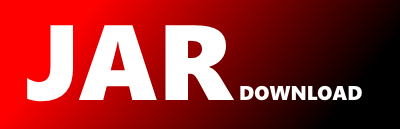
software.crldev.elrondspringbootstarterreactive.api.model.NetworkConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.math.BigInteger;
/**
* API response when querying for network configuration
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = NetworkConfig.NetworkConfigBuilder.class)
public final class NetworkConfig {
@JsonProperty("erd_chain_id")
private final String chainId;
@JsonProperty("erd_denomination")
private final Long denomination;
@JsonProperty("erd_gas_per_data_byte")
private final Long gasPerDataByte;
@JsonProperty("erd_gas_price_modifier")
private final Double gasPriceModifier;
@JsonProperty("erd_latest_tag_software_version")
private final String latestTagSoftwareVersion;
@JsonProperty("erd_meta_consensus_group_size")
private final Integer metaConsensusGroupSize;
@JsonProperty("erd_min_gas_limit")
private final BigInteger minGasLimit;
@JsonProperty("erd_min_gas_price")
private final BigInteger minGasPrice;
@JsonProperty("erd_min_transaction_version")
private final Integer minTransactionVersion;
@JsonProperty("erd_num_metachain_nodes")
private final Integer numMetachainNodes;
@JsonProperty("erd_num_nodes_in_shard")
private final Integer numNodesInShard;
@JsonProperty("erd_num_shards_without_meta")
private final Integer numShardsWithoutMeta;
@JsonProperty("erd_rewards_top_up_gradient_poInteger")
private final BigInteger rewardsTopUpGradientPoInteger;
@JsonProperty("erd_round_duration")
private final Long roundDuration;
@JsonProperty("erd_rounds_per_epoch")
private final Long roundPerEpoch;
@JsonProperty("erd_shard_consensus_group_size")
private final Integer shardConsensusGroupSize;
@JsonProperty("erd_start_time")
private final Long startTime;
@JsonProperty("erd_top_up_factor")
private final Double topUpFactor;
NetworkConfig(final String chainId, final Long denomination, final Long gasPerDataByte, final Double gasPriceModifier, final String latestTagSoftwareVersion, final Integer metaConsensusGroupSize, final BigInteger minGasLimit, final BigInteger minGasPrice, final Integer minTransactionVersion, final Integer numMetachainNodes, final Integer numNodesInShard, final Integer numShardsWithoutMeta, final BigInteger rewardsTopUpGradientPoInteger, final Long roundDuration, final Long roundPerEpoch, final Integer shardConsensusGroupSize, final Long startTime, final Double topUpFactor) {
this.chainId = chainId;
this.denomination = denomination;
this.gasPerDataByte = gasPerDataByte;
this.gasPriceModifier = gasPriceModifier;
this.latestTagSoftwareVersion = latestTagSoftwareVersion;
this.metaConsensusGroupSize = metaConsensusGroupSize;
this.minGasLimit = minGasLimit;
this.minGasPrice = minGasPrice;
this.minTransactionVersion = minTransactionVersion;
this.numMetachainNodes = numMetachainNodes;
this.numNodesInShard = numNodesInShard;
this.numShardsWithoutMeta = numShardsWithoutMeta;
this.rewardsTopUpGradientPoInteger = rewardsTopUpGradientPoInteger;
this.roundDuration = roundDuration;
this.roundPerEpoch = roundPerEpoch;
this.shardConsensusGroupSize = shardConsensusGroupSize;
this.startTime = startTime;
this.topUpFactor = topUpFactor;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class NetworkConfigBuilder {
private String chainId;
private Long denomination;
private Long gasPerDataByte;
private Double gasPriceModifier;
private String latestTagSoftwareVersion;
private Integer metaConsensusGroupSize;
private BigInteger minGasLimit;
private BigInteger minGasPrice;
private Integer minTransactionVersion;
private Integer numMetachainNodes;
private Integer numNodesInShard;
private Integer numShardsWithoutMeta;
private BigInteger rewardsTopUpGradientPoInteger;
private Long roundDuration;
private Long roundPerEpoch;
private Integer shardConsensusGroupSize;
private Long startTime;
private Double topUpFactor;
NetworkConfigBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_chain_id")
public NetworkConfig.NetworkConfigBuilder chainId(final String chainId) {
this.chainId = chainId;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_denomination")
public NetworkConfig.NetworkConfigBuilder denomination(final Long denomination) {
this.denomination = denomination;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_gas_per_data_byte")
public NetworkConfig.NetworkConfigBuilder gasPerDataByte(final Long gasPerDataByte) {
this.gasPerDataByte = gasPerDataByte;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_gas_price_modifier")
public NetworkConfig.NetworkConfigBuilder gasPriceModifier(final Double gasPriceModifier) {
this.gasPriceModifier = gasPriceModifier;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_latest_tag_software_version")
public NetworkConfig.NetworkConfigBuilder latestTagSoftwareVersion(final String latestTagSoftwareVersion) {
this.latestTagSoftwareVersion = latestTagSoftwareVersion;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_meta_consensus_group_size")
public NetworkConfig.NetworkConfigBuilder metaConsensusGroupSize(final Integer metaConsensusGroupSize) {
this.metaConsensusGroupSize = metaConsensusGroupSize;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_min_gas_limit")
public NetworkConfig.NetworkConfigBuilder minGasLimit(final BigInteger minGasLimit) {
this.minGasLimit = minGasLimit;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_min_gas_price")
public NetworkConfig.NetworkConfigBuilder minGasPrice(final BigInteger minGasPrice) {
this.minGasPrice = minGasPrice;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_min_transaction_version")
public NetworkConfig.NetworkConfigBuilder minTransactionVersion(final Integer minTransactionVersion) {
this.minTransactionVersion = minTransactionVersion;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_num_metachain_nodes")
public NetworkConfig.NetworkConfigBuilder numMetachainNodes(final Integer numMetachainNodes) {
this.numMetachainNodes = numMetachainNodes;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_num_nodes_in_shard")
public NetworkConfig.NetworkConfigBuilder numNodesInShard(final Integer numNodesInShard) {
this.numNodesInShard = numNodesInShard;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_num_shards_without_meta")
public NetworkConfig.NetworkConfigBuilder numShardsWithoutMeta(final Integer numShardsWithoutMeta) {
this.numShardsWithoutMeta = numShardsWithoutMeta;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_rewards_top_up_gradient_poInteger")
public NetworkConfig.NetworkConfigBuilder rewardsTopUpGradientPoInteger(final BigInteger rewardsTopUpGradientPoInteger) {
this.rewardsTopUpGradientPoInteger = rewardsTopUpGradientPoInteger;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_round_duration")
public NetworkConfig.NetworkConfigBuilder roundDuration(final Long roundDuration) {
this.roundDuration = roundDuration;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_rounds_per_epoch")
public NetworkConfig.NetworkConfigBuilder roundPerEpoch(final Long roundPerEpoch) {
this.roundPerEpoch = roundPerEpoch;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_shard_consensus_group_size")
public NetworkConfig.NetworkConfigBuilder shardConsensusGroupSize(final Integer shardConsensusGroupSize) {
this.shardConsensusGroupSize = shardConsensusGroupSize;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_start_time")
public NetworkConfig.NetworkConfigBuilder startTime(final Long startTime) {
this.startTime = startTime;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_top_up_factor")
public NetworkConfig.NetworkConfigBuilder topUpFactor(final Double topUpFactor) {
this.topUpFactor = topUpFactor;
return this;
}
public NetworkConfig build() {
return new NetworkConfig(this.chainId, this.denomination, this.gasPerDataByte, this.gasPriceModifier, this.latestTagSoftwareVersion, this.metaConsensusGroupSize, this.minGasLimit, this.minGasPrice, this.minTransactionVersion, this.numMetachainNodes, this.numNodesInShard, this.numShardsWithoutMeta, this.rewardsTopUpGradientPoInteger, this.roundDuration, this.roundPerEpoch, this.shardConsensusGroupSize, this.startTime, this.topUpFactor);
}
@Override
public String toString() {
return "NetworkConfig.NetworkConfigBuilder(chainId=" + this.chainId + ", denomination=" + this.denomination + ", gasPerDataByte=" + this.gasPerDataByte + ", gasPriceModifier=" + this.gasPriceModifier + ", latestTagSoftwareVersion=" + this.latestTagSoftwareVersion + ", metaConsensusGroupSize=" + this.metaConsensusGroupSize + ", minGasLimit=" + this.minGasLimit + ", minGasPrice=" + this.minGasPrice + ", minTransactionVersion=" + this.minTransactionVersion + ", numMetachainNodes=" + this.numMetachainNodes + ", numNodesInShard=" + this.numNodesInShard + ", numShardsWithoutMeta=" + this.numShardsWithoutMeta + ", rewardsTopUpGradientPoInteger=" + this.rewardsTopUpGradientPoInteger + ", roundDuration=" + this.roundDuration + ", roundPerEpoch=" + this.roundPerEpoch + ", shardConsensusGroupSize=" + this.shardConsensusGroupSize + ", startTime=" + this.startTime + ", topUpFactor=" + this.topUpFactor + ")";
}
}
public static NetworkConfig.NetworkConfigBuilder builder() {
return new NetworkConfig.NetworkConfigBuilder();
}
public String getChainId() {
return this.chainId;
}
public Long getDenomination() {
return this.denomination;
}
public Long getGasPerDataByte() {
return this.gasPerDataByte;
}
public Double getGasPriceModifier() {
return this.gasPriceModifier;
}
public String getLatestTagSoftwareVersion() {
return this.latestTagSoftwareVersion;
}
public Integer getMetaConsensusGroupSize() {
return this.metaConsensusGroupSize;
}
public BigInteger getMinGasLimit() {
return this.minGasLimit;
}
public BigInteger getMinGasPrice() {
return this.minGasPrice;
}
public Integer getMinTransactionVersion() {
return this.minTransactionVersion;
}
public Integer getNumMetachainNodes() {
return this.numMetachainNodes;
}
public Integer getNumNodesInShard() {
return this.numNodesInShard;
}
public Integer getNumShardsWithoutMeta() {
return this.numShardsWithoutMeta;
}
public BigInteger getRewardsTopUpGradientPoInteger() {
return this.rewardsTopUpGradientPoInteger;
}
public Long getRoundDuration() {
return this.roundDuration;
}
public Long getRoundPerEpoch() {
return this.roundPerEpoch;
}
public Integer getShardConsensusGroupSize() {
return this.shardConsensusGroupSize;
}
public Long getStartTime() {
return this.startTime;
}
public Double getTopUpFactor() {
return this.topUpFactor;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof NetworkConfig)) return false;
final NetworkConfig other = (NetworkConfig) o;
final Object this$denomination = this.getDenomination();
final Object other$denomination = other.getDenomination();
if (this$denomination == null ? other$denomination != null : !this$denomination.equals(other$denomination)) return false;
final Object this$gasPerDataByte = this.getGasPerDataByte();
final Object other$gasPerDataByte = other.getGasPerDataByte();
if (this$gasPerDataByte == null ? other$gasPerDataByte != null : !this$gasPerDataByte.equals(other$gasPerDataByte)) return false;
final Object this$gasPriceModifier = this.getGasPriceModifier();
final Object other$gasPriceModifier = other.getGasPriceModifier();
if (this$gasPriceModifier == null ? other$gasPriceModifier != null : !this$gasPriceModifier.equals(other$gasPriceModifier)) return false;
final Object this$metaConsensusGroupSize = this.getMetaConsensusGroupSize();
final Object other$metaConsensusGroupSize = other.getMetaConsensusGroupSize();
if (this$metaConsensusGroupSize == null ? other$metaConsensusGroupSize != null : !this$metaConsensusGroupSize.equals(other$metaConsensusGroupSize)) return false;
final Object this$minTransactionVersion = this.getMinTransactionVersion();
final Object other$minTransactionVersion = other.getMinTransactionVersion();
if (this$minTransactionVersion == null ? other$minTransactionVersion != null : !this$minTransactionVersion.equals(other$minTransactionVersion)) return false;
final Object this$numMetachainNodes = this.getNumMetachainNodes();
final Object other$numMetachainNodes = other.getNumMetachainNodes();
if (this$numMetachainNodes == null ? other$numMetachainNodes != null : !this$numMetachainNodes.equals(other$numMetachainNodes)) return false;
final Object this$numNodesInShard = this.getNumNodesInShard();
final Object other$numNodesInShard = other.getNumNodesInShard();
if (this$numNodesInShard == null ? other$numNodesInShard != null : !this$numNodesInShard.equals(other$numNodesInShard)) return false;
final Object this$numShardsWithoutMeta = this.getNumShardsWithoutMeta();
final Object other$numShardsWithoutMeta = other.getNumShardsWithoutMeta();
if (this$numShardsWithoutMeta == null ? other$numShardsWithoutMeta != null : !this$numShardsWithoutMeta.equals(other$numShardsWithoutMeta)) return false;
final Object this$roundDuration = this.getRoundDuration();
final Object other$roundDuration = other.getRoundDuration();
if (this$roundDuration == null ? other$roundDuration != null : !this$roundDuration.equals(other$roundDuration)) return false;
final Object this$roundPerEpoch = this.getRoundPerEpoch();
final Object other$roundPerEpoch = other.getRoundPerEpoch();
if (this$roundPerEpoch == null ? other$roundPerEpoch != null : !this$roundPerEpoch.equals(other$roundPerEpoch)) return false;
final Object this$shardConsensusGroupSize = this.getShardConsensusGroupSize();
final Object other$shardConsensusGroupSize = other.getShardConsensusGroupSize();
if (this$shardConsensusGroupSize == null ? other$shardConsensusGroupSize != null : !this$shardConsensusGroupSize.equals(other$shardConsensusGroupSize)) return false;
final Object this$startTime = this.getStartTime();
final Object other$startTime = other.getStartTime();
if (this$startTime == null ? other$startTime != null : !this$startTime.equals(other$startTime)) return false;
final Object this$topUpFactor = this.getTopUpFactor();
final Object other$topUpFactor = other.getTopUpFactor();
if (this$topUpFactor == null ? other$topUpFactor != null : !this$topUpFactor.equals(other$topUpFactor)) return false;
final Object this$chainId = this.getChainId();
final Object other$chainId = other.getChainId();
if (this$chainId == null ? other$chainId != null : !this$chainId.equals(other$chainId)) return false;
final Object this$latestTagSoftwareVersion = this.getLatestTagSoftwareVersion();
final Object other$latestTagSoftwareVersion = other.getLatestTagSoftwareVersion();
if (this$latestTagSoftwareVersion == null ? other$latestTagSoftwareVersion != null : !this$latestTagSoftwareVersion.equals(other$latestTagSoftwareVersion)) return false;
final Object this$minGasLimit = this.getMinGasLimit();
final Object other$minGasLimit = other.getMinGasLimit();
if (this$minGasLimit == null ? other$minGasLimit != null : !this$minGasLimit.equals(other$minGasLimit)) return false;
final Object this$minGasPrice = this.getMinGasPrice();
final Object other$minGasPrice = other.getMinGasPrice();
if (this$minGasPrice == null ? other$minGasPrice != null : !this$minGasPrice.equals(other$minGasPrice)) return false;
final Object this$rewardsTopUpGradientPoInteger = this.getRewardsTopUpGradientPoInteger();
final Object other$rewardsTopUpGradientPoInteger = other.getRewardsTopUpGradientPoInteger();
if (this$rewardsTopUpGradientPoInteger == null ? other$rewardsTopUpGradientPoInteger != null : !this$rewardsTopUpGradientPoInteger.equals(other$rewardsTopUpGradientPoInteger)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $denomination = this.getDenomination();
result = result * PRIME + ($denomination == null ? 43 : $denomination.hashCode());
final Object $gasPerDataByte = this.getGasPerDataByte();
result = result * PRIME + ($gasPerDataByte == null ? 43 : $gasPerDataByte.hashCode());
final Object $gasPriceModifier = this.getGasPriceModifier();
result = result * PRIME + ($gasPriceModifier == null ? 43 : $gasPriceModifier.hashCode());
final Object $metaConsensusGroupSize = this.getMetaConsensusGroupSize();
result = result * PRIME + ($metaConsensusGroupSize == null ? 43 : $metaConsensusGroupSize.hashCode());
final Object $minTransactionVersion = this.getMinTransactionVersion();
result = result * PRIME + ($minTransactionVersion == null ? 43 : $minTransactionVersion.hashCode());
final Object $numMetachainNodes = this.getNumMetachainNodes();
result = result * PRIME + ($numMetachainNodes == null ? 43 : $numMetachainNodes.hashCode());
final Object $numNodesInShard = this.getNumNodesInShard();
result = result * PRIME + ($numNodesInShard == null ? 43 : $numNodesInShard.hashCode());
final Object $numShardsWithoutMeta = this.getNumShardsWithoutMeta();
result = result * PRIME + ($numShardsWithoutMeta == null ? 43 : $numShardsWithoutMeta.hashCode());
final Object $roundDuration = this.getRoundDuration();
result = result * PRIME + ($roundDuration == null ? 43 : $roundDuration.hashCode());
final Object $roundPerEpoch = this.getRoundPerEpoch();
result = result * PRIME + ($roundPerEpoch == null ? 43 : $roundPerEpoch.hashCode());
final Object $shardConsensusGroupSize = this.getShardConsensusGroupSize();
result = result * PRIME + ($shardConsensusGroupSize == null ? 43 : $shardConsensusGroupSize.hashCode());
final Object $startTime = this.getStartTime();
result = result * PRIME + ($startTime == null ? 43 : $startTime.hashCode());
final Object $topUpFactor = this.getTopUpFactor();
result = result * PRIME + ($topUpFactor == null ? 43 : $topUpFactor.hashCode());
final Object $chainId = this.getChainId();
result = result * PRIME + ($chainId == null ? 43 : $chainId.hashCode());
final Object $latestTagSoftwareVersion = this.getLatestTagSoftwareVersion();
result = result * PRIME + ($latestTagSoftwareVersion == null ? 43 : $latestTagSoftwareVersion.hashCode());
final Object $minGasLimit = this.getMinGasLimit();
result = result * PRIME + ($minGasLimit == null ? 43 : $minGasLimit.hashCode());
final Object $minGasPrice = this.getMinGasPrice();
result = result * PRIME + ($minGasPrice == null ? 43 : $minGasPrice.hashCode());
final Object $rewardsTopUpGradientPoInteger = this.getRewardsTopUpGradientPoInteger();
result = result * PRIME + ($rewardsTopUpGradientPoInteger == null ? 43 : $rewardsTopUpGradientPoInteger.hashCode());
return result;
}
@Override
public String toString() {
return "NetworkConfig(chainId=" + this.getChainId() + ", denomination=" + this.getDenomination() + ", gasPerDataByte=" + this.getGasPerDataByte() + ", gasPriceModifier=" + this.getGasPriceModifier() + ", latestTagSoftwareVersion=" + this.getLatestTagSoftwareVersion() + ", metaConsensusGroupSize=" + this.getMetaConsensusGroupSize() + ", minGasLimit=" + this.getMinGasLimit() + ", minGasPrice=" + this.getMinGasPrice() + ", minTransactionVersion=" + this.getMinTransactionVersion() + ", numMetachainNodes=" + this.getNumMetachainNodes() + ", numNodesInShard=" + this.getNumNodesInShard() + ", numShardsWithoutMeta=" + this.getNumShardsWithoutMeta() + ", rewardsTopUpGradientPoInteger=" + this.getRewardsTopUpGradientPoInteger() + ", roundDuration=" + this.getRoundDuration() + ", roundPerEpoch=" + this.getRoundPerEpoch() + ", shardConsensusGroupSize=" + this.getShardConsensusGroupSize() + ", startTime=" + this.getStartTime() + ", topUpFactor=" + this.getTopUpFactor() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy