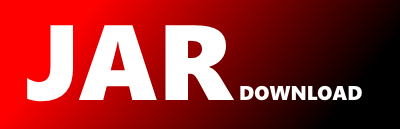
software.crldev.elrondspringbootstarterreactive.api.model.AccountESDTRoles Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Map;
import java.util.Set;
/**
* part of API response used in ESDT role queries
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = AccountESDTRoles.AccountESDTRolesBuilder.class)
public final class AccountESDTRoles {
@JsonProperty("roles")
private final Map> roles;
AccountESDTRoles(final Map> roles) {
this.roles = roles;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class AccountESDTRolesBuilder {
private Map> roles;
AccountESDTRolesBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("roles")
public AccountESDTRoles.AccountESDTRolesBuilder roles(final Map> roles) {
this.roles = roles;
return this;
}
public AccountESDTRoles build() {
return new AccountESDTRoles(this.roles);
}
@Override
public String toString() {
return "AccountESDTRoles.AccountESDTRolesBuilder(roles=" + this.roles + ")";
}
}
public static AccountESDTRoles.AccountESDTRolesBuilder builder() {
return new AccountESDTRoles.AccountESDTRolesBuilder();
}
public Map> getRoles() {
return this.roles;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof AccountESDTRoles)) return false;
final AccountESDTRoles other = (AccountESDTRoles) o;
final Object this$roles = this.getRoles();
final Object other$roles = other.getRoles();
if (this$roles == null ? other$roles != null : !this$roles.equals(other$roles)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $roles = this.getRoles();
result = result * PRIME + ($roles == null ? 43 : $roles.hashCode());
return result;
}
@Override
public String toString() {
return "AccountESDTRoles(roles=" + this.getRoles() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy