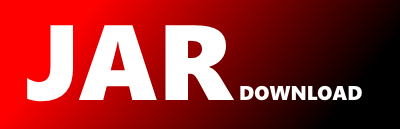
software.crldev.elrondspringbootstarterreactive.api.model.ShardBlock Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.math.BigInteger;
/**
* API response when querying for block shard data
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = ShardBlock.ShardBlockBuilder.class)
public final class ShardBlock {
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("round")
private final Long round;
@JsonProperty("hash")
private final String hash;
@JsonProperty("prevBlockHash")
private final String previousBlockHash;
@JsonProperty("epoch")
private final Long epoch;
@JsonProperty("shard")
private final Integer shard;
@JsonProperty("accumulatedFees")
private final BigInteger accumulatedFees;
@JsonProperty("developerFees")
private final BigInteger developerFees;
@JsonProperty("status")
private final String status;
ShardBlock(final Long nonce, final Long round, final String hash, final String previousBlockHash, final Long epoch, final Integer shard, final BigInteger accumulatedFees, final BigInteger developerFees, final String status) {
this.nonce = nonce;
this.round = round;
this.hash = hash;
this.previousBlockHash = previousBlockHash;
this.epoch = epoch;
this.shard = shard;
this.accumulatedFees = accumulatedFees;
this.developerFees = developerFees;
this.status = status;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class ShardBlockBuilder {
private Long nonce;
private Long round;
private String hash;
private String previousBlockHash;
private Long epoch;
private Integer shard;
private BigInteger accumulatedFees;
private BigInteger developerFees;
private String status;
ShardBlockBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
public ShardBlock.ShardBlockBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("round")
public ShardBlock.ShardBlockBuilder round(final Long round) {
this.round = round;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("hash")
public ShardBlock.ShardBlockBuilder hash(final String hash) {
this.hash = hash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("prevBlockHash")
public ShardBlock.ShardBlockBuilder previousBlockHash(final String previousBlockHash) {
this.previousBlockHash = previousBlockHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("epoch")
public ShardBlock.ShardBlockBuilder epoch(final Long epoch) {
this.epoch = epoch;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("shard")
public ShardBlock.ShardBlockBuilder shard(final Integer shard) {
this.shard = shard;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("accumulatedFees")
public ShardBlock.ShardBlockBuilder accumulatedFees(final BigInteger accumulatedFees) {
this.accumulatedFees = accumulatedFees;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("developerFees")
public ShardBlock.ShardBlockBuilder developerFees(final BigInteger developerFees) {
this.developerFees = developerFees;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("status")
public ShardBlock.ShardBlockBuilder status(final String status) {
this.status = status;
return this;
}
public ShardBlock build() {
return new ShardBlock(this.nonce, this.round, this.hash, this.previousBlockHash, this.epoch, this.shard, this.accumulatedFees, this.developerFees, this.status);
}
@Override
public String toString() {
return "ShardBlock.ShardBlockBuilder(nonce=" + this.nonce + ", round=" + this.round + ", hash=" + this.hash + ", previousBlockHash=" + this.previousBlockHash + ", epoch=" + this.epoch + ", shard=" + this.shard + ", accumulatedFees=" + this.accumulatedFees + ", developerFees=" + this.developerFees + ", status=" + this.status + ")";
}
}
public static ShardBlock.ShardBlockBuilder builder() {
return new ShardBlock.ShardBlockBuilder();
}
public Long getNonce() {
return this.nonce;
}
public Long getRound() {
return this.round;
}
public String getHash() {
return this.hash;
}
public String getPreviousBlockHash() {
return this.previousBlockHash;
}
public Long getEpoch() {
return this.epoch;
}
public Integer getShard() {
return this.shard;
}
public BigInteger getAccumulatedFees() {
return this.accumulatedFees;
}
public BigInteger getDeveloperFees() {
return this.developerFees;
}
public String getStatus() {
return this.status;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ShardBlock)) return false;
final ShardBlock other = (ShardBlock) o;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$round = this.getRound();
final Object other$round = other.getRound();
if (this$round == null ? other$round != null : !this$round.equals(other$round)) return false;
final Object this$epoch = this.getEpoch();
final Object other$epoch = other.getEpoch();
if (this$epoch == null ? other$epoch != null : !this$epoch.equals(other$epoch)) return false;
final Object this$shard = this.getShard();
final Object other$shard = other.getShard();
if (this$shard == null ? other$shard != null : !this$shard.equals(other$shard)) return false;
final Object this$hash = this.getHash();
final Object other$hash = other.getHash();
if (this$hash == null ? other$hash != null : !this$hash.equals(other$hash)) return false;
final Object this$previousBlockHash = this.getPreviousBlockHash();
final Object other$previousBlockHash = other.getPreviousBlockHash();
if (this$previousBlockHash == null ? other$previousBlockHash != null : !this$previousBlockHash.equals(other$previousBlockHash)) return false;
final Object this$accumulatedFees = this.getAccumulatedFees();
final Object other$accumulatedFees = other.getAccumulatedFees();
if (this$accumulatedFees == null ? other$accumulatedFees != null : !this$accumulatedFees.equals(other$accumulatedFees)) return false;
final Object this$developerFees = this.getDeveloperFees();
final Object other$developerFees = other.getDeveloperFees();
if (this$developerFees == null ? other$developerFees != null : !this$developerFees.equals(other$developerFees)) return false;
final Object this$status = this.getStatus();
final Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $round = this.getRound();
result = result * PRIME + ($round == null ? 43 : $round.hashCode());
final Object $epoch = this.getEpoch();
result = result * PRIME + ($epoch == null ? 43 : $epoch.hashCode());
final Object $shard = this.getShard();
result = result * PRIME + ($shard == null ? 43 : $shard.hashCode());
final Object $hash = this.getHash();
result = result * PRIME + ($hash == null ? 43 : $hash.hashCode());
final Object $previousBlockHash = this.getPreviousBlockHash();
result = result * PRIME + ($previousBlockHash == null ? 43 : $previousBlockHash.hashCode());
final Object $accumulatedFees = this.getAccumulatedFees();
result = result * PRIME + ($accumulatedFees == null ? 43 : $accumulatedFees.hashCode());
final Object $developerFees = this.getDeveloperFees();
result = result * PRIME + ($developerFees == null ? 43 : $developerFees.hashCode());
final Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
@Override
public String toString() {
return "ShardBlock(nonce=" + this.getNonce() + ", round=" + this.getRound() + ", hash=" + this.getHash() + ", previousBlockHash=" + this.getPreviousBlockHash() + ", epoch=" + this.getEpoch() + ", shard=" + this.getShard() + ", accumulatedFees=" + this.getAccumulatedFees() + ", developerFees=" + this.getDeveloperFees() + ", status=" + this.getStatus() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy