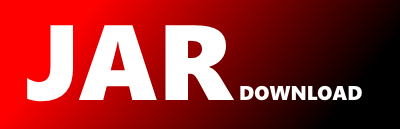
software.crldev.elrondspringbootstarterreactive.api.model.NFTData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.math.BigInteger;
import java.util.List;
/**
* API response when querying for NFT data
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = NFTData.NFTDataBuilder.class)
public final class NFTData {
@JsonProperty("attributes")
private final String attributes;
@JsonProperty("balance")
private final BigInteger balance;
@JsonProperty("creator")
private final String creatorAddress;
@JsonProperty("hash")
private final String hash;
@JsonProperty("name")
private final String name;
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("properties")
private final String properties;
@JsonProperty("royalties")
private final String royalties;
@JsonProperty("tokenIdentifier")
private final String tokenIdentifier;
@JsonProperty("uris")
private final List uris;
NFTData(final String attributes, final BigInteger balance, final String creatorAddress, final String hash, final String name, final Long nonce, final String properties, final String royalties, final String tokenIdentifier, final List uris) {
this.attributes = attributes;
this.balance = balance;
this.creatorAddress = creatorAddress;
this.hash = hash;
this.name = name;
this.nonce = nonce;
this.properties = properties;
this.royalties = royalties;
this.tokenIdentifier = tokenIdentifier;
this.uris = uris;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class NFTDataBuilder {
private String attributes;
private BigInteger balance;
private String creatorAddress;
private String hash;
private String name;
private Long nonce;
private String properties;
private String royalties;
private String tokenIdentifier;
private List uris;
NFTDataBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("attributes")
public NFTData.NFTDataBuilder attributes(final String attributes) {
this.attributes = attributes;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("balance")
public NFTData.NFTDataBuilder balance(final BigInteger balance) {
this.balance = balance;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("creator")
public NFTData.NFTDataBuilder creatorAddress(final String creatorAddress) {
this.creatorAddress = creatorAddress;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("hash")
public NFTData.NFTDataBuilder hash(final String hash) {
this.hash = hash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("name")
public NFTData.NFTDataBuilder name(final String name) {
this.name = name;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
public NFTData.NFTDataBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("properties")
public NFTData.NFTDataBuilder properties(final String properties) {
this.properties = properties;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("royalties")
public NFTData.NFTDataBuilder royalties(final String royalties) {
this.royalties = royalties;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("tokenIdentifier")
public NFTData.NFTDataBuilder tokenIdentifier(final String tokenIdentifier) {
this.tokenIdentifier = tokenIdentifier;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("uris")
public NFTData.NFTDataBuilder uris(final List uris) {
this.uris = uris;
return this;
}
public NFTData build() {
return new NFTData(this.attributes, this.balance, this.creatorAddress, this.hash, this.name, this.nonce, this.properties, this.royalties, this.tokenIdentifier, this.uris);
}
@Override
public String toString() {
return "NFTData.NFTDataBuilder(attributes=" + this.attributes + ", balance=" + this.balance + ", creatorAddress=" + this.creatorAddress + ", hash=" + this.hash + ", name=" + this.name + ", nonce=" + this.nonce + ", properties=" + this.properties + ", royalties=" + this.royalties + ", tokenIdentifier=" + this.tokenIdentifier + ", uris=" + this.uris + ")";
}
}
public static NFTData.NFTDataBuilder builder() {
return new NFTData.NFTDataBuilder();
}
public String getAttributes() {
return this.attributes;
}
public BigInteger getBalance() {
return this.balance;
}
public String getCreatorAddress() {
return this.creatorAddress;
}
public String getHash() {
return this.hash;
}
public String getName() {
return this.name;
}
public Long getNonce() {
return this.nonce;
}
public String getProperties() {
return this.properties;
}
public String getRoyalties() {
return this.royalties;
}
public String getTokenIdentifier() {
return this.tokenIdentifier;
}
public List getUris() {
return this.uris;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof NFTData)) return false;
final NFTData other = (NFTData) o;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$attributes = this.getAttributes();
final Object other$attributes = other.getAttributes();
if (this$attributes == null ? other$attributes != null : !this$attributes.equals(other$attributes)) return false;
final Object this$balance = this.getBalance();
final Object other$balance = other.getBalance();
if (this$balance == null ? other$balance != null : !this$balance.equals(other$balance)) return false;
final Object this$creatorAddress = this.getCreatorAddress();
final Object other$creatorAddress = other.getCreatorAddress();
if (this$creatorAddress == null ? other$creatorAddress != null : !this$creatorAddress.equals(other$creatorAddress)) return false;
final Object this$hash = this.getHash();
final Object other$hash = other.getHash();
if (this$hash == null ? other$hash != null : !this$hash.equals(other$hash)) return false;
final Object this$name = this.getName();
final Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final Object this$properties = this.getProperties();
final Object other$properties = other.getProperties();
if (this$properties == null ? other$properties != null : !this$properties.equals(other$properties)) return false;
final Object this$royalties = this.getRoyalties();
final Object other$royalties = other.getRoyalties();
if (this$royalties == null ? other$royalties != null : !this$royalties.equals(other$royalties)) return false;
final Object this$tokenIdentifier = this.getTokenIdentifier();
final Object other$tokenIdentifier = other.getTokenIdentifier();
if (this$tokenIdentifier == null ? other$tokenIdentifier != null : !this$tokenIdentifier.equals(other$tokenIdentifier)) return false;
final Object this$uris = this.getUris();
final Object other$uris = other.getUris();
if (this$uris == null ? other$uris != null : !this$uris.equals(other$uris)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $attributes = this.getAttributes();
result = result * PRIME + ($attributes == null ? 43 : $attributes.hashCode());
final Object $balance = this.getBalance();
result = result * PRIME + ($balance == null ? 43 : $balance.hashCode());
final Object $creatorAddress = this.getCreatorAddress();
result = result * PRIME + ($creatorAddress == null ? 43 : $creatorAddress.hashCode());
final Object $hash = this.getHash();
result = result * PRIME + ($hash == null ? 43 : $hash.hashCode());
final Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final Object $properties = this.getProperties();
result = result * PRIME + ($properties == null ? 43 : $properties.hashCode());
final Object $royalties = this.getRoyalties();
result = result * PRIME + ($royalties == null ? 43 : $royalties.hashCode());
final Object $tokenIdentifier = this.getTokenIdentifier();
result = result * PRIME + ($tokenIdentifier == null ? 43 : $tokenIdentifier.hashCode());
final Object $uris = this.getUris();
result = result * PRIME + ($uris == null ? 43 : $uris.hashCode());
return result;
}
@Override
public String toString() {
return "NFTData(attributes=" + this.getAttributes() + ", balance=" + this.getBalance() + ", creatorAddress=" + this.getCreatorAddress() + ", hash=" + this.getHash() + ", name=" + this.getName() + ", nonce=" + this.getNonce() + ", properties=" + this.getProperties() + ", royalties=" + this.getRoyalties() + ", tokenIdentifier=" + this.getTokenIdentifier() + ", uris=" + this.getUris() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy