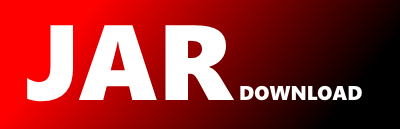
software.crldev.elrondspringbootstarterreactive.api.model.AccountOnNetwork Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import software.crldev.elrondspringbootstarterreactive.domain.ApiModelToDomainConvertible;
import software.crldev.elrondspringbootstarterreactive.domain.account.Account;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.common.Nonce;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.math.BigInteger;
/**
* API response when querying for account info
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = AccountOnNetwork.AccountOnNetworkBuilder.class)
public final class AccountOnNetwork implements ApiModelToDomainConvertible {
@JsonProperty("address")
private final String address;
@JsonProperty("balance")
private final BigInteger balance;
@JsonProperty("developerReward")
private final BigInteger developerReward;
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("username")
private final String username;
@JsonProperty("code")
private final String code;
@JsonProperty("codeHash")
private final String codeHash;
@JsonProperty("codeMetadata")
private final String codeMetadata;
@JsonProperty("ownerAddress")
private final String ownerAddress;
@JsonProperty("rootHash")
private final String rootHash;
@Override
public Account toDomainObject() {
var account = new Account();
account.setAddress(Address.fromBech32(this.getAddress()));
account.setNonce(Nonce.fromLong(this.getNonce()));
account.setBalance(Balance.fromNumber(this.getBalance()));
account.setCode(this.getCode());
account.setUsername(this.getUsername());
return account;
}
AccountOnNetwork(final String address, final BigInteger balance, final BigInteger developerReward, final Long nonce, final String username, final String code, final String codeHash, final String codeMetadata, final String ownerAddress, final String rootHash) {
this.address = address;
this.balance = balance;
this.developerReward = developerReward;
this.nonce = nonce;
this.username = username;
this.code = code;
this.codeHash = codeHash;
this.codeMetadata = codeMetadata;
this.ownerAddress = ownerAddress;
this.rootHash = rootHash;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class AccountOnNetworkBuilder {
private String address;
private BigInteger balance;
private BigInteger developerReward;
private Long nonce;
private String username;
private String code;
private String codeHash;
private String codeMetadata;
private String ownerAddress;
private String rootHash;
AccountOnNetworkBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("address")
public AccountOnNetwork.AccountOnNetworkBuilder address(final String address) {
this.address = address;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("balance")
public AccountOnNetwork.AccountOnNetworkBuilder balance(final BigInteger balance) {
this.balance = balance;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("developerReward")
public AccountOnNetwork.AccountOnNetworkBuilder developerReward(final BigInteger developerReward) {
this.developerReward = developerReward;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
public AccountOnNetwork.AccountOnNetworkBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("username")
public AccountOnNetwork.AccountOnNetworkBuilder username(final String username) {
this.username = username;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("code")
public AccountOnNetwork.AccountOnNetworkBuilder code(final String code) {
this.code = code;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("codeHash")
public AccountOnNetwork.AccountOnNetworkBuilder codeHash(final String codeHash) {
this.codeHash = codeHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("codeMetadata")
public AccountOnNetwork.AccountOnNetworkBuilder codeMetadata(final String codeMetadata) {
this.codeMetadata = codeMetadata;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("ownerAddress")
public AccountOnNetwork.AccountOnNetworkBuilder ownerAddress(final String ownerAddress) {
this.ownerAddress = ownerAddress;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("rootHash")
public AccountOnNetwork.AccountOnNetworkBuilder rootHash(final String rootHash) {
this.rootHash = rootHash;
return this;
}
public AccountOnNetwork build() {
return new AccountOnNetwork(this.address, this.balance, this.developerReward, this.nonce, this.username, this.code, this.codeHash, this.codeMetadata, this.ownerAddress, this.rootHash);
}
@Override
public String toString() {
return "AccountOnNetwork.AccountOnNetworkBuilder(address=" + this.address + ", balance=" + this.balance + ", developerReward=" + this.developerReward + ", nonce=" + this.nonce + ", username=" + this.username + ", code=" + this.code + ", codeHash=" + this.codeHash + ", codeMetadata=" + this.codeMetadata + ", ownerAddress=" + this.ownerAddress + ", rootHash=" + this.rootHash + ")";
}
}
public static AccountOnNetwork.AccountOnNetworkBuilder builder() {
return new AccountOnNetwork.AccountOnNetworkBuilder();
}
public String getAddress() {
return this.address;
}
public BigInteger getBalance() {
return this.balance;
}
public BigInteger getDeveloperReward() {
return this.developerReward;
}
public Long getNonce() {
return this.nonce;
}
public String getUsername() {
return this.username;
}
public String getCode() {
return this.code;
}
public String getCodeHash() {
return this.codeHash;
}
public String getCodeMetadata() {
return this.codeMetadata;
}
public String getOwnerAddress() {
return this.ownerAddress;
}
public String getRootHash() {
return this.rootHash;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof AccountOnNetwork)) return false;
final AccountOnNetwork other = (AccountOnNetwork) o;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$address = this.getAddress();
final Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
final Object this$balance = this.getBalance();
final Object other$balance = other.getBalance();
if (this$balance == null ? other$balance != null : !this$balance.equals(other$balance)) return false;
final Object this$developerReward = this.getDeveloperReward();
final Object other$developerReward = other.getDeveloperReward();
if (this$developerReward == null ? other$developerReward != null : !this$developerReward.equals(other$developerReward)) return false;
final Object this$username = this.getUsername();
final Object other$username = other.getUsername();
if (this$username == null ? other$username != null : !this$username.equals(other$username)) return false;
final Object this$code = this.getCode();
final Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final Object this$codeHash = this.getCodeHash();
final Object other$codeHash = other.getCodeHash();
if (this$codeHash == null ? other$codeHash != null : !this$codeHash.equals(other$codeHash)) return false;
final Object this$codeMetadata = this.getCodeMetadata();
final Object other$codeMetadata = other.getCodeMetadata();
if (this$codeMetadata == null ? other$codeMetadata != null : !this$codeMetadata.equals(other$codeMetadata)) return false;
final Object this$ownerAddress = this.getOwnerAddress();
final Object other$ownerAddress = other.getOwnerAddress();
if (this$ownerAddress == null ? other$ownerAddress != null : !this$ownerAddress.equals(other$ownerAddress)) return false;
final Object this$rootHash = this.getRootHash();
final Object other$rootHash = other.getRootHash();
if (this$rootHash == null ? other$rootHash != null : !this$rootHash.equals(other$rootHash)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
final Object $balance = this.getBalance();
result = result * PRIME + ($balance == null ? 43 : $balance.hashCode());
final Object $developerReward = this.getDeveloperReward();
result = result * PRIME + ($developerReward == null ? 43 : $developerReward.hashCode());
final Object $username = this.getUsername();
result = result * PRIME + ($username == null ? 43 : $username.hashCode());
final Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final Object $codeHash = this.getCodeHash();
result = result * PRIME + ($codeHash == null ? 43 : $codeHash.hashCode());
final Object $codeMetadata = this.getCodeMetadata();
result = result * PRIME + ($codeMetadata == null ? 43 : $codeMetadata.hashCode());
final Object $ownerAddress = this.getOwnerAddress();
result = result * PRIME + ($ownerAddress == null ? 43 : $ownerAddress.hashCode());
final Object $rootHash = this.getRootHash();
result = result * PRIME + ($rootHash == null ? 43 : $rootHash.hashCode());
return result;
}
@Override
public String toString() {
return "AccountOnNetwork(address=" + this.getAddress() + ", balance=" + this.getBalance() + ", developerReward=" + this.getDeveloperReward() + ", nonce=" + this.getNonce() + ", username=" + this.getUsername() + ", code=" + this.getCode() + ", codeHash=" + this.getCodeHash() + ", codeMetadata=" + this.getCodeMetadata() + ", ownerAddress=" + this.getOwnerAddress() + ", rootHash=" + this.getRootHash() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy