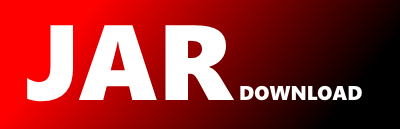
software.crldev.elrondspringbootstarterreactive.api.model.ShardStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* API response when querying for shard status
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = ShardStatus.ShardStatusBuilder.class)
public final class ShardStatus {
@JsonProperty("erd_current_round")
private final long currentRound;
@JsonProperty("erd_epoch_number")
private final long epochNumber;
@JsonProperty("erd_highest_final_nonce")
private final long highestFinalNonce;
@JsonProperty("erd_nonce")
private final long nonce;
@JsonProperty("erd_nonce_at_epoch_start")
private final long nonceAtEpochStart;
@JsonProperty("erd_nonces_passed_in_current_epoch")
private final long noncesPassedInCurrentEpoch;
@JsonProperty("erd_round_at_epoch_start")
private final long roundAtEpochStart;
@JsonProperty("erd_rounds_passed_in_current_epoch")
private final long roundsPassedInCurrentEpoch;
@JsonProperty("erd_rounds_per_epoch")
private final long roundsPerEpoch;
ShardStatus(final long currentRound, final long epochNumber, final long highestFinalNonce, final long nonce, final long nonceAtEpochStart, final long noncesPassedInCurrentEpoch, final long roundAtEpochStart, final long roundsPassedInCurrentEpoch, final long roundsPerEpoch) {
this.currentRound = currentRound;
this.epochNumber = epochNumber;
this.highestFinalNonce = highestFinalNonce;
this.nonce = nonce;
this.nonceAtEpochStart = nonceAtEpochStart;
this.noncesPassedInCurrentEpoch = noncesPassedInCurrentEpoch;
this.roundAtEpochStart = roundAtEpochStart;
this.roundsPassedInCurrentEpoch = roundsPassedInCurrentEpoch;
this.roundsPerEpoch = roundsPerEpoch;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class ShardStatusBuilder {
private long currentRound;
private long epochNumber;
private long highestFinalNonce;
private long nonce;
private long nonceAtEpochStart;
private long noncesPassedInCurrentEpoch;
private long roundAtEpochStart;
private long roundsPassedInCurrentEpoch;
private long roundsPerEpoch;
ShardStatusBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_current_round")
public ShardStatus.ShardStatusBuilder currentRound(final long currentRound) {
this.currentRound = currentRound;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_epoch_number")
public ShardStatus.ShardStatusBuilder epochNumber(final long epochNumber) {
this.epochNumber = epochNumber;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_highest_final_nonce")
public ShardStatus.ShardStatusBuilder highestFinalNonce(final long highestFinalNonce) {
this.highestFinalNonce = highestFinalNonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_nonce")
public ShardStatus.ShardStatusBuilder nonce(final long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_nonce_at_epoch_start")
public ShardStatus.ShardStatusBuilder nonceAtEpochStart(final long nonceAtEpochStart) {
this.nonceAtEpochStart = nonceAtEpochStart;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_nonces_passed_in_current_epoch")
public ShardStatus.ShardStatusBuilder noncesPassedInCurrentEpoch(final long noncesPassedInCurrentEpoch) {
this.noncesPassedInCurrentEpoch = noncesPassedInCurrentEpoch;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_round_at_epoch_start")
public ShardStatus.ShardStatusBuilder roundAtEpochStart(final long roundAtEpochStart) {
this.roundAtEpochStart = roundAtEpochStart;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_rounds_passed_in_current_epoch")
public ShardStatus.ShardStatusBuilder roundsPassedInCurrentEpoch(final long roundsPassedInCurrentEpoch) {
this.roundsPassedInCurrentEpoch = roundsPassedInCurrentEpoch;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("erd_rounds_per_epoch")
public ShardStatus.ShardStatusBuilder roundsPerEpoch(final long roundsPerEpoch) {
this.roundsPerEpoch = roundsPerEpoch;
return this;
}
public ShardStatus build() {
return new ShardStatus(this.currentRound, this.epochNumber, this.highestFinalNonce, this.nonce, this.nonceAtEpochStart, this.noncesPassedInCurrentEpoch, this.roundAtEpochStart, this.roundsPassedInCurrentEpoch, this.roundsPerEpoch);
}
@Override
public String toString() {
return "ShardStatus.ShardStatusBuilder(currentRound=" + this.currentRound + ", epochNumber=" + this.epochNumber + ", highestFinalNonce=" + this.highestFinalNonce + ", nonce=" + this.nonce + ", nonceAtEpochStart=" + this.nonceAtEpochStart + ", noncesPassedInCurrentEpoch=" + this.noncesPassedInCurrentEpoch + ", roundAtEpochStart=" + this.roundAtEpochStart + ", roundsPassedInCurrentEpoch=" + this.roundsPassedInCurrentEpoch + ", roundsPerEpoch=" + this.roundsPerEpoch + ")";
}
}
public static ShardStatus.ShardStatusBuilder builder() {
return new ShardStatus.ShardStatusBuilder();
}
public long getCurrentRound() {
return this.currentRound;
}
public long getEpochNumber() {
return this.epochNumber;
}
public long getHighestFinalNonce() {
return this.highestFinalNonce;
}
public long getNonce() {
return this.nonce;
}
public long getNonceAtEpochStart() {
return this.nonceAtEpochStart;
}
public long getNoncesPassedInCurrentEpoch() {
return this.noncesPassedInCurrentEpoch;
}
public long getRoundAtEpochStart() {
return this.roundAtEpochStart;
}
public long getRoundsPassedInCurrentEpoch() {
return this.roundsPassedInCurrentEpoch;
}
public long getRoundsPerEpoch() {
return this.roundsPerEpoch;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ShardStatus)) return false;
final ShardStatus other = (ShardStatus) o;
if (this.getCurrentRound() != other.getCurrentRound()) return false;
if (this.getEpochNumber() != other.getEpochNumber()) return false;
if (this.getHighestFinalNonce() != other.getHighestFinalNonce()) return false;
if (this.getNonce() != other.getNonce()) return false;
if (this.getNonceAtEpochStart() != other.getNonceAtEpochStart()) return false;
if (this.getNoncesPassedInCurrentEpoch() != other.getNoncesPassedInCurrentEpoch()) return false;
if (this.getRoundAtEpochStart() != other.getRoundAtEpochStart()) return false;
if (this.getRoundsPassedInCurrentEpoch() != other.getRoundsPassedInCurrentEpoch()) return false;
if (this.getRoundsPerEpoch() != other.getRoundsPerEpoch()) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final long $currentRound = this.getCurrentRound();
result = result * PRIME + (int) ($currentRound >>> 32 ^ $currentRound);
final long $epochNumber = this.getEpochNumber();
result = result * PRIME + (int) ($epochNumber >>> 32 ^ $epochNumber);
final long $highestFinalNonce = this.getHighestFinalNonce();
result = result * PRIME + (int) ($highestFinalNonce >>> 32 ^ $highestFinalNonce);
final long $nonce = this.getNonce();
result = result * PRIME + (int) ($nonce >>> 32 ^ $nonce);
final long $nonceAtEpochStart = this.getNonceAtEpochStart();
result = result * PRIME + (int) ($nonceAtEpochStart >>> 32 ^ $nonceAtEpochStart);
final long $noncesPassedInCurrentEpoch = this.getNoncesPassedInCurrentEpoch();
result = result * PRIME + (int) ($noncesPassedInCurrentEpoch >>> 32 ^ $noncesPassedInCurrentEpoch);
final long $roundAtEpochStart = this.getRoundAtEpochStart();
result = result * PRIME + (int) ($roundAtEpochStart >>> 32 ^ $roundAtEpochStart);
final long $roundsPassedInCurrentEpoch = this.getRoundsPassedInCurrentEpoch();
result = result * PRIME + (int) ($roundsPassedInCurrentEpoch >>> 32 ^ $roundsPassedInCurrentEpoch);
final long $roundsPerEpoch = this.getRoundsPerEpoch();
result = result * PRIME + (int) ($roundsPerEpoch >>> 32 ^ $roundsPerEpoch);
return result;
}
@Override
public String toString() {
return "ShardStatus(currentRound=" + this.getCurrentRound() + ", epochNumber=" + this.getEpochNumber() + ", highestFinalNonce=" + this.getHighestFinalNonce() + ", nonce=" + this.getNonce() + ", nonceAtEpochStart=" + this.getNonceAtEpochStart() + ", noncesPassedInCurrentEpoch=" + this.getNoncesPassedInCurrentEpoch() + ", roundAtEpochStart=" + this.getRoundAtEpochStart() + ", roundsPassedInCurrentEpoch=" + this.getRoundsPassedInCurrentEpoch() + ", roundsPerEpoch=" + this.getRoundsPerEpoch() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy