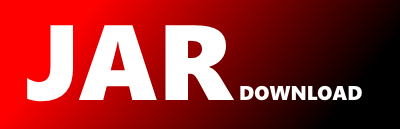
software.amazon.awssdk.services.workspacesweb.WorkSpacesWebAsyncClient Maven / Gradle / Ivy
Show all versions of workspacesweb Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.workspacesweb;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.workspacesweb.model.AssociateBrowserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.AssociateBrowserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.AssociateIpAccessSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.AssociateIpAccessSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.AssociateNetworkSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.AssociateNetworkSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.AssociateTrustStoreRequest;
import software.amazon.awssdk.services.workspacesweb.model.AssociateTrustStoreResponse;
import software.amazon.awssdk.services.workspacesweb.model.AssociateUserAccessLoggingSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.AssociateUserAccessLoggingSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.AssociateUserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.AssociateUserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.CreateBrowserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.CreateBrowserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.CreateIdentityProviderRequest;
import software.amazon.awssdk.services.workspacesweb.model.CreateIdentityProviderResponse;
import software.amazon.awssdk.services.workspacesweb.model.CreateIpAccessSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.CreateIpAccessSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.CreateNetworkSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.CreateNetworkSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.CreatePortalRequest;
import software.amazon.awssdk.services.workspacesweb.model.CreatePortalResponse;
import software.amazon.awssdk.services.workspacesweb.model.CreateTrustStoreRequest;
import software.amazon.awssdk.services.workspacesweb.model.CreateTrustStoreResponse;
import software.amazon.awssdk.services.workspacesweb.model.CreateUserAccessLoggingSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.CreateUserAccessLoggingSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.CreateUserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.CreateUserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DeleteBrowserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DeleteBrowserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DeleteIdentityProviderRequest;
import software.amazon.awssdk.services.workspacesweb.model.DeleteIdentityProviderResponse;
import software.amazon.awssdk.services.workspacesweb.model.DeleteIpAccessSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DeleteIpAccessSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DeleteNetworkSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DeleteNetworkSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DeletePortalRequest;
import software.amazon.awssdk.services.workspacesweb.model.DeletePortalResponse;
import software.amazon.awssdk.services.workspacesweb.model.DeleteTrustStoreRequest;
import software.amazon.awssdk.services.workspacesweb.model.DeleteTrustStoreResponse;
import software.amazon.awssdk.services.workspacesweb.model.DeleteUserAccessLoggingSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DeleteUserAccessLoggingSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DeleteUserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DeleteUserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateBrowserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateBrowserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateIpAccessSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateIpAccessSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateNetworkSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateNetworkSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateTrustStoreRequest;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateTrustStoreResponse;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateUserAccessLoggingSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateUserAccessLoggingSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateUserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.DisassociateUserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetBrowserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetBrowserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetIdentityProviderRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetIdentityProviderResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetIpAccessSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetIpAccessSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetNetworkSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetNetworkSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetPortalRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetPortalResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetPortalServiceProviderMetadataRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetPortalServiceProviderMetadataResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetTrustStoreCertificateRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetTrustStoreCertificateResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetTrustStoreRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetTrustStoreResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetUserAccessLoggingSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetUserAccessLoggingSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.GetUserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.GetUserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListPortalsRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListPortalsResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.TagResourceRequest;
import software.amazon.awssdk.services.workspacesweb.model.TagResourceResponse;
import software.amazon.awssdk.services.workspacesweb.model.UntagResourceRequest;
import software.amazon.awssdk.services.workspacesweb.model.UntagResourceResponse;
import software.amazon.awssdk.services.workspacesweb.model.UpdateBrowserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.UpdateBrowserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.UpdateIdentityProviderRequest;
import software.amazon.awssdk.services.workspacesweb.model.UpdateIdentityProviderResponse;
import software.amazon.awssdk.services.workspacesweb.model.UpdateIpAccessSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.UpdateIpAccessSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.UpdateNetworkSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.UpdateNetworkSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.UpdatePortalRequest;
import software.amazon.awssdk.services.workspacesweb.model.UpdatePortalResponse;
import software.amazon.awssdk.services.workspacesweb.model.UpdateTrustStoreRequest;
import software.amazon.awssdk.services.workspacesweb.model.UpdateTrustStoreResponse;
import software.amazon.awssdk.services.workspacesweb.model.UpdateUserAccessLoggingSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.UpdateUserAccessLoggingSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.model.UpdateUserSettingsRequest;
import software.amazon.awssdk.services.workspacesweb.model.UpdateUserSettingsResponse;
import software.amazon.awssdk.services.workspacesweb.paginators.ListBrowserSettingsPublisher;
import software.amazon.awssdk.services.workspacesweb.paginators.ListIdentityProvidersPublisher;
import software.amazon.awssdk.services.workspacesweb.paginators.ListIpAccessSettingsPublisher;
import software.amazon.awssdk.services.workspacesweb.paginators.ListNetworkSettingsPublisher;
import software.amazon.awssdk.services.workspacesweb.paginators.ListPortalsPublisher;
import software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoreCertificatesPublisher;
import software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoresPublisher;
import software.amazon.awssdk.services.workspacesweb.paginators.ListUserAccessLoggingSettingsPublisher;
import software.amazon.awssdk.services.workspacesweb.paginators.ListUserSettingsPublisher;
/**
* Service client for accessing Amazon WorkSpaces Web asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
*
* Amazon WorkSpaces Secure Browser is a low cost, fully managed WorkSpace built specifically to facilitate secure,
* web-based workloads. WorkSpaces Secure Browser makes it easy for customers to safely provide their employees with
* access to internal websites and SaaS web applications without the administrative burden of appliances or specialized
* client software. WorkSpaces Secure Browser provides simple policy tools tailored for user interactions, while
* offloading common tasks like capacity management, scaling, and maintaining browser images.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface WorkSpacesWebAsyncClient extends AwsClient {
String SERVICE_NAME = "workspaces-web";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "workspaces-web";
/**
*
* Associates a browser settings resource with a web portal.
*
*
* @param associateBrowserSettingsRequest
* @return A Java Future containing the result of the AssociateBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture associateBrowserSettings(
AssociateBrowserSettingsRequest associateBrowserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a browser settings resource with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateBrowserSettingsRequest.Builder} avoiding
* the need to create one manually via {@link AssociateBrowserSettingsRequest#builder()}
*
*
* @param associateBrowserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.AssociateBrowserSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture associateBrowserSettings(
Consumer associateBrowserSettingsRequest) {
return associateBrowserSettings(AssociateBrowserSettingsRequest.builder().applyMutation(associateBrowserSettingsRequest)
.build());
}
/**
*
* Associates an IP access settings resource with a web portal.
*
*
* @param associateIpAccessSettingsRequest
* @return A Java Future containing the result of the AssociateIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture associateIpAccessSettings(
AssociateIpAccessSettingsRequest associateIpAccessSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates an IP access settings resource with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateIpAccessSettingsRequest.Builder} avoiding
* the need to create one manually via {@link AssociateIpAccessSettingsRequest#builder()}
*
*
* @param associateIpAccessSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.AssociateIpAccessSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture associateIpAccessSettings(
Consumer associateIpAccessSettingsRequest) {
return associateIpAccessSettings(AssociateIpAccessSettingsRequest.builder()
.applyMutation(associateIpAccessSettingsRequest).build());
}
/**
*
* Associates a network settings resource with a web portal.
*
*
* @param associateNetworkSettingsRequest
* @return A Java Future containing the result of the AssociateNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture associateNetworkSettings(
AssociateNetworkSettingsRequest associateNetworkSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a network settings resource with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateNetworkSettingsRequest.Builder} avoiding
* the need to create one manually via {@link AssociateNetworkSettingsRequest#builder()}
*
*
* @param associateNetworkSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.AssociateNetworkSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture associateNetworkSettings(
Consumer associateNetworkSettingsRequest) {
return associateNetworkSettings(AssociateNetworkSettingsRequest.builder().applyMutation(associateNetworkSettingsRequest)
.build());
}
/**
*
* Associates a trust store with a web portal.
*
*
* @param associateTrustStoreRequest
* @return A Java Future containing the result of the AssociateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture associateTrustStore(
AssociateTrustStoreRequest associateTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a trust store with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateTrustStoreRequest.Builder} avoiding the
* need to create one manually via {@link AssociateTrustStoreRequest#builder()}
*
*
* @param associateTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.AssociateTrustStoreRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture associateTrustStore(
Consumer associateTrustStoreRequest) {
return associateTrustStore(AssociateTrustStoreRequest.builder().applyMutation(associateTrustStoreRequest).build());
}
/**
*
* Associates a user access logging settings resource with a web portal.
*
*
* @param associateUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the AssociateUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture associateUserAccessLoggingSettings(
AssociateUserAccessLoggingSettingsRequest associateUserAccessLoggingSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a user access logging settings resource with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateUserAccessLoggingSettingsRequest.Builder}
* avoiding the need to create one manually via {@link AssociateUserAccessLoggingSettingsRequest#builder()}
*
*
* @param associateUserAccessLoggingSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.AssociateUserAccessLoggingSettingsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the AssociateUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture associateUserAccessLoggingSettings(
Consumer associateUserAccessLoggingSettingsRequest) {
return associateUserAccessLoggingSettings(AssociateUserAccessLoggingSettingsRequest.builder()
.applyMutation(associateUserAccessLoggingSettingsRequest).build());
}
/**
*
* Associates a user settings resource with a web portal.
*
*
* @param associateUserSettingsRequest
* @return A Java Future containing the result of the AssociateUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateUserSettings
* @see AWS API Documentation
*/
default CompletableFuture associateUserSettings(
AssociateUserSettingsRequest associateUserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a user settings resource with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateUserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link AssociateUserSettingsRequest#builder()}
*
*
* @param associateUserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.AssociateUserSettingsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the AssociateUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.AssociateUserSettings
* @see AWS API Documentation
*/
default CompletableFuture associateUserSettings(
Consumer associateUserSettingsRequest) {
return associateUserSettings(AssociateUserSettingsRequest.builder().applyMutation(associateUserSettingsRequest).build());
}
/**
*
* Creates a browser settings resource that can be associated with a web portal. Once associated with a web portal,
* browser settings control how the browser will behave once a user starts a streaming session for the web portal.
*
*
* @param createBrowserSettingsRequest
* @return A Java Future containing the result of the CreateBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture createBrowserSettings(
CreateBrowserSettingsRequest createBrowserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a browser settings resource that can be associated with a web portal. Once associated with a web portal,
* browser settings control how the browser will behave once a user starts a streaming session for the web portal.
*
*
*
* This is a convenience which creates an instance of the {@link CreateBrowserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link CreateBrowserSettingsRequest#builder()}
*
*
* @param createBrowserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.CreateBrowserSettingsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture createBrowserSettings(
Consumer createBrowserSettingsRequest) {
return createBrowserSettings(CreateBrowserSettingsRequest.builder().applyMutation(createBrowserSettingsRequest).build());
}
/**
*
* Creates an identity provider resource that is then associated with a web portal.
*
*
* @param createIdentityProviderRequest
* @return A Java Future containing the result of the CreateIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateIdentityProvider
* @see AWS API Documentation
*/
default CompletableFuture createIdentityProvider(
CreateIdentityProviderRequest createIdentityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an identity provider resource that is then associated with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link CreateIdentityProviderRequest.Builder} avoiding the
* need to create one manually via {@link CreateIdentityProviderRequest#builder()}
*
*
* @param createIdentityProviderRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.CreateIdentityProviderRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateIdentityProvider
* @see AWS API Documentation
*/
default CompletableFuture createIdentityProvider(
Consumer createIdentityProviderRequest) {
return createIdentityProvider(CreateIdentityProviderRequest.builder().applyMutation(createIdentityProviderRequest)
.build());
}
/**
*
* Creates an IP access settings resource that can be associated with a web portal.
*
*
* @param createIpAccessSettingsRequest
* @return A Java Future containing the result of the CreateIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture createIpAccessSettings(
CreateIpAccessSettingsRequest createIpAccessSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an IP access settings resource that can be associated with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link CreateIpAccessSettingsRequest.Builder} avoiding the
* need to create one manually via {@link CreateIpAccessSettingsRequest#builder()}
*
*
* @param createIpAccessSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.CreateIpAccessSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture createIpAccessSettings(
Consumer createIpAccessSettingsRequest) {
return createIpAccessSettings(CreateIpAccessSettingsRequest.builder().applyMutation(createIpAccessSettingsRequest)
.build());
}
/**
*
* Creates a network settings resource that can be associated with a web portal. Once associated with a web portal,
* network settings define how streaming instances will connect with your specified VPC.
*
*
* @param createNetworkSettingsRequest
* @return A Java Future containing the result of the CreateNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture createNetworkSettings(
CreateNetworkSettingsRequest createNetworkSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a network settings resource that can be associated with a web portal. Once associated with a web portal,
* network settings define how streaming instances will connect with your specified VPC.
*
*
*
* This is a convenience which creates an instance of the {@link CreateNetworkSettingsRequest.Builder} avoiding the
* need to create one manually via {@link CreateNetworkSettingsRequest#builder()}
*
*
* @param createNetworkSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.CreateNetworkSettingsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture createNetworkSettings(
Consumer createNetworkSettingsRequest) {
return createNetworkSettings(CreateNetworkSettingsRequest.builder().applyMutation(createNetworkSettingsRequest).build());
}
/**
*
* Creates a web portal.
*
*
* @param createPortalRequest
* @return A Java Future containing the result of the CreatePortal operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreatePortal
* @see AWS
* API Documentation
*/
default CompletableFuture createPortal(CreatePortalRequest createPortalRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePortalRequest.Builder} avoiding the need to
* create one manually via {@link CreatePortalRequest#builder()}
*
*
* @param createPortalRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.CreatePortalRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreatePortal operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreatePortal
* @see AWS
* API Documentation
*/
default CompletableFuture createPortal(Consumer createPortalRequest) {
return createPortal(CreatePortalRequest.builder().applyMutation(createPortalRequest).build());
}
/**
*
* Creates a trust store that can be associated with a web portal. A trust store contains certificate authority (CA)
* certificates. Once associated with a web portal, the browser in a streaming session will recognize certificates
* that have been issued using any of the CAs in the trust store. If your organization has internal websites that
* use certificates issued by private CAs, you should add the private CA certificate to the trust store.
*
*
* @param createTrustStoreRequest
* @return A Java Future containing the result of the CreateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture createTrustStore(CreateTrustStoreRequest createTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a trust store that can be associated with a web portal. A trust store contains certificate authority (CA)
* certificates. Once associated with a web portal, the browser in a streaming session will recognize certificates
* that have been issued using any of the CAs in the trust store. If your organization has internal websites that
* use certificates issued by private CAs, you should add the private CA certificate to the trust store.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTrustStoreRequest.Builder} avoiding the need
* to create one manually via {@link CreateTrustStoreRequest#builder()}
*
*
* @param createTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.CreateTrustStoreRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture createTrustStore(
Consumer createTrustStoreRequest) {
return createTrustStore(CreateTrustStoreRequest.builder().applyMutation(createTrustStoreRequest).build());
}
/**
*
* Creates a user access logging settings resource that can be associated with a web portal.
*
*
* @param createUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the CreateUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture createUserAccessLoggingSettings(
CreateUserAccessLoggingSettingsRequest createUserAccessLoggingSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a user access logging settings resource that can be associated with a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserAccessLoggingSettingsRequest.Builder}
* avoiding the need to create one manually via {@link CreateUserAccessLoggingSettingsRequest#builder()}
*
*
* @param createUserAccessLoggingSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.CreateUserAccessLoggingSettingsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the CreateUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture createUserAccessLoggingSettings(
Consumer createUserAccessLoggingSettingsRequest) {
return createUserAccessLoggingSettings(CreateUserAccessLoggingSettingsRequest.builder()
.applyMutation(createUserAccessLoggingSettingsRequest).build());
}
/**
*
* Creates a user settings resource that can be associated with a web portal. Once associated with a web portal,
* user settings control how users can transfer data between a streaming session and the their local devices.
*
*
* @param createUserSettingsRequest
* @return A Java Future containing the result of the CreateUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateUserSettings
* @see AWS API Documentation
*/
default CompletableFuture createUserSettings(CreateUserSettingsRequest createUserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a user settings resource that can be associated with a web portal. Once associated with a web portal,
* user settings control how users can transfer data between a streaming session and the their local devices.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link CreateUserSettingsRequest#builder()}
*
*
* @param createUserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.CreateUserSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.CreateUserSettings
* @see AWS API Documentation
*/
default CompletableFuture createUserSettings(
Consumer createUserSettingsRequest) {
return createUserSettings(CreateUserSettingsRequest.builder().applyMutation(createUserSettingsRequest).build());
}
/**
*
* Deletes browser settings.
*
*
* @param deleteBrowserSettingsRequest
* @return A Java Future containing the result of the DeleteBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteBrowserSettings(
DeleteBrowserSettingsRequest deleteBrowserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes browser settings.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteBrowserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link DeleteBrowserSettingsRequest#builder()}
*
*
* @param deleteBrowserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DeleteBrowserSettingsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeleteBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteBrowserSettings(
Consumer deleteBrowserSettingsRequest) {
return deleteBrowserSettings(DeleteBrowserSettingsRequest.builder().applyMutation(deleteBrowserSettingsRequest).build());
}
/**
*
* Deletes the identity provider.
*
*
* @param deleteIdentityProviderRequest
* @return A Java Future containing the result of the DeleteIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteIdentityProvider
* @see AWS API Documentation
*/
default CompletableFuture deleteIdentityProvider(
DeleteIdentityProviderRequest deleteIdentityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the identity provider.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteIdentityProviderRequest.Builder} avoiding the
* need to create one manually via {@link DeleteIdentityProviderRequest#builder()}
*
*
* @param deleteIdentityProviderRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DeleteIdentityProviderRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteIdentityProvider
* @see AWS API Documentation
*/
default CompletableFuture deleteIdentityProvider(
Consumer deleteIdentityProviderRequest) {
return deleteIdentityProvider(DeleteIdentityProviderRequest.builder().applyMutation(deleteIdentityProviderRequest)
.build());
}
/**
*
* Deletes IP access settings.
*
*
* @param deleteIpAccessSettingsRequest
* @return A Java Future containing the result of the DeleteIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteIpAccessSettings(
DeleteIpAccessSettingsRequest deleteIpAccessSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes IP access settings.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteIpAccessSettingsRequest.Builder} avoiding the
* need to create one manually via {@link DeleteIpAccessSettingsRequest#builder()}
*
*
* @param deleteIpAccessSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DeleteIpAccessSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteIpAccessSettings(
Consumer deleteIpAccessSettingsRequest) {
return deleteIpAccessSettings(DeleteIpAccessSettingsRequest.builder().applyMutation(deleteIpAccessSettingsRequest)
.build());
}
/**
*
* Deletes network settings.
*
*
* @param deleteNetworkSettingsRequest
* @return A Java Future containing the result of the DeleteNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteNetworkSettings(
DeleteNetworkSettingsRequest deleteNetworkSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes network settings.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNetworkSettingsRequest.Builder} avoiding the
* need to create one manually via {@link DeleteNetworkSettingsRequest#builder()}
*
*
* @param deleteNetworkSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DeleteNetworkSettingsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeleteNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteNetworkSettings(
Consumer deleteNetworkSettingsRequest) {
return deleteNetworkSettings(DeleteNetworkSettingsRequest.builder().applyMutation(deleteNetworkSettingsRequest).build());
}
/**
*
* Deletes a web portal.
*
*
* @param deletePortalRequest
* @return A Java Future containing the result of the DeletePortal operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeletePortal
* @see AWS
* API Documentation
*/
default CompletableFuture deletePortal(DeletePortalRequest deletePortalRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePortalRequest.Builder} avoiding the need to
* create one manually via {@link DeletePortalRequest#builder()}
*
*
* @param deletePortalRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DeletePortalRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeletePortal operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeletePortal
* @see AWS
* API Documentation
*/
default CompletableFuture deletePortal(Consumer deletePortalRequest) {
return deletePortal(DeletePortalRequest.builder().applyMutation(deletePortalRequest).build());
}
/**
*
* Deletes the trust store.
*
*
* @param deleteTrustStoreRequest
* @return A Java Future containing the result of the DeleteTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteTrustStore
* @see AWS API Documentation
*/
default CompletableFuture deleteTrustStore(DeleteTrustStoreRequest deleteTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the trust store.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTrustStoreRequest.Builder} avoiding the need
* to create one manually via {@link DeleteTrustStoreRequest#builder()}
*
*
* @param deleteTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DeleteTrustStoreRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteTrustStore
* @see AWS API Documentation
*/
default CompletableFuture deleteTrustStore(
Consumer deleteTrustStoreRequest) {
return deleteTrustStore(DeleteTrustStoreRequest.builder().applyMutation(deleteTrustStoreRequest).build());
}
/**
*
* Deletes user access logging settings.
*
*
* @param deleteUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the DeleteUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteUserAccessLoggingSettings(
DeleteUserAccessLoggingSettingsRequest deleteUserAccessLoggingSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes user access logging settings.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserAccessLoggingSettingsRequest.Builder}
* avoiding the need to create one manually via {@link DeleteUserAccessLoggingSettingsRequest#builder()}
*
*
* @param deleteUserAccessLoggingSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DeleteUserAccessLoggingSettingsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DeleteUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteUserAccessLoggingSettings(
Consumer deleteUserAccessLoggingSettingsRequest) {
return deleteUserAccessLoggingSettings(DeleteUserAccessLoggingSettingsRequest.builder()
.applyMutation(deleteUserAccessLoggingSettingsRequest).build());
}
/**
*
* Deletes user settings.
*
*
* @param deleteUserSettingsRequest
* @return A Java Future containing the result of the DeleteUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteUserSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteUserSettings(DeleteUserSettingsRequest deleteUserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes user settings.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link DeleteUserSettingsRequest#builder()}
*
*
* @param deleteUserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DeleteUserSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DeleteUserSettings
* @see AWS API Documentation
*/
default CompletableFuture deleteUserSettings(
Consumer deleteUserSettingsRequest) {
return deleteUserSettings(DeleteUserSettingsRequest.builder().applyMutation(deleteUserSettingsRequest).build());
}
/**
*
* Disassociates browser settings from a web portal.
*
*
* @param disassociateBrowserSettingsRequest
* @return A Java Future containing the result of the DisassociateBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateBrowserSettings(
DisassociateBrowserSettingsRequest disassociateBrowserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates browser settings from a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateBrowserSettingsRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateBrowserSettingsRequest#builder()}
*
*
* @param disassociateBrowserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DisassociateBrowserSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateBrowserSettings(
Consumer disassociateBrowserSettingsRequest) {
return disassociateBrowserSettings(DisassociateBrowserSettingsRequest.builder()
.applyMutation(disassociateBrowserSettingsRequest).build());
}
/**
*
* Disassociates IP access settings from a web portal.
*
*
* @param disassociateIpAccessSettingsRequest
* @return A Java Future containing the result of the DisassociateIpAccessSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateIpAccessSettings(
DisassociateIpAccessSettingsRequest disassociateIpAccessSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates IP access settings from a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateIpAccessSettingsRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateIpAccessSettingsRequest#builder()}
*
*
* @param disassociateIpAccessSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DisassociateIpAccessSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateIpAccessSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateIpAccessSettings(
Consumer disassociateIpAccessSettingsRequest) {
return disassociateIpAccessSettings(DisassociateIpAccessSettingsRequest.builder()
.applyMutation(disassociateIpAccessSettingsRequest).build());
}
/**
*
* Disassociates network settings from a web portal.
*
*
* @param disassociateNetworkSettingsRequest
* @return A Java Future containing the result of the DisassociateNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateNetworkSettings(
DisassociateNetworkSettingsRequest disassociateNetworkSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates network settings from a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateNetworkSettingsRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateNetworkSettingsRequest#builder()}
*
*
* @param disassociateNetworkSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DisassociateNetworkSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateNetworkSettings(
Consumer disassociateNetworkSettingsRequest) {
return disassociateNetworkSettings(DisassociateNetworkSettingsRequest.builder()
.applyMutation(disassociateNetworkSettingsRequest).build());
}
/**
*
* Disassociates a trust store from a web portal.
*
*
* @param disassociateTrustStoreRequest
* @return A Java Future containing the result of the DisassociateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture disassociateTrustStore(
DisassociateTrustStoreRequest disassociateTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a trust store from a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateTrustStoreRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateTrustStoreRequest#builder()}
*
*
* @param disassociateTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DisassociateTrustStoreRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture disassociateTrustStore(
Consumer disassociateTrustStoreRequest) {
return disassociateTrustStore(DisassociateTrustStoreRequest.builder().applyMutation(disassociateTrustStoreRequest)
.build());
}
/**
*
* Disassociates user access logging settings from a web portal.
*
*
* @param disassociateUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the DisassociateUserAccessLoggingSettings operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateUserAccessLoggingSettings(
DisassociateUserAccessLoggingSettingsRequest disassociateUserAccessLoggingSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates user access logging settings from a web portal.
*
*
*
* This is a convenience which creates an instance of the
* {@link DisassociateUserAccessLoggingSettingsRequest.Builder} avoiding the need to create one manually via
* {@link DisassociateUserAccessLoggingSettingsRequest#builder()}
*
*
* @param disassociateUserAccessLoggingSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DisassociateUserAccessLoggingSettingsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DisassociateUserAccessLoggingSettings operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateUserAccessLoggingSettings(
Consumer disassociateUserAccessLoggingSettingsRequest) {
return disassociateUserAccessLoggingSettings(DisassociateUserAccessLoggingSettingsRequest.builder()
.applyMutation(disassociateUserAccessLoggingSettingsRequest).build());
}
/**
*
* Disassociates user settings from a web portal.
*
*
* @param disassociateUserSettingsRequest
* @return A Java Future containing the result of the DisassociateUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateUserSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateUserSettings(
DisassociateUserSettingsRequest disassociateUserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates user settings from a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateUserSettingsRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateUserSettingsRequest#builder()}
*
*
* @param disassociateUserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.DisassociateUserSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.DisassociateUserSettings
* @see AWS API Documentation
*/
default CompletableFuture disassociateUserSettings(
Consumer disassociateUserSettingsRequest) {
return disassociateUserSettings(DisassociateUserSettingsRequest.builder().applyMutation(disassociateUserSettingsRequest)
.build());
}
/**
*
* Gets browser settings.
*
*
* @param getBrowserSettingsRequest
* @return A Java Future containing the result of the GetBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture getBrowserSettings(GetBrowserSettingsRequest getBrowserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets browser settings.
*
*
*
* This is a convenience which creates an instance of the {@link GetBrowserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link GetBrowserSettingsRequest#builder()}
*
*
* @param getBrowserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetBrowserSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture getBrowserSettings(
Consumer getBrowserSettingsRequest) {
return getBrowserSettings(GetBrowserSettingsRequest.builder().applyMutation(getBrowserSettingsRequest).build());
}
/**
*
* Gets the identity provider.
*
*
* @param getIdentityProviderRequest
* @return A Java Future containing the result of the GetIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetIdentityProvider
* @see AWS API Documentation
*/
default CompletableFuture getIdentityProvider(
GetIdentityProviderRequest getIdentityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the identity provider.
*
*
*
* This is a convenience which creates an instance of the {@link GetIdentityProviderRequest.Builder} avoiding the
* need to create one manually via {@link GetIdentityProviderRequest#builder()}
*
*
* @param getIdentityProviderRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetIdentityProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetIdentityProvider
* @see AWS API Documentation
*/
default CompletableFuture getIdentityProvider(
Consumer getIdentityProviderRequest) {
return getIdentityProvider(GetIdentityProviderRequest.builder().applyMutation(getIdentityProviderRequest).build());
}
/**
*
* Gets the IP access settings.
*
*
* @param getIpAccessSettingsRequest
* @return A Java Future containing the result of the GetIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture getIpAccessSettings(
GetIpAccessSettingsRequest getIpAccessSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the IP access settings.
*
*
*
* This is a convenience which creates an instance of the {@link GetIpAccessSettingsRequest.Builder} avoiding the
* need to create one manually via {@link GetIpAccessSettingsRequest#builder()}
*
*
* @param getIpAccessSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetIpAccessSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture getIpAccessSettings(
Consumer getIpAccessSettingsRequest) {
return getIpAccessSettings(GetIpAccessSettingsRequest.builder().applyMutation(getIpAccessSettingsRequest).build());
}
/**
*
* Gets the network settings.
*
*
* @param getNetworkSettingsRequest
* @return A Java Future containing the result of the GetNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture getNetworkSettings(GetNetworkSettingsRequest getNetworkSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the network settings.
*
*
*
* This is a convenience which creates an instance of the {@link GetNetworkSettingsRequest.Builder} avoiding the
* need to create one manually via {@link GetNetworkSettingsRequest#builder()}
*
*
* @param getNetworkSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetNetworkSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture getNetworkSettings(
Consumer getNetworkSettingsRequest) {
return getNetworkSettings(GetNetworkSettingsRequest.builder().applyMutation(getNetworkSettingsRequest).build());
}
/**
*
* Gets the web portal.
*
*
* @param getPortalRequest
* @return A Java Future containing the result of the GetPortal operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetPortal
* @see AWS API
* Documentation
*/
default CompletableFuture getPortal(GetPortalRequest getPortalRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the web portal.
*
*
*
* This is a convenience which creates an instance of the {@link GetPortalRequest.Builder} avoiding the need to
* create one manually via {@link GetPortalRequest#builder()}
*
*
* @param getPortalRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetPortalRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetPortal operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetPortal
* @see AWS API
* Documentation
*/
default CompletableFuture getPortal(Consumer getPortalRequest) {
return getPortal(GetPortalRequest.builder().applyMutation(getPortalRequest).build());
}
/**
*
* Gets the service provider metadata.
*
*
* @param getPortalServiceProviderMetadataRequest
* @return A Java Future containing the result of the GetPortalServiceProviderMetadata operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetPortalServiceProviderMetadata
* @see AWS API Documentation
*/
default CompletableFuture getPortalServiceProviderMetadata(
GetPortalServiceProviderMetadataRequest getPortalServiceProviderMetadataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the service provider metadata.
*
*
*
* This is a convenience which creates an instance of the {@link GetPortalServiceProviderMetadataRequest.Builder}
* avoiding the need to create one manually via {@link GetPortalServiceProviderMetadataRequest#builder()}
*
*
* @param getPortalServiceProviderMetadataRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetPortalServiceProviderMetadataRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetPortalServiceProviderMetadata operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetPortalServiceProviderMetadata
* @see AWS API Documentation
*/
default CompletableFuture getPortalServiceProviderMetadata(
Consumer getPortalServiceProviderMetadataRequest) {
return getPortalServiceProviderMetadata(GetPortalServiceProviderMetadataRequest.builder()
.applyMutation(getPortalServiceProviderMetadataRequest).build());
}
/**
*
* Gets the trust store.
*
*
* @param getTrustStoreRequest
* @return A Java Future containing the result of the GetTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetTrustStore
* @see AWS
* API Documentation
*/
default CompletableFuture getTrustStore(GetTrustStoreRequest getTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the trust store.
*
*
*
* This is a convenience which creates an instance of the {@link GetTrustStoreRequest.Builder} avoiding the need to
* create one manually via {@link GetTrustStoreRequest#builder()}
*
*
* @param getTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetTrustStoreRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetTrustStore
* @see AWS
* API Documentation
*/
default CompletableFuture getTrustStore(Consumer getTrustStoreRequest) {
return getTrustStore(GetTrustStoreRequest.builder().applyMutation(getTrustStoreRequest).build());
}
/**
*
* Gets the trust store certificate.
*
*
* @param getTrustStoreCertificateRequest
* @return A Java Future containing the result of the GetTrustStoreCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetTrustStoreCertificate
* @see AWS API Documentation
*/
default CompletableFuture getTrustStoreCertificate(
GetTrustStoreCertificateRequest getTrustStoreCertificateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the trust store certificate.
*
*
*
* This is a convenience which creates an instance of the {@link GetTrustStoreCertificateRequest.Builder} avoiding
* the need to create one manually via {@link GetTrustStoreCertificateRequest#builder()}
*
*
* @param getTrustStoreCertificateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetTrustStoreCertificateRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetTrustStoreCertificate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetTrustStoreCertificate
* @see AWS API Documentation
*/
default CompletableFuture getTrustStoreCertificate(
Consumer getTrustStoreCertificateRequest) {
return getTrustStoreCertificate(GetTrustStoreCertificateRequest.builder().applyMutation(getTrustStoreCertificateRequest)
.build());
}
/**
*
* Gets user access logging settings.
*
*
* @param getUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the GetUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture getUserAccessLoggingSettings(
GetUserAccessLoggingSettingsRequest getUserAccessLoggingSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets user access logging settings.
*
*
*
* This is a convenience which creates an instance of the {@link GetUserAccessLoggingSettingsRequest.Builder}
* avoiding the need to create one manually via {@link GetUserAccessLoggingSettingsRequest#builder()}
*
*
* @param getUserAccessLoggingSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetUserAccessLoggingSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture getUserAccessLoggingSettings(
Consumer getUserAccessLoggingSettingsRequest) {
return getUserAccessLoggingSettings(GetUserAccessLoggingSettingsRequest.builder()
.applyMutation(getUserAccessLoggingSettingsRequest).build());
}
/**
*
* Gets user settings.
*
*
* @param getUserSettingsRequest
* @return A Java Future containing the result of the GetUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetUserSettings
* @see AWS API Documentation
*/
default CompletableFuture getUserSettings(GetUserSettingsRequest getUserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets user settings.
*
*
*
* This is a convenience which creates an instance of the {@link GetUserSettingsRequest.Builder} avoiding the need
* to create one manually via {@link GetUserSettingsRequest#builder()}
*
*
* @param getUserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.GetUserSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.GetUserSettings
* @see AWS API Documentation
*/
default CompletableFuture getUserSettings(
Consumer getUserSettingsRequest) {
return getUserSettings(GetUserSettingsRequest.builder().applyMutation(getUserSettingsRequest).build());
}
/**
*
* Retrieves a list of browser settings.
*
*
* @param listBrowserSettingsRequest
* @return A Java Future containing the result of the ListBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture listBrowserSettings(
ListBrowserSettingsRequest listBrowserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of browser settings.
*
*
*
* This is a convenience which creates an instance of the {@link ListBrowserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link ListBrowserSettingsRequest#builder()}
*
*
* @param listBrowserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture listBrowserSettings(
Consumer listBrowserSettingsRequest) {
return listBrowserSettings(ListBrowserSettingsRequest.builder().applyMutation(listBrowserSettingsRequest).build());
}
/**
*
* This is a variant of
* {@link #listBrowserSettings(software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListBrowserSettingsPublisher publisher = client.listBrowserSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListBrowserSettingsPublisher publisher = client.listBrowserSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBrowserSettings(software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsRequest)}
* operation.
*
*
* @param listBrowserSettingsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListBrowserSettings
* @see AWS API Documentation
*/
default ListBrowserSettingsPublisher listBrowserSettingsPaginator(ListBrowserSettingsRequest listBrowserSettingsRequest) {
return new ListBrowserSettingsPublisher(this, listBrowserSettingsRequest);
}
/**
*
* This is a variant of
* {@link #listBrowserSettings(software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListBrowserSettingsPublisher publisher = client.listBrowserSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListBrowserSettingsPublisher publisher = client.listBrowserSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBrowserSettings(software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListBrowserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link ListBrowserSettingsRequest#builder()}
*
*
* @param listBrowserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListBrowserSettingsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListBrowserSettings
* @see AWS API Documentation
*/
default ListBrowserSettingsPublisher listBrowserSettingsPaginator(
Consumer listBrowserSettingsRequest) {
return listBrowserSettingsPaginator(ListBrowserSettingsRequest.builder().applyMutation(listBrowserSettingsRequest)
.build());
}
/**
*
* Retrieves a list of identity providers for a specific web portal.
*
*
* @param listIdentityProvidersRequest
* @return A Java Future containing the result of the ListIdentityProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListIdentityProviders
* @see AWS API Documentation
*/
default CompletableFuture listIdentityProviders(
ListIdentityProvidersRequest listIdentityProvidersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of identity providers for a specific web portal.
*
*
*
* This is a convenience which creates an instance of the {@link ListIdentityProvidersRequest.Builder} avoiding the
* need to create one manually via {@link ListIdentityProvidersRequest#builder()}
*
*
* @param listIdentityProvidersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListIdentityProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListIdentityProviders
* @see AWS API Documentation
*/
default CompletableFuture listIdentityProviders(
Consumer listIdentityProvidersRequest) {
return listIdentityProviders(ListIdentityProvidersRequest.builder().applyMutation(listIdentityProvidersRequest).build());
}
/**
*
* This is a variant of
* {@link #listIdentityProviders(software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListIdentityProvidersPublisher publisher = client.listIdentityProvidersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListIdentityProvidersPublisher publisher = client.listIdentityProvidersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIdentityProviders(software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersRequest)}
* operation.
*
*
* @param listIdentityProvidersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListIdentityProviders
* @see AWS API Documentation
*/
default ListIdentityProvidersPublisher listIdentityProvidersPaginator(
ListIdentityProvidersRequest listIdentityProvidersRequest) {
return new ListIdentityProvidersPublisher(this, listIdentityProvidersRequest);
}
/**
*
* This is a variant of
* {@link #listIdentityProviders(software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListIdentityProvidersPublisher publisher = client.listIdentityProvidersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListIdentityProvidersPublisher publisher = client.listIdentityProvidersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIdentityProviders(software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListIdentityProvidersRequest.Builder} avoiding the
* need to create one manually via {@link ListIdentityProvidersRequest#builder()}
*
*
* @param listIdentityProvidersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListIdentityProvidersRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListIdentityProviders
* @see AWS API Documentation
*/
default ListIdentityProvidersPublisher listIdentityProvidersPaginator(
Consumer listIdentityProvidersRequest) {
return listIdentityProvidersPaginator(ListIdentityProvidersRequest.builder().applyMutation(listIdentityProvidersRequest)
.build());
}
/**
*
* Retrieves a list of IP access settings.
*
*
* @param listIpAccessSettingsRequest
* @return A Java Future containing the result of the ListIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture listIpAccessSettings(
ListIpAccessSettingsRequest listIpAccessSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of IP access settings.
*
*
*
* This is a convenience which creates an instance of the {@link ListIpAccessSettingsRequest.Builder} avoiding the
* need to create one manually via {@link ListIpAccessSettingsRequest#builder()}
*
*
* @param listIpAccessSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture listIpAccessSettings(
Consumer listIpAccessSettingsRequest) {
return listIpAccessSettings(ListIpAccessSettingsRequest.builder().applyMutation(listIpAccessSettingsRequest).build());
}
/**
*
* This is a variant of
* {@link #listIpAccessSettings(software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListIpAccessSettingsPublisher publisher = client.listIpAccessSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListIpAccessSettingsPublisher publisher = client.listIpAccessSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIpAccessSettings(software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsRequest)}
* operation.
*
*
* @param listIpAccessSettingsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListIpAccessSettings
* @see AWS API Documentation
*/
default ListIpAccessSettingsPublisher listIpAccessSettingsPaginator(ListIpAccessSettingsRequest listIpAccessSettingsRequest) {
return new ListIpAccessSettingsPublisher(this, listIpAccessSettingsRequest);
}
/**
*
* This is a variant of
* {@link #listIpAccessSettings(software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListIpAccessSettingsPublisher publisher = client.listIpAccessSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListIpAccessSettingsPublisher publisher = client.listIpAccessSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIpAccessSettings(software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListIpAccessSettingsRequest.Builder} avoiding the
* need to create one manually via {@link ListIpAccessSettingsRequest#builder()}
*
*
* @param listIpAccessSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListIpAccessSettingsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListIpAccessSettings
* @see AWS API Documentation
*/
default ListIpAccessSettingsPublisher listIpAccessSettingsPaginator(
Consumer listIpAccessSettingsRequest) {
return listIpAccessSettingsPaginator(ListIpAccessSettingsRequest.builder().applyMutation(listIpAccessSettingsRequest)
.build());
}
/**
*
* Retrieves a list of network settings.
*
*
* @param listNetworkSettingsRequest
* @return A Java Future containing the result of the ListNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture listNetworkSettings(
ListNetworkSettingsRequest listNetworkSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of network settings.
*
*
*
* This is a convenience which creates an instance of the {@link ListNetworkSettingsRequest.Builder} avoiding the
* need to create one manually via {@link ListNetworkSettingsRequest#builder()}
*
*
* @param listNetworkSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture listNetworkSettings(
Consumer listNetworkSettingsRequest) {
return listNetworkSettings(ListNetworkSettingsRequest.builder().applyMutation(listNetworkSettingsRequest).build());
}
/**
*
* This is a variant of
* {@link #listNetworkSettings(software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListNetworkSettingsPublisher publisher = client.listNetworkSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListNetworkSettingsPublisher publisher = client.listNetworkSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listNetworkSettings(software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsRequest)}
* operation.
*
*
* @param listNetworkSettingsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListNetworkSettings
* @see AWS API Documentation
*/
default ListNetworkSettingsPublisher listNetworkSettingsPaginator(ListNetworkSettingsRequest listNetworkSettingsRequest) {
return new ListNetworkSettingsPublisher(this, listNetworkSettingsRequest);
}
/**
*
* This is a variant of
* {@link #listNetworkSettings(software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListNetworkSettingsPublisher publisher = client.listNetworkSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListNetworkSettingsPublisher publisher = client.listNetworkSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listNetworkSettings(software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListNetworkSettingsRequest.Builder} avoiding the
* need to create one manually via {@link ListNetworkSettingsRequest#builder()}
*
*
* @param listNetworkSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListNetworkSettingsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListNetworkSettings
* @see AWS API Documentation
*/
default ListNetworkSettingsPublisher listNetworkSettingsPaginator(
Consumer listNetworkSettingsRequest) {
return listNetworkSettingsPaginator(ListNetworkSettingsRequest.builder().applyMutation(listNetworkSettingsRequest)
.build());
}
/**
*
* Retrieves a list or web portals.
*
*
* @param listPortalsRequest
* @return A Java Future containing the result of the ListPortals operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListPortals
* @see AWS
* API Documentation
*/
default CompletableFuture listPortals(ListPortalsRequest listPortalsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list or web portals.
*
*
*
* This is a convenience which creates an instance of the {@link ListPortalsRequest.Builder} avoiding the need to
* create one manually via {@link ListPortalsRequest#builder()}
*
*
* @param listPortalsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListPortalsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPortals operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListPortals
* @see AWS
* API Documentation
*/
default CompletableFuture listPortals(Consumer listPortalsRequest) {
return listPortals(ListPortalsRequest.builder().applyMutation(listPortalsRequest).build());
}
/**
*
* This is a variant of {@link #listPortals(software.amazon.awssdk.services.workspacesweb.model.ListPortalsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListPortalsPublisher publisher = client.listPortalsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListPortalsPublisher publisher = client.listPortalsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListPortalsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPortals(software.amazon.awssdk.services.workspacesweb.model.ListPortalsRequest)} operation.
*
*
* @param listPortalsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListPortals
* @see AWS
* API Documentation
*/
default ListPortalsPublisher listPortalsPaginator(ListPortalsRequest listPortalsRequest) {
return new ListPortalsPublisher(this, listPortalsRequest);
}
/**
*
* This is a variant of {@link #listPortals(software.amazon.awssdk.services.workspacesweb.model.ListPortalsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListPortalsPublisher publisher = client.listPortalsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListPortalsPublisher publisher = client.listPortalsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListPortalsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPortals(software.amazon.awssdk.services.workspacesweb.model.ListPortalsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListPortalsRequest.Builder} avoiding the need to
* create one manually via {@link ListPortalsRequest#builder()}
*
*
* @param listPortalsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListPortalsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListPortals
* @see AWS
* API Documentation
*/
default ListPortalsPublisher listPortalsPaginator(Consumer listPortalsRequest) {
return listPortalsPaginator(ListPortalsRequest.builder().applyMutation(listPortalsRequest).build());
}
/**
*
* Retrieves a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of tags for a resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Retrieves a list of trust store certificates.
*
*
* @param listTrustStoreCertificatesRequest
* @return A Java Future containing the result of the ListTrustStoreCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTrustStoreCertificates
* @see AWS API Documentation
*/
default CompletableFuture listTrustStoreCertificates(
ListTrustStoreCertificatesRequest listTrustStoreCertificatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of trust store certificates.
*
*
*
* This is a convenience which creates an instance of the {@link ListTrustStoreCertificatesRequest.Builder} avoiding
* the need to create one manually via {@link ListTrustStoreCertificatesRequest#builder()}
*
*
* @param listTrustStoreCertificatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListTrustStoreCertificates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTrustStoreCertificates
* @see AWS API Documentation
*/
default CompletableFuture listTrustStoreCertificates(
Consumer listTrustStoreCertificatesRequest) {
return listTrustStoreCertificates(ListTrustStoreCertificatesRequest.builder()
.applyMutation(listTrustStoreCertificatesRequest).build());
}
/**
*
* This is a variant of
* {@link #listTrustStoreCertificates(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoreCertificatesPublisher publisher = client.listTrustStoreCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoreCertificatesPublisher publisher = client.listTrustStoreCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTrustStoreCertificates(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesRequest)}
* operation.
*
*
* @param listTrustStoreCertificatesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTrustStoreCertificates
* @see AWS API Documentation
*/
default ListTrustStoreCertificatesPublisher listTrustStoreCertificatesPaginator(
ListTrustStoreCertificatesRequest listTrustStoreCertificatesRequest) {
return new ListTrustStoreCertificatesPublisher(this, listTrustStoreCertificatesRequest);
}
/**
*
* This is a variant of
* {@link #listTrustStoreCertificates(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoreCertificatesPublisher publisher = client.listTrustStoreCertificatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoreCertificatesPublisher publisher = client.listTrustStoreCertificatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTrustStoreCertificates(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTrustStoreCertificatesRequest.Builder} avoiding
* the need to create one manually via {@link ListTrustStoreCertificatesRequest#builder()}
*
*
* @param listTrustStoreCertificatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListTrustStoreCertificatesRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTrustStoreCertificates
* @see AWS API Documentation
*/
default ListTrustStoreCertificatesPublisher listTrustStoreCertificatesPaginator(
Consumer listTrustStoreCertificatesRequest) {
return listTrustStoreCertificatesPaginator(ListTrustStoreCertificatesRequest.builder()
.applyMutation(listTrustStoreCertificatesRequest).build());
}
/**
*
* Retrieves a list of trust stores.
*
*
* @param listTrustStoresRequest
* @return A Java Future containing the result of the ListTrustStores operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTrustStores
* @see AWS API Documentation
*/
default CompletableFuture listTrustStores(ListTrustStoresRequest listTrustStoresRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of trust stores.
*
*
*
* This is a convenience which creates an instance of the {@link ListTrustStoresRequest.Builder} avoiding the need
* to create one manually via {@link ListTrustStoresRequest#builder()}
*
*
* @param listTrustStoresRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTrustStores operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTrustStores
* @see AWS API Documentation
*/
default CompletableFuture listTrustStores(
Consumer listTrustStoresRequest) {
return listTrustStores(ListTrustStoresRequest.builder().applyMutation(listTrustStoresRequest).build());
}
/**
*
* This is a variant of
* {@link #listTrustStores(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoresPublisher publisher = client.listTrustStoresPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoresPublisher publisher = client.listTrustStoresPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTrustStores(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresRequest)}
* operation.
*
*
* @param listTrustStoresRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTrustStores
* @see AWS API Documentation
*/
default ListTrustStoresPublisher listTrustStoresPaginator(ListTrustStoresRequest listTrustStoresRequest) {
return new ListTrustStoresPublisher(this, listTrustStoresRequest);
}
/**
*
* This is a variant of
* {@link #listTrustStores(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoresPublisher publisher = client.listTrustStoresPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListTrustStoresPublisher publisher = client.listTrustStoresPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTrustStores(software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListTrustStoresRequest.Builder} avoiding the need
* to create one manually via {@link ListTrustStoresRequest#builder()}
*
*
* @param listTrustStoresRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListTrustStoresRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListTrustStores
* @see AWS API Documentation
*/
default ListTrustStoresPublisher listTrustStoresPaginator(Consumer listTrustStoresRequest) {
return listTrustStoresPaginator(ListTrustStoresRequest.builder().applyMutation(listTrustStoresRequest).build());
}
/**
*
* Retrieves a list of user access logging settings.
*
*
* @param listUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the ListUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture listUserAccessLoggingSettings(
ListUserAccessLoggingSettingsRequest listUserAccessLoggingSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of user access logging settings.
*
*
*
* This is a convenience which creates an instance of the {@link ListUserAccessLoggingSettingsRequest.Builder}
* avoiding the need to create one manually via {@link ListUserAccessLoggingSettingsRequest#builder()}
*
*
* @param listUserAccessLoggingSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ListUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture listUserAccessLoggingSettings(
Consumer listUserAccessLoggingSettingsRequest) {
return listUserAccessLoggingSettings(ListUserAccessLoggingSettingsRequest.builder()
.applyMutation(listUserAccessLoggingSettingsRequest).build());
}
/**
*
* This is a variant of
* {@link #listUserAccessLoggingSettings(software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListUserAccessLoggingSettingsPublisher publisher = client.listUserAccessLoggingSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListUserAccessLoggingSettingsPublisher publisher = client.listUserAccessLoggingSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUserAccessLoggingSettings(software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsRequest)}
* operation.
*
*
* @param listUserAccessLoggingSettingsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListUserAccessLoggingSettings
* @see AWS API Documentation
*/
default ListUserAccessLoggingSettingsPublisher listUserAccessLoggingSettingsPaginator(
ListUserAccessLoggingSettingsRequest listUserAccessLoggingSettingsRequest) {
return new ListUserAccessLoggingSettingsPublisher(this, listUserAccessLoggingSettingsRequest);
}
/**
*
* This is a variant of
* {@link #listUserAccessLoggingSettings(software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListUserAccessLoggingSettingsPublisher publisher = client.listUserAccessLoggingSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListUserAccessLoggingSettingsPublisher publisher = client.listUserAccessLoggingSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUserAccessLoggingSettings(software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListUserAccessLoggingSettingsRequest.Builder}
* avoiding the need to create one manually via {@link ListUserAccessLoggingSettingsRequest#builder()}
*
*
* @param listUserAccessLoggingSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListUserAccessLoggingSettingsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListUserAccessLoggingSettings
* @see AWS API Documentation
*/
default ListUserAccessLoggingSettingsPublisher listUserAccessLoggingSettingsPaginator(
Consumer listUserAccessLoggingSettingsRequest) {
return listUserAccessLoggingSettingsPaginator(ListUserAccessLoggingSettingsRequest.builder()
.applyMutation(listUserAccessLoggingSettingsRequest).build());
}
/**
*
* Retrieves a list of user settings.
*
*
* @param listUserSettingsRequest
* @return A Java Future containing the result of the ListUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListUserSettings
* @see AWS API Documentation
*/
default CompletableFuture listUserSettings(ListUserSettingsRequest listUserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of user settings.
*
*
*
* This is a convenience which creates an instance of the {@link ListUserSettingsRequest.Builder} avoiding the need
* to create one manually via {@link ListUserSettingsRequest#builder()}
*
*
* @param listUserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListUserSettings
* @see AWS API Documentation
*/
default CompletableFuture listUserSettings(
Consumer listUserSettingsRequest) {
return listUserSettings(ListUserSettingsRequest.builder().applyMutation(listUserSettingsRequest).build());
}
/**
*
* This is a variant of
* {@link #listUserSettings(software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListUserSettingsPublisher publisher = client.listUserSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListUserSettingsPublisher publisher = client.listUserSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUserSettings(software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsRequest)}
* operation.
*
*
* @param listUserSettingsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListUserSettings
* @see AWS API Documentation
*/
default ListUserSettingsPublisher listUserSettingsPaginator(ListUserSettingsRequest listUserSettingsRequest) {
return new ListUserSettingsPublisher(this, listUserSettingsRequest);
}
/**
*
* This is a variant of
* {@link #listUserSettings(software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListUserSettingsPublisher publisher = client.listUserSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.workspacesweb.paginators.ListUserSettingsPublisher publisher = client.listUserSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUserSettings(software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListUserSettingsRequest.Builder} avoiding the need
* to create one manually via {@link ListUserSettingsRequest#builder()}
*
*
* @param listUserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.ListUserSettingsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.ListUserSettings
* @see AWS API Documentation
*/
default ListUserSettingsPublisher listUserSettingsPaginator(Consumer listUserSettingsRequest) {
return listUserSettingsPaginator(ListUserSettingsRequest.builder().applyMutation(listUserSettingsRequest).build());
}
/**
*
* Adds or overwrites one or more tags for the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - TooManyTagsException There are too many tags.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or overwrites one or more tags for the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.TagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - TooManyTagsException There are too many tags.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes one or more tags from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UntagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates browser settings.
*
*
* @param updateBrowserSettingsRequest
* @return A Java Future containing the result of the UpdateBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture updateBrowserSettings(
UpdateBrowserSettingsRequest updateBrowserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates browser settings.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateBrowserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link UpdateBrowserSettingsRequest#builder()}
*
*
* @param updateBrowserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UpdateBrowserSettingsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the UpdateBrowserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateBrowserSettings
* @see AWS API Documentation
*/
default CompletableFuture updateBrowserSettings(
Consumer updateBrowserSettingsRequest) {
return updateBrowserSettings(UpdateBrowserSettingsRequest.builder().applyMutation(updateBrowserSettingsRequest).build());
}
/**
*
* Updates the identity provider.
*
*
* @param updateIdentityProviderRequest
* @return A Java Future containing the result of the UpdateIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateIdentityProvider
* @see AWS API Documentation
*/
default CompletableFuture updateIdentityProvider(
UpdateIdentityProviderRequest updateIdentityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the identity provider.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateIdentityProviderRequest.Builder} avoiding the
* need to create one manually via {@link UpdateIdentityProviderRequest#builder()}
*
*
* @param updateIdentityProviderRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UpdateIdentityProviderRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateIdentityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateIdentityProvider
* @see AWS API Documentation
*/
default CompletableFuture updateIdentityProvider(
Consumer updateIdentityProviderRequest) {
return updateIdentityProvider(UpdateIdentityProviderRequest.builder().applyMutation(updateIdentityProviderRequest)
.build());
}
/**
*
* Updates IP access settings.
*
*
* @param updateIpAccessSettingsRequest
* @return A Java Future containing the result of the UpdateIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture updateIpAccessSettings(
UpdateIpAccessSettingsRequest updateIpAccessSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates IP access settings.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateIpAccessSettingsRequest.Builder} avoiding the
* need to create one manually via {@link UpdateIpAccessSettingsRequest#builder()}
*
*
* @param updateIpAccessSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UpdateIpAccessSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateIpAccessSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateIpAccessSettings
* @see AWS API Documentation
*/
default CompletableFuture updateIpAccessSettings(
Consumer updateIpAccessSettingsRequest) {
return updateIpAccessSettings(UpdateIpAccessSettingsRequest.builder().applyMutation(updateIpAccessSettingsRequest)
.build());
}
/**
*
* Updates network settings.
*
*
* @param updateNetworkSettingsRequest
* @return A Java Future containing the result of the UpdateNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture updateNetworkSettings(
UpdateNetworkSettingsRequest updateNetworkSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates network settings.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateNetworkSettingsRequest.Builder} avoiding the
* need to create one manually via {@link UpdateNetworkSettingsRequest#builder()}
*
*
* @param updateNetworkSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UpdateNetworkSettingsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the UpdateNetworkSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateNetworkSettings
* @see AWS API Documentation
*/
default CompletableFuture updateNetworkSettings(
Consumer updateNetworkSettingsRequest) {
return updateNetworkSettings(UpdateNetworkSettingsRequest.builder().applyMutation(updateNetworkSettingsRequest).build());
}
/**
*
* Updates a web portal.
*
*
* @param updatePortalRequest
* @return A Java Future containing the result of the UpdatePortal operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdatePortal
* @see AWS
* API Documentation
*/
default CompletableFuture updatePortal(UpdatePortalRequest updatePortalRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a web portal.
*
*
*
* This is a convenience which creates an instance of the {@link UpdatePortalRequest.Builder} avoiding the need to
* create one manually via {@link UpdatePortalRequest#builder()}
*
*
* @param updatePortalRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UpdatePortalRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdatePortal operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - ConflictException There is a conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdatePortal
* @see AWS
* API Documentation
*/
default CompletableFuture updatePortal(Consumer updatePortalRequest) {
return updatePortal(UpdatePortalRequest.builder().applyMutation(updatePortalRequest).build());
}
/**
*
* Updates the trust store.
*
*
* @param updateTrustStoreRequest
* @return A Java Future containing the result of the UpdateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture updateTrustStore(UpdateTrustStoreRequest updateTrustStoreRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the trust store.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateTrustStoreRequest.Builder} avoiding the need
* to create one manually via {@link UpdateTrustStoreRequest#builder()}
*
*
* @param updateTrustStoreRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UpdateTrustStoreRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateTrustStore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateTrustStore
* @see AWS API Documentation
*/
default CompletableFuture updateTrustStore(
Consumer updateTrustStoreRequest) {
return updateTrustStore(UpdateTrustStoreRequest.builder().applyMutation(updateTrustStoreRequest).build());
}
/**
*
* Updates the user access logging settings.
*
*
* @param updateUserAccessLoggingSettingsRequest
* @return A Java Future containing the result of the UpdateUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture updateUserAccessLoggingSettings(
UpdateUserAccessLoggingSettingsRequest updateUserAccessLoggingSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the user access logging settings.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserAccessLoggingSettingsRequest.Builder}
* avoiding the need to create one manually via {@link UpdateUserAccessLoggingSettingsRequest#builder()}
*
*
* @param updateUserAccessLoggingSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UpdateUserAccessLoggingSettingsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the UpdateUserAccessLoggingSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateUserAccessLoggingSettings
* @see AWS API Documentation
*/
default CompletableFuture updateUserAccessLoggingSettings(
Consumer updateUserAccessLoggingSettingsRequest) {
return updateUserAccessLoggingSettings(UpdateUserAccessLoggingSettingsRequest.builder()
.applyMutation(updateUserAccessLoggingSettingsRequest).build());
}
/**
*
* Updates the user settings.
*
*
* @param updateUserSettingsRequest
* @return A Java Future containing the result of the UpdateUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateUserSettings
* @see AWS API Documentation
*/
default CompletableFuture updateUserSettings(UpdateUserSettingsRequest updateUserSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the user settings.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserSettingsRequest.Builder} avoiding the
* need to create one manually via {@link UpdateUserSettingsRequest#builder()}
*
*
* @param updateUserSettingsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.workspacesweb.model.UpdateUserSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateUserSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException There is an internal server error.
* - ResourceNotFoundException The resource cannot be found.
* - AccessDeniedException Access is denied.
* - ThrottlingException There is a throttling error.
* - ValidationException There is a validation error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - WorkSpacesWebException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample WorkSpacesWebAsyncClient.UpdateUserSettings
* @see AWS API Documentation
*/
default CompletableFuture updateUserSettings(
Consumer updateUserSettingsRequest) {
return updateUserSettings(UpdateUserSettingsRequest.builder().applyMutation(updateUserSettingsRequest).build());
}
@Override
default WorkSpacesWebServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link WorkSpacesWebAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static WorkSpacesWebAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link WorkSpacesWebAsyncClient}.
*/
static WorkSpacesWebAsyncClientBuilder builder() {
return new DefaultWorkSpacesWebAsyncClientBuilder();
}
}