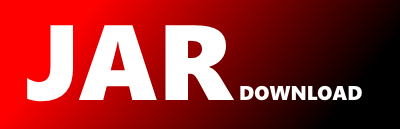
software.amazon.smithy.build.ProjectionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smithy-build Show documentation
Show all versions of smithy-build Show documentation
This module is a library used to validate Smithy models, create filtered projections of a model, and generate build artifacts.
/*
* Copyright 2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package software.amazon.smithy.build;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import software.amazon.smithy.model.Model;
import software.amazon.smithy.model.validation.Severity;
import software.amazon.smithy.model.validation.ValidationEvent;
import software.amazon.smithy.utils.BuilderRef;
import software.amazon.smithy.utils.SmithyBuilder;
/**
* The result of applying a projection to a model.
*/
public final class ProjectionResult {
private final String projectionName;
private final Model model;
private final Map pluginManifests;
private final List events;
private ProjectionResult(Builder builder) {
this.projectionName = SmithyBuilder.requiredState("projectionName", builder.projectionName);
this.model = SmithyBuilder.requiredState("model", builder.model);
this.events = builder.events.copy();
this.pluginManifests = builder.pluginManifests.copy();
}
/**
* Creates a {@link ProjectionResult} builder.
*
* @return Returns the created builder.
*/
public static Builder builder() {
return new Builder();
}
/**
* Gets the name of the projection that was executed.
*
* @return Returns the projection name.
*/
public String getProjectionName() {
return projectionName;
}
/**
* Gets the result of projecting the model.
*
* @return Returns the projected model.
*/
public Model getModel() {
return model;
}
/**
* Returns true if the projection contains error or danger events.
*
* @return Returns true if the projected model is broken.
*/
public boolean isBroken() {
for (ValidationEvent e : events) {
if (e.getSeverity() == Severity.ERROR || e.getSeverity() == Severity.DANGER) {
return true;
}
}
return false;
}
/**
* Gets the validation events encountered after projecting the model.
*
* @return Returns the encountered validation events.
*/
public List getEvents() {
return events;
}
/**
* Gets the results of each plugin.
*
* @return Returns a map of plugin names to their file manifests.
*/
public Map getPluginManifests() {
return pluginManifests;
}
/**
* Gets the result of a specific plugin by plugin artifact name.
*
* If no artifact name is configured for a plugin in smithy-build.json (e.g., "plugin::artifact"), the
* artifact name defaults to the plugin name.
*
* @param artifactName Name of the plugin artifact to retrieve.
* @return Returns files created by the given plugin or an empty list.
*/
public Optional getPluginManifest(String artifactName) {
return Optional.ofNullable(pluginManifests.get(artifactName));
}
/**
* Builds up a {@link ProjectionResult}.
*/
public static final class Builder implements SmithyBuilder {
private String projectionName;
private Model model;
private final BuilderRef
© 2015 - 2025 Weber Informatics LLC | Privacy Policy