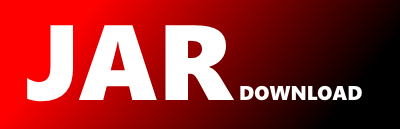
software.crldev.elrondspringbootstarterreactive.api.model.Hyperblock Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.math.BigInteger;
import java.util.List;
/**
* API response when querying for hyperblock data
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = Hyperblock.HyperblockBuilder.class)
public final class Hyperblock {
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("round")
private final Long round;
@JsonProperty("hash")
private final String hash;
@JsonProperty("prevBlockHash")
private final String previousBlockHash;
@JsonProperty("epoch")
private final Long epoch;
@JsonProperty("shardBlocks")
private final List shardBlocks;
@JsonProperty("accumulatedFees")
private final BigInteger accumulatedFees;
@JsonProperty("developerFees")
private final BigInteger developerFees;
@JsonProperty("accumulatedFeesInEpoch")
private final BigInteger accumulatedFeesInEpoch;
@JsonProperty("developerFeesInEpoch")
private final BigInteger developerFeesInEpoch;
@JsonProperty("status")
private final String status;
Hyperblock(final Long nonce, final Long round, final String hash, final String previousBlockHash, final Long epoch, final List shardBlocks, final BigInteger accumulatedFees, final BigInteger developerFees, final BigInteger accumulatedFeesInEpoch, final BigInteger developerFeesInEpoch, final String status) {
this.nonce = nonce;
this.round = round;
this.hash = hash;
this.previousBlockHash = previousBlockHash;
this.epoch = epoch;
this.shardBlocks = shardBlocks;
this.accumulatedFees = accumulatedFees;
this.developerFees = developerFees;
this.accumulatedFeesInEpoch = accumulatedFeesInEpoch;
this.developerFeesInEpoch = developerFeesInEpoch;
this.status = status;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class HyperblockBuilder {
private Long nonce;
private Long round;
private String hash;
private String previousBlockHash;
private Long epoch;
private List shardBlocks;
private BigInteger accumulatedFees;
private BigInteger developerFees;
private BigInteger accumulatedFeesInEpoch;
private BigInteger developerFeesInEpoch;
private String status;
HyperblockBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
public Hyperblock.HyperblockBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("round")
public Hyperblock.HyperblockBuilder round(final Long round) {
this.round = round;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("hash")
public Hyperblock.HyperblockBuilder hash(final String hash) {
this.hash = hash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("prevBlockHash")
public Hyperblock.HyperblockBuilder previousBlockHash(final String previousBlockHash) {
this.previousBlockHash = previousBlockHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("epoch")
public Hyperblock.HyperblockBuilder epoch(final Long epoch) {
this.epoch = epoch;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("shardBlocks")
public Hyperblock.HyperblockBuilder shardBlocks(final List shardBlocks) {
this.shardBlocks = shardBlocks;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("accumulatedFees")
public Hyperblock.HyperblockBuilder accumulatedFees(final BigInteger accumulatedFees) {
this.accumulatedFees = accumulatedFees;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("developerFees")
public Hyperblock.HyperblockBuilder developerFees(final BigInteger developerFees) {
this.developerFees = developerFees;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("accumulatedFeesInEpoch")
public Hyperblock.HyperblockBuilder accumulatedFeesInEpoch(final BigInteger accumulatedFeesInEpoch) {
this.accumulatedFeesInEpoch = accumulatedFeesInEpoch;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("developerFeesInEpoch")
public Hyperblock.HyperblockBuilder developerFeesInEpoch(final BigInteger developerFeesInEpoch) {
this.developerFeesInEpoch = developerFeesInEpoch;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("status")
public Hyperblock.HyperblockBuilder status(final String status) {
this.status = status;
return this;
}
public Hyperblock build() {
return new Hyperblock(this.nonce, this.round, this.hash, this.previousBlockHash, this.epoch, this.shardBlocks, this.accumulatedFees, this.developerFees, this.accumulatedFeesInEpoch, this.developerFeesInEpoch, this.status);
}
@Override
public String toString() {
return "Hyperblock.HyperblockBuilder(nonce=" + this.nonce + ", round=" + this.round + ", hash=" + this.hash + ", previousBlockHash=" + this.previousBlockHash + ", epoch=" + this.epoch + ", shardBlocks=" + this.shardBlocks + ", accumulatedFees=" + this.accumulatedFees + ", developerFees=" + this.developerFees + ", accumulatedFeesInEpoch=" + this.accumulatedFeesInEpoch + ", developerFeesInEpoch=" + this.developerFeesInEpoch + ", status=" + this.status + ")";
}
}
public static Hyperblock.HyperblockBuilder builder() {
return new Hyperblock.HyperblockBuilder();
}
public Long getNonce() {
return this.nonce;
}
public Long getRound() {
return this.round;
}
public String getHash() {
return this.hash;
}
public String getPreviousBlockHash() {
return this.previousBlockHash;
}
public Long getEpoch() {
return this.epoch;
}
public List getShardBlocks() {
return this.shardBlocks;
}
public BigInteger getAccumulatedFees() {
return this.accumulatedFees;
}
public BigInteger getDeveloperFees() {
return this.developerFees;
}
public BigInteger getAccumulatedFeesInEpoch() {
return this.accumulatedFeesInEpoch;
}
public BigInteger getDeveloperFeesInEpoch() {
return this.developerFeesInEpoch;
}
public String getStatus() {
return this.status;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Hyperblock)) return false;
final Hyperblock other = (Hyperblock) o;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$round = this.getRound();
final Object other$round = other.getRound();
if (this$round == null ? other$round != null : !this$round.equals(other$round)) return false;
final Object this$epoch = this.getEpoch();
final Object other$epoch = other.getEpoch();
if (this$epoch == null ? other$epoch != null : !this$epoch.equals(other$epoch)) return false;
final Object this$hash = this.getHash();
final Object other$hash = other.getHash();
if (this$hash == null ? other$hash != null : !this$hash.equals(other$hash)) return false;
final Object this$previousBlockHash = this.getPreviousBlockHash();
final Object other$previousBlockHash = other.getPreviousBlockHash();
if (this$previousBlockHash == null ? other$previousBlockHash != null : !this$previousBlockHash.equals(other$previousBlockHash)) return false;
final Object this$shardBlocks = this.getShardBlocks();
final Object other$shardBlocks = other.getShardBlocks();
if (this$shardBlocks == null ? other$shardBlocks != null : !this$shardBlocks.equals(other$shardBlocks)) return false;
final Object this$accumulatedFees = this.getAccumulatedFees();
final Object other$accumulatedFees = other.getAccumulatedFees();
if (this$accumulatedFees == null ? other$accumulatedFees != null : !this$accumulatedFees.equals(other$accumulatedFees)) return false;
final Object this$developerFees = this.getDeveloperFees();
final Object other$developerFees = other.getDeveloperFees();
if (this$developerFees == null ? other$developerFees != null : !this$developerFees.equals(other$developerFees)) return false;
final Object this$accumulatedFeesInEpoch = this.getAccumulatedFeesInEpoch();
final Object other$accumulatedFeesInEpoch = other.getAccumulatedFeesInEpoch();
if (this$accumulatedFeesInEpoch == null ? other$accumulatedFeesInEpoch != null : !this$accumulatedFeesInEpoch.equals(other$accumulatedFeesInEpoch)) return false;
final Object this$developerFeesInEpoch = this.getDeveloperFeesInEpoch();
final Object other$developerFeesInEpoch = other.getDeveloperFeesInEpoch();
if (this$developerFeesInEpoch == null ? other$developerFeesInEpoch != null : !this$developerFeesInEpoch.equals(other$developerFeesInEpoch)) return false;
final Object this$status = this.getStatus();
final Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $round = this.getRound();
result = result * PRIME + ($round == null ? 43 : $round.hashCode());
final Object $epoch = this.getEpoch();
result = result * PRIME + ($epoch == null ? 43 : $epoch.hashCode());
final Object $hash = this.getHash();
result = result * PRIME + ($hash == null ? 43 : $hash.hashCode());
final Object $previousBlockHash = this.getPreviousBlockHash();
result = result * PRIME + ($previousBlockHash == null ? 43 : $previousBlockHash.hashCode());
final Object $shardBlocks = this.getShardBlocks();
result = result * PRIME + ($shardBlocks == null ? 43 : $shardBlocks.hashCode());
final Object $accumulatedFees = this.getAccumulatedFees();
result = result * PRIME + ($accumulatedFees == null ? 43 : $accumulatedFees.hashCode());
final Object $developerFees = this.getDeveloperFees();
result = result * PRIME + ($developerFees == null ? 43 : $developerFees.hashCode());
final Object $accumulatedFeesInEpoch = this.getAccumulatedFeesInEpoch();
result = result * PRIME + ($accumulatedFeesInEpoch == null ? 43 : $accumulatedFeesInEpoch.hashCode());
final Object $developerFeesInEpoch = this.getDeveloperFeesInEpoch();
result = result * PRIME + ($developerFeesInEpoch == null ? 43 : $developerFeesInEpoch.hashCode());
final Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
@Override
public String toString() {
return "Hyperblock(nonce=" + this.getNonce() + ", round=" + this.getRound() + ", hash=" + this.getHash() + ", previousBlockHash=" + this.getPreviousBlockHash() + ", epoch=" + this.getEpoch() + ", shardBlocks=" + this.getShardBlocks() + ", accumulatedFees=" + this.getAccumulatedFees() + ", developerFees=" + this.getDeveloperFees() + ", accumulatedFeesInEpoch=" + this.getAccumulatedFeesInEpoch() + ", developerFeesInEpoch=" + this.getDeveloperFeesInEpoch() + ", status=" + this.getStatus() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy