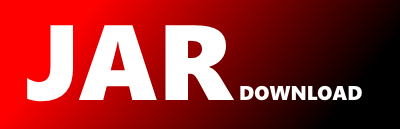
software.crldev.elrondspringbootstarterreactive.api.ApiResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api;
import software.crldev.elrondspringbootstarterreactive.error.exception.ResponseException;
import static io.netty.util.internal.StringUtil.isNullOrEmpty;
/**
* Base API response received from the Elrond proxy
* with generic based on response type
*
* @author carlo_stanciu
*/
public class ApiResponse {
private String error;
private String code;
private T data;
/**
* Method throws exception if the
* api response code is other than successful
*/
public void throwIfError() {
if (!isNullOrEmpty(error)) throw new ResponseException(error);
if (!code.equals("successful")) throw new ResponseException();
}
public ApiResponse() {
}
public String getError() {
return this.error;
}
public String getCode() {
return this.code;
}
public T getData() {
return this.data;
}
public void setError(final String error) {
this.error = error;
}
public void setCode(final String code) {
this.code = code;
}
public void setData(final T data) {
this.data = data;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ApiResponse)) return false;
final ApiResponse> other = (ApiResponse>) o;
if (!other.canEqual((Object) this)) return false;
final Object this$error = this.getError();
final Object other$error = other.getError();
if (this$error == null ? other$error != null : !this$error.equals(other$error)) return false;
final Object this$code = this.getCode();
final Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final Object this$data = this.getData();
final Object other$data = other.getData();
if (this$data == null ? other$data != null : !this$data.equals(other$data)) return false;
return true;
}
protected boolean canEqual(final Object other) {
return other instanceof ApiResponse;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $error = this.getError();
result = result * PRIME + ($error == null ? 43 : $error.hashCode());
final Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final Object $data = this.getData();
result = result * PRIME + ($data == null ? 43 : $data.hashCode());
return result;
}
@Override
public String toString() {
return "ApiResponse(error=" + this.getError() + ", code=" + this.getCode() + ", data=" + this.getData() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy