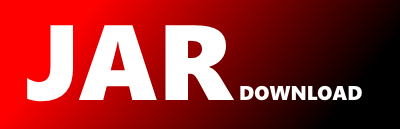
software.crldev.elrondspringbootstarterreactive.api.model.ShardFromSimulatedTransaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* part of API response when simulating transaction execution
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = ShardFromSimulatedTransaction.ShardFromSimulatedTransactionBuilder.class)
public final class ShardFromSimulatedTransaction {
@JsonProperty("status")
private final String status;
@JsonProperty("failReason")
private final String failReason;
@JsonProperty("hash")
private final String hash;
ShardFromSimulatedTransaction(final String status, final String failReason, final String hash) {
this.status = status;
this.failReason = failReason;
this.hash = hash;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class ShardFromSimulatedTransactionBuilder {
private String status;
private String failReason;
private String hash;
ShardFromSimulatedTransactionBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("status")
public ShardFromSimulatedTransaction.ShardFromSimulatedTransactionBuilder status(final String status) {
this.status = status;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("failReason")
public ShardFromSimulatedTransaction.ShardFromSimulatedTransactionBuilder failReason(final String failReason) {
this.failReason = failReason;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("hash")
public ShardFromSimulatedTransaction.ShardFromSimulatedTransactionBuilder hash(final String hash) {
this.hash = hash;
return this;
}
public ShardFromSimulatedTransaction build() {
return new ShardFromSimulatedTransaction(this.status, this.failReason, this.hash);
}
@Override
public String toString() {
return "ShardFromSimulatedTransaction.ShardFromSimulatedTransactionBuilder(status=" + this.status + ", failReason=" + this.failReason + ", hash=" + this.hash + ")";
}
}
public static ShardFromSimulatedTransaction.ShardFromSimulatedTransactionBuilder builder() {
return new ShardFromSimulatedTransaction.ShardFromSimulatedTransactionBuilder();
}
public String getStatus() {
return this.status;
}
public String getFailReason() {
return this.failReason;
}
public String getHash() {
return this.hash;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ShardFromSimulatedTransaction)) return false;
final ShardFromSimulatedTransaction other = (ShardFromSimulatedTransaction) o;
final Object this$status = this.getStatus();
final Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final Object this$failReason = this.getFailReason();
final Object other$failReason = other.getFailReason();
if (this$failReason == null ? other$failReason != null : !this$failReason.equals(other$failReason)) return false;
final Object this$hash = this.getHash();
final Object other$hash = other.getHash();
if (this$hash == null ? other$hash != null : !this$hash.equals(other$hash)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final Object $failReason = this.getFailReason();
result = result * PRIME + ($failReason == null ? 43 : $failReason.hashCode());
final Object $hash = this.getHash();
result = result * PRIME + ($hash == null ? 43 : $hash.hashCode());
return result;
}
@Override
public String toString() {
return "ShardFromSimulatedTransaction(status=" + this.getStatus() + ", failReason=" + this.getFailReason() + ", hash=" + this.getHash() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy