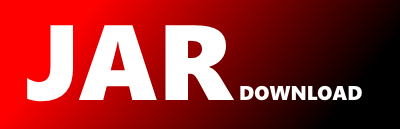
software.amazon.smithy.diff.testrunner.SmithyDiffTestSuite Maven / Gradle / Ivy
Show all versions of smithy-diff Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
* SPDX-License-Identifier: Apache-2.0
*/
package software.amazon.smithy.diff.testrunner;
import java.io.IOException;
import java.net.URISyntaxException;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.Future;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.smithy.diff.ModelDiff;
import software.amazon.smithy.model.Model;
import software.amazon.smithy.model.loader.ModelAssembler;
/**
* Runs diff test cases against corresponding model `a`, model `b`, and validation `events` files.
*/
public final class SmithyDiffTestSuite {
static final String EVENTS = ".events";
private static final String DEFAULT_TEST_CASE_LOCATION = "diffs";
private static final String EXT_SMITHY = ".smithy";
private static final String EXT_JSON = ".json";
private static final String MODEL_A = ".a";
private static final String MODEL_B = ".b";
private final List cases = new ArrayList();
private Supplier modelAssemblerFactory = ModelAssembler::new;
private SmithyDiffTestSuite() {}
/**
* Creates a new Smithy diff test suite.
*
* @return Returns the created test suite.
*/
public static SmithyDiffTestSuite runner() {
return new SmithyDiffTestSuite();
}
/**
* Factory method used to easily create a JUnit 5 {@code ParameterizedTest}
* {@code MethodSource} based on the given {@code Class}.
*
* This method assumes that there is a resource named {@code diffs}
* relative to the given class that contains test cases. It also assumes
* validators and traits should be loaded using the {@code ClassLoader}
* of the given {@code contextClass}, and that model discovery should be
* used using the given {@code contextClass}.
*
*
Each returned {@code Object[]} contains the filename of the test as
* the first argument, followed by a {@code Callable}
* as the second argument. All a parameterized test needs to do is call
* {@code call} on the provided {@code Callable} to execute the test and
* fail if the test case is invalid.
*
* For example, the following can be used as a unit test:
*
*
{@code
* import java.util.concurrent.Callable;
* import java.util.stream.Stream;
* import org.junit.jupiter.params.ParameterizedTest;
* import org.junit.jupiter.params.provider.MethodSource;
* import software.amazon.smithy.diff.testrunner.SmithyDiffTestCase;
* import software.amazon.smithy.diff.testrunner.SmithyDiffTestSuite;
*
* public class TestRunnerTest {
* \@ParameterizedTest(name = "\{0\}")
* \@MethodSource("source")
* public void testRunner(String filename, Callable<SmithyDiffTestCase.Result> callable)
* throws Exception {
* callable.call();
* }
*
* public static Stream<?> source() {
* return SmithyDiffTestSuite.defaultParameterizedTestSource(TestRunnerTest.class);
* }
* }
* }
*
* @param contextClass The class to use for loading diffs and model discovery.
* @return Returns the Stream that should be used as a JUnit 5 {@code MethodSource} return value.
*/
public static Stream