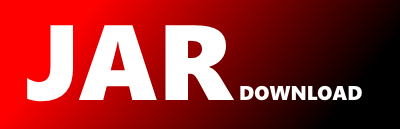
software.crldev.elrondspringbootstarterreactive.api.model.NodeHeartbeatStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.Instant;
/**
* API response when querying for node status
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = NodeHeartbeatStatus.NodeHeartbeatStatusBuilder.class)
public final class NodeHeartbeatStatus {
@JsonProperty("timeStamp")
private final Instant timestamp;
@JsonProperty("publicKey")
private final String publicKey;
@JsonProperty("versionNumber")
private final String versionNumber;
@JsonProperty("nodeDisplayName")
private final String nodeDisplayName;
@JsonProperty("identity")
private final String identity;
@JsonProperty("totalUpTimeSec")
private final Long totalUpTimeSeconds;
@JsonProperty("totalDownTimeSec")
private final Long totalDownTimeSeconds;
@JsonProperty("maxInactiveTime")
private final String maxInactiveTime;
@JsonProperty("receivedShardID")
private final Long receivedShardId;
@JsonProperty("computedShardID")
private final Long computedShardId;
@JsonProperty("peerType")
private final String peerType;
@JsonProperty("isActive")
private final Boolean isActive;
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("numInstances")
private final Long numOfInstances;
NodeHeartbeatStatus(final Instant timestamp, final String publicKey, final String versionNumber, final String nodeDisplayName, final String identity, final Long totalUpTimeSeconds, final Long totalDownTimeSeconds, final String maxInactiveTime, final Long receivedShardId, final Long computedShardId, final String peerType, final Boolean isActive, final Long nonce, final Long numOfInstances) {
this.timestamp = timestamp;
this.publicKey = publicKey;
this.versionNumber = versionNumber;
this.nodeDisplayName = nodeDisplayName;
this.identity = identity;
this.totalUpTimeSeconds = totalUpTimeSeconds;
this.totalDownTimeSeconds = totalDownTimeSeconds;
this.maxInactiveTime = maxInactiveTime;
this.receivedShardId = receivedShardId;
this.computedShardId = computedShardId;
this.peerType = peerType;
this.isActive = isActive;
this.nonce = nonce;
this.numOfInstances = numOfInstances;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class NodeHeartbeatStatusBuilder {
private Instant timestamp;
private String publicKey;
private String versionNumber;
private String nodeDisplayName;
private String identity;
private Long totalUpTimeSeconds;
private Long totalDownTimeSeconds;
private String maxInactiveTime;
private Long receivedShardId;
private Long computedShardId;
private String peerType;
private Boolean isActive;
private Long nonce;
private Long numOfInstances;
NodeHeartbeatStatusBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("timeStamp")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder timestamp(final Instant timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("publicKey")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder publicKey(final String publicKey) {
this.publicKey = publicKey;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("versionNumber")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder versionNumber(final String versionNumber) {
this.versionNumber = versionNumber;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("nodeDisplayName")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder nodeDisplayName(final String nodeDisplayName) {
this.nodeDisplayName = nodeDisplayName;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("identity")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder identity(final String identity) {
this.identity = identity;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("totalUpTimeSec")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder totalUpTimeSeconds(final Long totalUpTimeSeconds) {
this.totalUpTimeSeconds = totalUpTimeSeconds;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("totalDownTimeSec")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder totalDownTimeSeconds(final Long totalDownTimeSeconds) {
this.totalDownTimeSeconds = totalDownTimeSeconds;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("maxInactiveTime")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder maxInactiveTime(final String maxInactiveTime) {
this.maxInactiveTime = maxInactiveTime;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("receivedShardID")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder receivedShardId(final Long receivedShardId) {
this.receivedShardId = receivedShardId;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("computedShardID")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder computedShardId(final Long computedShardId) {
this.computedShardId = computedShardId;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("peerType")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder peerType(final String peerType) {
this.peerType = peerType;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("isActive")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder isActive(final Boolean isActive) {
this.isActive = isActive;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("numInstances")
public NodeHeartbeatStatus.NodeHeartbeatStatusBuilder numOfInstances(final Long numOfInstances) {
this.numOfInstances = numOfInstances;
return this;
}
public NodeHeartbeatStatus build() {
return new NodeHeartbeatStatus(this.timestamp, this.publicKey, this.versionNumber, this.nodeDisplayName, this.identity, this.totalUpTimeSeconds, this.totalDownTimeSeconds, this.maxInactiveTime, this.receivedShardId, this.computedShardId, this.peerType, this.isActive, this.nonce, this.numOfInstances);
}
@Override
public String toString() {
return "NodeHeartbeatStatus.NodeHeartbeatStatusBuilder(timestamp=" + this.timestamp + ", publicKey=" + this.publicKey + ", versionNumber=" + this.versionNumber + ", nodeDisplayName=" + this.nodeDisplayName + ", identity=" + this.identity + ", totalUpTimeSeconds=" + this.totalUpTimeSeconds + ", totalDownTimeSeconds=" + this.totalDownTimeSeconds + ", maxInactiveTime=" + this.maxInactiveTime + ", receivedShardId=" + this.receivedShardId + ", computedShardId=" + this.computedShardId + ", peerType=" + this.peerType + ", isActive=" + this.isActive + ", nonce=" + this.nonce + ", numOfInstances=" + this.numOfInstances + ")";
}
}
public static NodeHeartbeatStatus.NodeHeartbeatStatusBuilder builder() {
return new NodeHeartbeatStatus.NodeHeartbeatStatusBuilder();
}
public Instant getTimestamp() {
return this.timestamp;
}
public String getPublicKey() {
return this.publicKey;
}
public String getVersionNumber() {
return this.versionNumber;
}
public String getNodeDisplayName() {
return this.nodeDisplayName;
}
public String getIdentity() {
return this.identity;
}
public Long getTotalUpTimeSeconds() {
return this.totalUpTimeSeconds;
}
public Long getTotalDownTimeSeconds() {
return this.totalDownTimeSeconds;
}
public String getMaxInactiveTime() {
return this.maxInactiveTime;
}
public Long getReceivedShardId() {
return this.receivedShardId;
}
public Long getComputedShardId() {
return this.computedShardId;
}
public String getPeerType() {
return this.peerType;
}
public Boolean getIsActive() {
return this.isActive;
}
public Long getNonce() {
return this.nonce;
}
public Long getNumOfInstances() {
return this.numOfInstances;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof NodeHeartbeatStatus)) return false;
final NodeHeartbeatStatus other = (NodeHeartbeatStatus) o;
final Object this$totalUpTimeSeconds = this.getTotalUpTimeSeconds();
final Object other$totalUpTimeSeconds = other.getTotalUpTimeSeconds();
if (this$totalUpTimeSeconds == null ? other$totalUpTimeSeconds != null : !this$totalUpTimeSeconds.equals(other$totalUpTimeSeconds)) return false;
final Object this$totalDownTimeSeconds = this.getTotalDownTimeSeconds();
final Object other$totalDownTimeSeconds = other.getTotalDownTimeSeconds();
if (this$totalDownTimeSeconds == null ? other$totalDownTimeSeconds != null : !this$totalDownTimeSeconds.equals(other$totalDownTimeSeconds)) return false;
final Object this$receivedShardId = this.getReceivedShardId();
final Object other$receivedShardId = other.getReceivedShardId();
if (this$receivedShardId == null ? other$receivedShardId != null : !this$receivedShardId.equals(other$receivedShardId)) return false;
final Object this$computedShardId = this.getComputedShardId();
final Object other$computedShardId = other.getComputedShardId();
if (this$computedShardId == null ? other$computedShardId != null : !this$computedShardId.equals(other$computedShardId)) return false;
final Object this$isActive = this.getIsActive();
final Object other$isActive = other.getIsActive();
if (this$isActive == null ? other$isActive != null : !this$isActive.equals(other$isActive)) return false;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$numOfInstances = this.getNumOfInstances();
final Object other$numOfInstances = other.getNumOfInstances();
if (this$numOfInstances == null ? other$numOfInstances != null : !this$numOfInstances.equals(other$numOfInstances)) return false;
final Object this$timestamp = this.getTimestamp();
final Object other$timestamp = other.getTimestamp();
if (this$timestamp == null ? other$timestamp != null : !this$timestamp.equals(other$timestamp)) return false;
final Object this$publicKey = this.getPublicKey();
final Object other$publicKey = other.getPublicKey();
if (this$publicKey == null ? other$publicKey != null : !this$publicKey.equals(other$publicKey)) return false;
final Object this$versionNumber = this.getVersionNumber();
final Object other$versionNumber = other.getVersionNumber();
if (this$versionNumber == null ? other$versionNumber != null : !this$versionNumber.equals(other$versionNumber)) return false;
final Object this$nodeDisplayName = this.getNodeDisplayName();
final Object other$nodeDisplayName = other.getNodeDisplayName();
if (this$nodeDisplayName == null ? other$nodeDisplayName != null : !this$nodeDisplayName.equals(other$nodeDisplayName)) return false;
final Object this$identity = this.getIdentity();
final Object other$identity = other.getIdentity();
if (this$identity == null ? other$identity != null : !this$identity.equals(other$identity)) return false;
final Object this$maxInactiveTime = this.getMaxInactiveTime();
final Object other$maxInactiveTime = other.getMaxInactiveTime();
if (this$maxInactiveTime == null ? other$maxInactiveTime != null : !this$maxInactiveTime.equals(other$maxInactiveTime)) return false;
final Object this$peerType = this.getPeerType();
final Object other$peerType = other.getPeerType();
if (this$peerType == null ? other$peerType != null : !this$peerType.equals(other$peerType)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $totalUpTimeSeconds = this.getTotalUpTimeSeconds();
result = result * PRIME + ($totalUpTimeSeconds == null ? 43 : $totalUpTimeSeconds.hashCode());
final Object $totalDownTimeSeconds = this.getTotalDownTimeSeconds();
result = result * PRIME + ($totalDownTimeSeconds == null ? 43 : $totalDownTimeSeconds.hashCode());
final Object $receivedShardId = this.getReceivedShardId();
result = result * PRIME + ($receivedShardId == null ? 43 : $receivedShardId.hashCode());
final Object $computedShardId = this.getComputedShardId();
result = result * PRIME + ($computedShardId == null ? 43 : $computedShardId.hashCode());
final Object $isActive = this.getIsActive();
result = result * PRIME + ($isActive == null ? 43 : $isActive.hashCode());
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $numOfInstances = this.getNumOfInstances();
result = result * PRIME + ($numOfInstances == null ? 43 : $numOfInstances.hashCode());
final Object $timestamp = this.getTimestamp();
result = result * PRIME + ($timestamp == null ? 43 : $timestamp.hashCode());
final Object $publicKey = this.getPublicKey();
result = result * PRIME + ($publicKey == null ? 43 : $publicKey.hashCode());
final Object $versionNumber = this.getVersionNumber();
result = result * PRIME + ($versionNumber == null ? 43 : $versionNumber.hashCode());
final Object $nodeDisplayName = this.getNodeDisplayName();
result = result * PRIME + ($nodeDisplayName == null ? 43 : $nodeDisplayName.hashCode());
final Object $identity = this.getIdentity();
result = result * PRIME + ($identity == null ? 43 : $identity.hashCode());
final Object $maxInactiveTime = this.getMaxInactiveTime();
result = result * PRIME + ($maxInactiveTime == null ? 43 : $maxInactiveTime.hashCode());
final Object $peerType = this.getPeerType();
result = result * PRIME + ($peerType == null ? 43 : $peerType.hashCode());
return result;
}
@Override
public String toString() {
return "NodeHeartbeatStatus(timestamp=" + this.getTimestamp() + ", publicKey=" + this.getPublicKey() + ", versionNumber=" + this.getVersionNumber() + ", nodeDisplayName=" + this.getNodeDisplayName() + ", identity=" + this.getIdentity() + ", totalUpTimeSeconds=" + this.getTotalUpTimeSeconds() + ", totalDownTimeSeconds=" + this.getTotalDownTimeSeconds() + ", maxInactiveTime=" + this.getMaxInactiveTime() + ", receivedShardId=" + this.getReceivedShardId() + ", computedShardId=" + this.getComputedShardId() + ", peerType=" + this.getPeerType() + ", isActive=" + this.getIsActive() + ", nonce=" + this.getNonce() + ", numOfInstances=" + this.getNumOfInstances() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy