Java Future containing the result of the GetSoftwareSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.GetSoftwareSet
* @see AWS API Documentation
*/
default CompletableFuture getSoftwareSet(GetSoftwareSetRequest getSoftwareSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information for a software set.
*
*
*
* This is a convenience which creates an instance of the {@link GetSoftwareSetRequest.Builder} avoiding the need to
* create one manually via {@link GetSoftwareSetRequest#builder()}
*
*
* @param getSoftwareSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.GetSoftwareSetRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetSoftwareSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.GetSoftwareSet
* @see AWS API Documentation
*/
default CompletableFuture getSoftwareSet(Consumer getSoftwareSetRequest) {
return getSoftwareSet(GetSoftwareSetRequest.builder().applyMutation(getSoftwareSetRequest).build());
}
/**
*
* Returns a list of thin client devices.
*
*
* @param listDevicesRequest
* @return A Java Future containing the result of the ListDevices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListDevices
* @see AWS API Documentation
*/
default CompletableFuture listDevices(ListDevicesRequest listDevicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of thin client devices.
*
*
*
* This is a convenience which creates an instance of the {@link ListDevicesRequest.Builder} avoiding the need to
* create one manually via {@link ListDevicesRequest#builder()}
*
*
* @param listDevicesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListDevices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListDevices
* @see AWS API Documentation
*/
default CompletableFuture listDevices(Consumer listDevicesRequest) {
return listDevices(ListDevicesRequest.builder().applyMutation(listDevicesRequest).build());
}
/**
*
* This is a variant of
* {@link #listDevices(software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListDevicesPublisher publisher = client.listDevicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListDevicesPublisher publisher = client.listDevicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevices(software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesRequest)}
* operation.
*
*
* @param listDevicesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListDevices
* @see AWS API Documentation
*/
default ListDevicesPublisher listDevicesPaginator(ListDevicesRequest listDevicesRequest) {
return new ListDevicesPublisher(this, listDevicesRequest);
}
/**
*
* This is a variant of
* {@link #listDevices(software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListDevicesPublisher publisher = client.listDevicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListDevicesPublisher publisher = client.listDevicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevices(software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListDevicesRequest.Builder} avoiding the need to
* create one manually via {@link ListDevicesRequest#builder()}
*
*
* @param listDevicesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.ListDevicesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListDevices
* @see AWS API Documentation
*/
default ListDevicesPublisher listDevicesPaginator(Consumer listDevicesRequest) {
return listDevicesPaginator(ListDevicesRequest.builder().applyMutation(listDevicesRequest).build());
}
/**
*
* Returns a list of environments.
*
*
* @param listEnvironmentsRequest
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListEnvironments
* @see AWS API Documentation
*/
default CompletableFuture listEnvironments(ListEnvironmentsRequest listEnvironmentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of environments.
*
*
*
* This is a convenience which creates an instance of the {@link ListEnvironmentsRequest.Builder} avoiding the need
* to create one manually via {@link ListEnvironmentsRequest#builder()}
*
*
* @param listEnvironmentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListEnvironments
* @see AWS API Documentation
*/
default CompletableFuture listEnvironments(
Consumer listEnvironmentsRequest) {
return listEnvironments(ListEnvironmentsRequest.builder().applyMutation(listEnvironmentsRequest).build());
}
/**
*
* This is a variant of
* {@link #listEnvironments(software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListEnvironmentsPublisher publisher = client.listEnvironmentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListEnvironmentsPublisher publisher = client.listEnvironmentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEnvironments(software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsRequest)}
* operation.
*
*
* @param listEnvironmentsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListEnvironments
* @see AWS API Documentation
*/
default ListEnvironmentsPublisher listEnvironmentsPaginator(ListEnvironmentsRequest listEnvironmentsRequest) {
return new ListEnvironmentsPublisher(this, listEnvironmentsRequest);
}
/**
*
* This is a variant of
* {@link #listEnvironments(software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListEnvironmentsPublisher publisher = client.listEnvironmentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListEnvironmentsPublisher publisher = client.listEnvironmentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEnvironments(software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListEnvironmentsRequest.Builder} avoiding the need
* to create one manually via {@link ListEnvironmentsRequest#builder()}
*
*
* @param listEnvironmentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.ListEnvironmentsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListEnvironments
* @see AWS API Documentation
*/
default ListEnvironmentsPublisher listEnvironmentsPaginator(Consumer listEnvironmentsRequest) {
return listEnvironmentsPaginator(ListEnvironmentsRequest.builder().applyMutation(listEnvironmentsRequest).build());
}
/**
*
* Returns a list of software sets.
*
*
* @param listSoftwareSetsRequest
* @return A Java Future containing the result of the ListSoftwareSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListSoftwareSets
* @see AWS API Documentation
*/
default CompletableFuture listSoftwareSets(ListSoftwareSetsRequest listSoftwareSetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of software sets.
*
*
*
* This is a convenience which creates an instance of the {@link ListSoftwareSetsRequest.Builder} avoiding the need
* to create one manually via {@link ListSoftwareSetsRequest#builder()}
*
*
* @param listSoftwareSetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListSoftwareSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListSoftwareSets
* @see AWS API Documentation
*/
default CompletableFuture listSoftwareSets(
Consumer listSoftwareSetsRequest) {
return listSoftwareSets(ListSoftwareSetsRequest.builder().applyMutation(listSoftwareSetsRequest).build());
}
/**
*
* This is a variant of
* {@link #listSoftwareSets(software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListSoftwareSetsPublisher publisher = client.listSoftwareSetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListSoftwareSetsPublisher publisher = client.listSoftwareSetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSoftwareSets(software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsRequest)}
* operation.
*
*
* @param listSoftwareSetsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListSoftwareSets
* @see AWS API Documentation
*/
default ListSoftwareSetsPublisher listSoftwareSetsPaginator(ListSoftwareSetsRequest listSoftwareSetsRequest) {
return new ListSoftwareSetsPublisher(this, listSoftwareSetsRequest);
}
/**
*
* This is a variant of
* {@link #listSoftwareSets(software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListSoftwareSetsPublisher publisher = client.listSoftwareSetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesthinclient.paginators.ListSoftwareSetsPublisher publisher = client.listSoftwareSetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSoftwareSets(software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSoftwareSetsRequest.Builder} avoiding the need
* to create one manually via {@link ListSoftwareSetsRequest#builder()}
*
*
* @param listSoftwareSetsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.ListSoftwareSetsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListSoftwareSets
* @see AWS API Documentation
*/
default ListSoftwareSetsPublisher listSoftwareSetsPaginator(Consumer listSoftwareSetsRequest) {
return listSoftwareSetsPaginator(ListSoftwareSetsRequest.builder().applyMutation(listSoftwareSetsRequest).build());
}
/**
*
* Returns a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of tags for a resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.ListTagsForResourceRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Assigns one or more tags (key-value pairs) to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.TagResource
* @see AWS API Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Assigns one or more tags (key-value pairs) to the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.TagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.TagResource
* @see AWS API Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes a tag or tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.UntagResource
* @see AWS API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes a tag or tags from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.UntagResourceRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - ConflictException The requested operation would cause a conflict with the current state of a service
* resource associated with the request. Resolve the conflict before retrying this request.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.UntagResource
* @see AWS API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a thin client device.
*
*
* @param updateDeviceRequest
* @return A Java Future containing the result of the UpdateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.UpdateDevice
* @see AWS API Documentation
*/
default CompletableFuture updateDevice(UpdateDeviceRequest updateDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a thin client device.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDeviceRequest.Builder} avoiding the need to
* create one manually via {@link UpdateDeviceRequest#builder()}
*
*
* @param updateDeviceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.UpdateDeviceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.UpdateDevice
* @see AWS API Documentation
*/
default CompletableFuture updateDevice(Consumer updateDeviceRequest) {
return updateDevice(UpdateDeviceRequest.builder().applyMutation(updateDeviceRequest).build());
}
/**
*
* Updates an environment.
*
*
* @param updateEnvironmentRequest
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.UpdateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture updateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an environment.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEnvironmentRequest.Builder} avoiding the need
* to create one manually via {@link UpdateEnvironmentRequest#builder()}
*
*
* @param updateEnvironmentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.UpdateEnvironmentRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.UpdateEnvironment
* @see AWS API Documentation
*/
default CompletableFuture updateEnvironment(
Consumer updateEnvironmentRequest) {
return updateEnvironment(UpdateEnvironmentRequest.builder().applyMutation(updateEnvironmentRequest).build());
}
/**
*
* Updates a software set.
*
*
* @param updateSoftwareSetRequest
* @return A Java Future containing the result of the UpdateSoftwareSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.UpdateSoftwareSet
* @see AWS API Documentation
*/
default CompletableFuture updateSoftwareSet(UpdateSoftwareSetRequest updateSoftwareSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a software set.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSoftwareSetRequest.Builder} avoiding the need
* to create one manually via {@link UpdateSoftwareSetRequest#builder()}
*
*
* @param updateSoftwareSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesthinclient.model.UpdateSoftwareSetRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateSoftwareSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the specified constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The resource specified in the request was not found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The server encountered an internal error and is unable to complete the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesThinClientException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample WorkSpacesThinClientAsyncClient.UpdateSoftwareSet
* @see AWS API Documentation
*/
default CompletableFuture updateSoftwareSet(
Consumer updateSoftwareSetRequest) {
return updateSoftwareSet(UpdateSoftwareSetRequest.builder().applyMutation(updateSoftwareSetRequest).build());
}
@Override
default WorkSpacesThinClientServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link WorkSpacesThinClientAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static WorkSpacesThinClientAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link WorkSpacesThinClientAsyncClient}.
*/
static WorkSpacesThinClientAsyncClientBuilder builder() {
return new DefaultWorkSpacesThinClientAsyncClientBuilder();
}
}