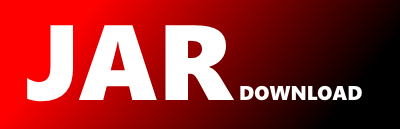
software.amazon.awssdk.services.workspacesweb.model.UserSettingsSummary Maven / Gradle / Ivy
Show all versions of workspacesweb Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workspacesweb.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The summary of user settings.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UserSettingsSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField COOKIE_SYNCHRONIZATION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("cookieSynchronizationConfiguration")
.getter(getter(UserSettingsSummary::cookieSynchronizationConfiguration))
.setter(setter(Builder::cookieSynchronizationConfiguration))
.constructor(CookieSynchronizationConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cookieSynchronizationConfiguration")
.build()).build();
private static final SdkField COPY_ALLOWED_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("copyAllowed").getter(getter(UserSettingsSummary::copyAllowedAsString))
.setter(setter(Builder::copyAllowed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("copyAllowed").build()).build();
private static final SdkField DISCONNECT_TIMEOUT_IN_MINUTES_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("disconnectTimeoutInMinutes")
.getter(getter(UserSettingsSummary::disconnectTimeoutInMinutes))
.setter(setter(Builder::disconnectTimeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("disconnectTimeoutInMinutes").build())
.build();
private static final SdkField DOWNLOAD_ALLOWED_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("downloadAllowed").getter(getter(UserSettingsSummary::downloadAllowedAsString))
.setter(setter(Builder::downloadAllowed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("downloadAllowed").build()).build();
private static final SdkField IDLE_DISCONNECT_TIMEOUT_IN_MINUTES_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("idleDisconnectTimeoutInMinutes")
.getter(getter(UserSettingsSummary::idleDisconnectTimeoutInMinutes))
.setter(setter(Builder::idleDisconnectTimeoutInMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("idleDisconnectTimeoutInMinutes")
.build()).build();
private static final SdkField PASTE_ALLOWED_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("pasteAllowed").getter(getter(UserSettingsSummary::pasteAllowedAsString))
.setter(setter(Builder::pasteAllowed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pasteAllowed").build()).build();
private static final SdkField PRINT_ALLOWED_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("printAllowed").getter(getter(UserSettingsSummary::printAllowedAsString))
.setter(setter(Builder::printAllowed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("printAllowed").build()).build();
private static final SdkField UPLOAD_ALLOWED_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("uploadAllowed").getter(getter(UserSettingsSummary::uploadAllowedAsString))
.setter(setter(Builder::uploadAllowed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("uploadAllowed").build()).build();
private static final SdkField USER_SETTINGS_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("userSettingsArn").getter(getter(UserSettingsSummary::userSettingsArn))
.setter(setter(Builder::userSettingsArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("userSettingsArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
COOKIE_SYNCHRONIZATION_CONFIGURATION_FIELD, COPY_ALLOWED_FIELD, DISCONNECT_TIMEOUT_IN_MINUTES_FIELD,
DOWNLOAD_ALLOWED_FIELD, IDLE_DISCONNECT_TIMEOUT_IN_MINUTES_FIELD, PASTE_ALLOWED_FIELD, PRINT_ALLOWED_FIELD,
UPLOAD_ALLOWED_FIELD, USER_SETTINGS_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final CookieSynchronizationConfiguration cookieSynchronizationConfiguration;
private final String copyAllowed;
private final Integer disconnectTimeoutInMinutes;
private final String downloadAllowed;
private final Integer idleDisconnectTimeoutInMinutes;
private final String pasteAllowed;
private final String printAllowed;
private final String uploadAllowed;
private final String userSettingsArn;
private UserSettingsSummary(BuilderImpl builder) {
this.cookieSynchronizationConfiguration = builder.cookieSynchronizationConfiguration;
this.copyAllowed = builder.copyAllowed;
this.disconnectTimeoutInMinutes = builder.disconnectTimeoutInMinutes;
this.downloadAllowed = builder.downloadAllowed;
this.idleDisconnectTimeoutInMinutes = builder.idleDisconnectTimeoutInMinutes;
this.pasteAllowed = builder.pasteAllowed;
this.printAllowed = builder.printAllowed;
this.uploadAllowed = builder.uploadAllowed;
this.userSettingsArn = builder.userSettingsArn;
}
/**
*
* The configuration that specifies which cookies should be synchronized from the end user's local browser to the
* remote browser.
*
*
* @return The configuration that specifies which cookies should be synchronized from the end user's local browser
* to the remote browser.
*/
public final CookieSynchronizationConfiguration cookieSynchronizationConfiguration() {
return cookieSynchronizationConfiguration;
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #copyAllowed} will
* return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #copyAllowedAsString}.
*
*
* @return Specifies whether the user can copy text from the streaming session to the local device.
* @see EnabledType
*/
public final EnabledType copyAllowed() {
return EnabledType.fromValue(copyAllowed);
}
/**
*
* Specifies whether the user can copy text from the streaming session to the local device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #copyAllowed} will
* return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #copyAllowedAsString}.
*
*
* @return Specifies whether the user can copy text from the streaming session to the local device.
* @see EnabledType
*/
public final String copyAllowedAsString() {
return copyAllowed;
}
/**
*
* The amount of time that a streaming session remains active after users disconnect.
*
*
* @return The amount of time that a streaming session remains active after users disconnect.
*/
public final Integer disconnectTimeoutInMinutes() {
return disconnectTimeoutInMinutes;
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #downloadAllowed}
* will return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #downloadAllowedAsString}.
*
*
* @return Specifies whether the user can download files from the streaming session to the local device.
* @see EnabledType
*/
public final EnabledType downloadAllowed() {
return EnabledType.fromValue(downloadAllowed);
}
/**
*
* Specifies whether the user can download files from the streaming session to the local device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #downloadAllowed}
* will return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #downloadAllowedAsString}.
*
*
* @return Specifies whether the user can download files from the streaming session to the local device.
* @see EnabledType
*/
public final String downloadAllowedAsString() {
return downloadAllowed;
}
/**
*
* The amount of time that users can be idle (inactive) before they are disconnected from their streaming session
* and the disconnect timeout interval begins.
*
*
* @return The amount of time that users can be idle (inactive) before they are disconnected from their streaming
* session and the disconnect timeout interval begins.
*/
public final Integer idleDisconnectTimeoutInMinutes() {
return idleDisconnectTimeoutInMinutes;
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pasteAllowed} will
* return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pasteAllowedAsString}.
*
*
* @return Specifies whether the user can paste text from the local device to the streaming session.
* @see EnabledType
*/
public final EnabledType pasteAllowed() {
return EnabledType.fromValue(pasteAllowed);
}
/**
*
* Specifies whether the user can paste text from the local device to the streaming session.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pasteAllowed} will
* return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pasteAllowedAsString}.
*
*
* @return Specifies whether the user can paste text from the local device to the streaming session.
* @see EnabledType
*/
public final String pasteAllowedAsString() {
return pasteAllowed;
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #printAllowed} will
* return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #printAllowedAsString}.
*
*
* @return Specifies whether the user can print to the local device.
* @see EnabledType
*/
public final EnabledType printAllowed() {
return EnabledType.fromValue(printAllowed);
}
/**
*
* Specifies whether the user can print to the local device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #printAllowed} will
* return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #printAllowedAsString}.
*
*
* @return Specifies whether the user can print to the local device.
* @see EnabledType
*/
public final String printAllowedAsString() {
return printAllowed;
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uploadAllowed}
* will return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uploadAllowedAsString}.
*
*
* @return Specifies whether the user can upload files from the local device to the streaming session.
* @see EnabledType
*/
public final EnabledType uploadAllowed() {
return EnabledType.fromValue(uploadAllowed);
}
/**
*
* Specifies whether the user can upload files from the local device to the streaming session.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uploadAllowed}
* will return {@link EnabledType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uploadAllowedAsString}.
*
*
* @return Specifies whether the user can upload files from the local device to the streaming session.
* @see EnabledType
*/
public final String uploadAllowedAsString() {
return uploadAllowed;
}
/**
*
* The ARN of the user settings.
*
*
* @return The ARN of the user settings.
*/
public final String userSettingsArn() {
return userSettingsArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(cookieSynchronizationConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(copyAllowedAsString());
hashCode = 31 * hashCode + Objects.hashCode(disconnectTimeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(downloadAllowedAsString());
hashCode = 31 * hashCode + Objects.hashCode(idleDisconnectTimeoutInMinutes());
hashCode = 31 * hashCode + Objects.hashCode(pasteAllowedAsString());
hashCode = 31 * hashCode + Objects.hashCode(printAllowedAsString());
hashCode = 31 * hashCode + Objects.hashCode(uploadAllowedAsString());
hashCode = 31 * hashCode + Objects.hashCode(userSettingsArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UserSettingsSummary)) {
return false;
}
UserSettingsSummary other = (UserSettingsSummary) obj;
return Objects.equals(cookieSynchronizationConfiguration(), other.cookieSynchronizationConfiguration())
&& Objects.equals(copyAllowedAsString(), other.copyAllowedAsString())
&& Objects.equals(disconnectTimeoutInMinutes(), other.disconnectTimeoutInMinutes())
&& Objects.equals(downloadAllowedAsString(), other.downloadAllowedAsString())
&& Objects.equals(idleDisconnectTimeoutInMinutes(), other.idleDisconnectTimeoutInMinutes())
&& Objects.equals(pasteAllowedAsString(), other.pasteAllowedAsString())
&& Objects.equals(printAllowedAsString(), other.printAllowedAsString())
&& Objects.equals(uploadAllowedAsString(), other.uploadAllowedAsString())
&& Objects.equals(userSettingsArn(), other.userSettingsArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("UserSettingsSummary")
.add("CookieSynchronizationConfiguration",
cookieSynchronizationConfiguration() == null ? null : "*** Sensitive Data Redacted ***")
.add("CopyAllowed", copyAllowedAsString()).add("DisconnectTimeoutInMinutes", disconnectTimeoutInMinutes())
.add("DownloadAllowed", downloadAllowedAsString())
.add("IdleDisconnectTimeoutInMinutes", idleDisconnectTimeoutInMinutes())
.add("PasteAllowed", pasteAllowedAsString()).add("PrintAllowed", printAllowedAsString())
.add("UploadAllowed", uploadAllowedAsString()).add("UserSettingsArn", userSettingsArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cookieSynchronizationConfiguration":
return Optional.ofNullable(clazz.cast(cookieSynchronizationConfiguration()));
case "copyAllowed":
return Optional.ofNullable(clazz.cast(copyAllowedAsString()));
case "disconnectTimeoutInMinutes":
return Optional.ofNullable(clazz.cast(disconnectTimeoutInMinutes()));
case "downloadAllowed":
return Optional.ofNullable(clazz.cast(downloadAllowedAsString()));
case "idleDisconnectTimeoutInMinutes":
return Optional.ofNullable(clazz.cast(idleDisconnectTimeoutInMinutes()));
case "pasteAllowed":
return Optional.ofNullable(clazz.cast(pasteAllowedAsString()));
case "printAllowed":
return Optional.ofNullable(clazz.cast(printAllowedAsString()));
case "uploadAllowed":
return Optional.ofNullable(clazz.cast(uploadAllowedAsString()));
case "userSettingsArn":
return Optional.ofNullable(clazz.cast(userSettingsArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function