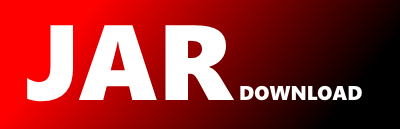
software.crldev.elrondspringbootstarterreactive.api.model.SmartContractResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.math.BigInteger;
/**
* part of API response when querying for transaction status
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = SmartContractResult.SmartContractResultBuilder.class)
public final class SmartContractResult {
@JsonProperty("hash")
private final String hash;
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("value")
private final BigInteger value;
@JsonProperty("receiver")
private final String receiver;
@JsonProperty("sender")
private final String sender;
@JsonProperty("data")
private final String data;
@JsonProperty("prevTxHash")
private final String previousTransactionHash;
@JsonProperty("originalTxHash")
private final String originalTransactionHash;
@JsonProperty("gasLimit")
private final BigInteger gasLimit;
@JsonProperty("gasPrice")
private final BigInteger gasPrice;
@JsonProperty("callType")
private final Integer callType;
SmartContractResult(final String hash, final Long nonce, final BigInteger value, final String receiver, final String sender, final String data, final String previousTransactionHash, final String originalTransactionHash, final BigInteger gasLimit, final BigInteger gasPrice, final Integer callType) {
this.hash = hash;
this.nonce = nonce;
this.value = value;
this.receiver = receiver;
this.sender = sender;
this.data = data;
this.previousTransactionHash = previousTransactionHash;
this.originalTransactionHash = originalTransactionHash;
this.gasLimit = gasLimit;
this.gasPrice = gasPrice;
this.callType = callType;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class SmartContractResultBuilder {
private String hash;
private Long nonce;
private BigInteger value;
private String receiver;
private String sender;
private String data;
private String previousTransactionHash;
private String originalTransactionHash;
private BigInteger gasLimit;
private BigInteger gasPrice;
private Integer callType;
SmartContractResultBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("hash")
public SmartContractResult.SmartContractResultBuilder hash(final String hash) {
this.hash = hash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
public SmartContractResult.SmartContractResultBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("value")
public SmartContractResult.SmartContractResultBuilder value(final BigInteger value) {
this.value = value;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("receiver")
public SmartContractResult.SmartContractResultBuilder receiver(final String receiver) {
this.receiver = receiver;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("sender")
public SmartContractResult.SmartContractResultBuilder sender(final String sender) {
this.sender = sender;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("data")
public SmartContractResult.SmartContractResultBuilder data(final String data) {
this.data = data;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("prevTxHash")
public SmartContractResult.SmartContractResultBuilder previousTransactionHash(final String previousTransactionHash) {
this.previousTransactionHash = previousTransactionHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("originalTxHash")
public SmartContractResult.SmartContractResultBuilder originalTransactionHash(final String originalTransactionHash) {
this.originalTransactionHash = originalTransactionHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasLimit")
public SmartContractResult.SmartContractResultBuilder gasLimit(final BigInteger gasLimit) {
this.gasLimit = gasLimit;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasPrice")
public SmartContractResult.SmartContractResultBuilder gasPrice(final BigInteger gasPrice) {
this.gasPrice = gasPrice;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("callType")
public SmartContractResult.SmartContractResultBuilder callType(final Integer callType) {
this.callType = callType;
return this;
}
public SmartContractResult build() {
return new SmartContractResult(this.hash, this.nonce, this.value, this.receiver, this.sender, this.data, this.previousTransactionHash, this.originalTransactionHash, this.gasLimit, this.gasPrice, this.callType);
}
@Override
public String toString() {
return "SmartContractResult.SmartContractResultBuilder(hash=" + this.hash + ", nonce=" + this.nonce + ", value=" + this.value + ", receiver=" + this.receiver + ", sender=" + this.sender + ", data=" + this.data + ", previousTransactionHash=" + this.previousTransactionHash + ", originalTransactionHash=" + this.originalTransactionHash + ", gasLimit=" + this.gasLimit + ", gasPrice=" + this.gasPrice + ", callType=" + this.callType + ")";
}
}
public static SmartContractResult.SmartContractResultBuilder builder() {
return new SmartContractResult.SmartContractResultBuilder();
}
public String getHash() {
return this.hash;
}
public Long getNonce() {
return this.nonce;
}
public BigInteger getValue() {
return this.value;
}
public String getReceiver() {
return this.receiver;
}
public String getSender() {
return this.sender;
}
public String getData() {
return this.data;
}
public String getPreviousTransactionHash() {
return this.previousTransactionHash;
}
public String getOriginalTransactionHash() {
return this.originalTransactionHash;
}
public BigInteger getGasLimit() {
return this.gasLimit;
}
public BigInteger getGasPrice() {
return this.gasPrice;
}
public Integer getCallType() {
return this.callType;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof SmartContractResult)) return false;
final SmartContractResult other = (SmartContractResult) o;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$callType = this.getCallType();
final Object other$callType = other.getCallType();
if (this$callType == null ? other$callType != null : !this$callType.equals(other$callType)) return false;
final Object this$hash = this.getHash();
final Object other$hash = other.getHash();
if (this$hash == null ? other$hash != null : !this$hash.equals(other$hash)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final Object this$receiver = this.getReceiver();
final Object other$receiver = other.getReceiver();
if (this$receiver == null ? other$receiver != null : !this$receiver.equals(other$receiver)) return false;
final Object this$sender = this.getSender();
final Object other$sender = other.getSender();
if (this$sender == null ? other$sender != null : !this$sender.equals(other$sender)) return false;
final Object this$data = this.getData();
final Object other$data = other.getData();
if (this$data == null ? other$data != null : !this$data.equals(other$data)) return false;
final Object this$previousTransactionHash = this.getPreviousTransactionHash();
final Object other$previousTransactionHash = other.getPreviousTransactionHash();
if (this$previousTransactionHash == null ? other$previousTransactionHash != null : !this$previousTransactionHash.equals(other$previousTransactionHash)) return false;
final Object this$originalTransactionHash = this.getOriginalTransactionHash();
final Object other$originalTransactionHash = other.getOriginalTransactionHash();
if (this$originalTransactionHash == null ? other$originalTransactionHash != null : !this$originalTransactionHash.equals(other$originalTransactionHash)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
final Object this$gasPrice = this.getGasPrice();
final Object other$gasPrice = other.getGasPrice();
if (this$gasPrice == null ? other$gasPrice != null : !this$gasPrice.equals(other$gasPrice)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $callType = this.getCallType();
result = result * PRIME + ($callType == null ? 43 : $callType.hashCode());
final Object $hash = this.getHash();
result = result * PRIME + ($hash == null ? 43 : $hash.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final Object $receiver = this.getReceiver();
result = result * PRIME + ($receiver == null ? 43 : $receiver.hashCode());
final Object $sender = this.getSender();
result = result * PRIME + ($sender == null ? 43 : $sender.hashCode());
final Object $data = this.getData();
result = result * PRIME + ($data == null ? 43 : $data.hashCode());
final Object $previousTransactionHash = this.getPreviousTransactionHash();
result = result * PRIME + ($previousTransactionHash == null ? 43 : $previousTransactionHash.hashCode());
final Object $originalTransactionHash = this.getOriginalTransactionHash();
result = result * PRIME + ($originalTransactionHash == null ? 43 : $originalTransactionHash.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
final Object $gasPrice = this.getGasPrice();
result = result * PRIME + ($gasPrice == null ? 43 : $gasPrice.hashCode());
return result;
}
@Override
public String toString() {
return "SmartContractResult(hash=" + this.getHash() + ", nonce=" + this.getNonce() + ", value=" + this.getValue() + ", receiver=" + this.getReceiver() + ", sender=" + this.getSender() + ", data=" + this.getData() + ", previousTransactionHash=" + this.getPreviousTransactionHash() + ", originalTransactionHash=" + this.getOriginalTransactionHash() + ", gasLimit=" + this.getGasLimit() + ", gasPrice=" + this.getGasPrice() + ", callType=" + this.getCallType() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy