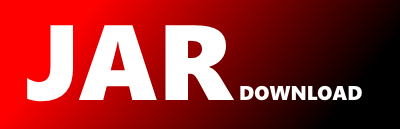
software.crldev.elrondspringbootstarterreactive.api.model.TransactionForAddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import software.crldev.elrondspringbootstarterreactive.domain.ApiModelToDomainConvertible;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.common.Nonce;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.TransactionStatus;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.*;
import java.math.BigInteger;
import java.util.List;
/**
* API response when querying for transactions by address
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = TransactionForAddress.TransactionForAddressBuilder.class)
public final class TransactionForAddress implements ApiModelToDomainConvertible {
@JsonProperty("hash")
private final String hash;
@JsonProperty("fee")
private final String fee;
@JsonProperty("miniBlockHash")
private final String miniBlockHash;
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("round")
private final Long round;
@JsonProperty("value")
private final BigInteger value;
@JsonProperty("receiver")
private final String receiver;
@JsonProperty("sender")
private final String sender;
@JsonProperty("receiverShard")
private final Long receiverShard;
@JsonProperty("senderShard")
private final Long senderShard;
@JsonProperty("gasPrice")
private final BigInteger gasPrice;
@JsonProperty("gasLimit")
private final BigInteger gasLimit;
@JsonProperty("gasUsed")
private final BigInteger gasUsed;
@JsonProperty("data")
private final String data;
@JsonProperty("signature")
private final String signature;
@JsonProperty("timestamp")
private final Long timestamp;
@JsonProperty("status")
private final String status;
@JsonProperty("searchOrder")
private final Integer searchOrder;
@JsonProperty("scResults")
private final List scResults;
@Override
public Transaction toDomainObject() {
var transaction = new Transaction();
transaction.setReceiver(Address.fromBech32(this.getReceiver()));
transaction.setSender(Address.fromBech32(this.getSender()));
transaction.setValue(Balance.fromNumber(this.getValue()));
transaction.setNonce(Nonce.fromLong(this.getNonce()));
transaction.setGasPrice(GasPrice.fromNumber(this.getGasPrice()));
transaction.setGasLimit(GasLimit.fromNumber(this.getGasLimit()));
transaction.setHash(Hash.fromString(this.getHash()));
transaction.setPayloadData(PayloadData.fromBase64Encoded(this.getData()));
transaction.applySignature(Signature.fromHex(this.getSignature()));
transaction.setStatus(TransactionStatus.fromString(this.getStatus()));
return transaction;
}
TransactionForAddress(final String hash, final String fee, final String miniBlockHash, final Long nonce, final Long round, final BigInteger value, final String receiver, final String sender, final Long receiverShard, final Long senderShard, final BigInteger gasPrice, final BigInteger gasLimit, final BigInteger gasUsed, final String data, final String signature, final Long timestamp, final String status, final Integer searchOrder, final List scResults) {
this.hash = hash;
this.fee = fee;
this.miniBlockHash = miniBlockHash;
this.nonce = nonce;
this.round = round;
this.value = value;
this.receiver = receiver;
this.sender = sender;
this.receiverShard = receiverShard;
this.senderShard = senderShard;
this.gasPrice = gasPrice;
this.gasLimit = gasLimit;
this.gasUsed = gasUsed;
this.data = data;
this.signature = signature;
this.timestamp = timestamp;
this.status = status;
this.searchOrder = searchOrder;
this.scResults = scResults;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class TransactionForAddressBuilder {
private String hash;
private String fee;
private String miniBlockHash;
private Long nonce;
private Long round;
private BigInteger value;
private String receiver;
private String sender;
private Long receiverShard;
private Long senderShard;
private BigInteger gasPrice;
private BigInteger gasLimit;
private BigInteger gasUsed;
private String data;
private String signature;
private Long timestamp;
private String status;
private Integer searchOrder;
private List scResults;
TransactionForAddressBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("hash")
public TransactionForAddress.TransactionForAddressBuilder hash(final String hash) {
this.hash = hash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("fee")
public TransactionForAddress.TransactionForAddressBuilder fee(final String fee) {
this.fee = fee;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("miniBlockHash")
public TransactionForAddress.TransactionForAddressBuilder miniBlockHash(final String miniBlockHash) {
this.miniBlockHash = miniBlockHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
public TransactionForAddress.TransactionForAddressBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("round")
public TransactionForAddress.TransactionForAddressBuilder round(final Long round) {
this.round = round;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("value")
public TransactionForAddress.TransactionForAddressBuilder value(final BigInteger value) {
this.value = value;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("receiver")
public TransactionForAddress.TransactionForAddressBuilder receiver(final String receiver) {
this.receiver = receiver;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("sender")
public TransactionForAddress.TransactionForAddressBuilder sender(final String sender) {
this.sender = sender;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("receiverShard")
public TransactionForAddress.TransactionForAddressBuilder receiverShard(final Long receiverShard) {
this.receiverShard = receiverShard;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("senderShard")
public TransactionForAddress.TransactionForAddressBuilder senderShard(final Long senderShard) {
this.senderShard = senderShard;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasPrice")
public TransactionForAddress.TransactionForAddressBuilder gasPrice(final BigInteger gasPrice) {
this.gasPrice = gasPrice;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasLimit")
public TransactionForAddress.TransactionForAddressBuilder gasLimit(final BigInteger gasLimit) {
this.gasLimit = gasLimit;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasUsed")
public TransactionForAddress.TransactionForAddressBuilder gasUsed(final BigInteger gasUsed) {
this.gasUsed = gasUsed;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("data")
public TransactionForAddress.TransactionForAddressBuilder data(final String data) {
this.data = data;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("signature")
public TransactionForAddress.TransactionForAddressBuilder signature(final String signature) {
this.signature = signature;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("timestamp")
public TransactionForAddress.TransactionForAddressBuilder timestamp(final Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("status")
public TransactionForAddress.TransactionForAddressBuilder status(final String status) {
this.status = status;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("searchOrder")
public TransactionForAddress.TransactionForAddressBuilder searchOrder(final Integer searchOrder) {
this.searchOrder = searchOrder;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("scResults")
public TransactionForAddress.TransactionForAddressBuilder scResults(final List scResults) {
this.scResults = scResults;
return this;
}
public TransactionForAddress build() {
return new TransactionForAddress(this.hash, this.fee, this.miniBlockHash, this.nonce, this.round, this.value, this.receiver, this.sender, this.receiverShard, this.senderShard, this.gasPrice, this.gasLimit, this.gasUsed, this.data, this.signature, this.timestamp, this.status, this.searchOrder, this.scResults);
}
@Override
public String toString() {
return "TransactionForAddress.TransactionForAddressBuilder(hash=" + this.hash + ", fee=" + this.fee + ", miniBlockHash=" + this.miniBlockHash + ", nonce=" + this.nonce + ", round=" + this.round + ", value=" + this.value + ", receiver=" + this.receiver + ", sender=" + this.sender + ", receiverShard=" + this.receiverShard + ", senderShard=" + this.senderShard + ", gasPrice=" + this.gasPrice + ", gasLimit=" + this.gasLimit + ", gasUsed=" + this.gasUsed + ", data=" + this.data + ", signature=" + this.signature + ", timestamp=" + this.timestamp + ", status=" + this.status + ", searchOrder=" + this.searchOrder + ", scResults=" + this.scResults + ")";
}
}
public static TransactionForAddress.TransactionForAddressBuilder builder() {
return new TransactionForAddress.TransactionForAddressBuilder();
}
public String getHash() {
return this.hash;
}
public String getFee() {
return this.fee;
}
public String getMiniBlockHash() {
return this.miniBlockHash;
}
public Long getNonce() {
return this.nonce;
}
public Long getRound() {
return this.round;
}
public BigInteger getValue() {
return this.value;
}
public String getReceiver() {
return this.receiver;
}
public String getSender() {
return this.sender;
}
public Long getReceiverShard() {
return this.receiverShard;
}
public Long getSenderShard() {
return this.senderShard;
}
public BigInteger getGasPrice() {
return this.gasPrice;
}
public BigInteger getGasLimit() {
return this.gasLimit;
}
public BigInteger getGasUsed() {
return this.gasUsed;
}
public String getData() {
return this.data;
}
public String getSignature() {
return this.signature;
}
public Long getTimestamp() {
return this.timestamp;
}
public String getStatus() {
return this.status;
}
public Integer getSearchOrder() {
return this.searchOrder;
}
public List getScResults() {
return this.scResults;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof TransactionForAddress)) return false;
final TransactionForAddress other = (TransactionForAddress) o;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$round = this.getRound();
final Object other$round = other.getRound();
if (this$round == null ? other$round != null : !this$round.equals(other$round)) return false;
final Object this$receiverShard = this.getReceiverShard();
final Object other$receiverShard = other.getReceiverShard();
if (this$receiverShard == null ? other$receiverShard != null : !this$receiverShard.equals(other$receiverShard)) return false;
final Object this$senderShard = this.getSenderShard();
final Object other$senderShard = other.getSenderShard();
if (this$senderShard == null ? other$senderShard != null : !this$senderShard.equals(other$senderShard)) return false;
final Object this$timestamp = this.getTimestamp();
final Object other$timestamp = other.getTimestamp();
if (this$timestamp == null ? other$timestamp != null : !this$timestamp.equals(other$timestamp)) return false;
final Object this$searchOrder = this.getSearchOrder();
final Object other$searchOrder = other.getSearchOrder();
if (this$searchOrder == null ? other$searchOrder != null : !this$searchOrder.equals(other$searchOrder)) return false;
final Object this$hash = this.getHash();
final Object other$hash = other.getHash();
if (this$hash == null ? other$hash != null : !this$hash.equals(other$hash)) return false;
final Object this$fee = this.getFee();
final Object other$fee = other.getFee();
if (this$fee == null ? other$fee != null : !this$fee.equals(other$fee)) return false;
final Object this$miniBlockHash = this.getMiniBlockHash();
final Object other$miniBlockHash = other.getMiniBlockHash();
if (this$miniBlockHash == null ? other$miniBlockHash != null : !this$miniBlockHash.equals(other$miniBlockHash)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final Object this$receiver = this.getReceiver();
final Object other$receiver = other.getReceiver();
if (this$receiver == null ? other$receiver != null : !this$receiver.equals(other$receiver)) return false;
final Object this$sender = this.getSender();
final Object other$sender = other.getSender();
if (this$sender == null ? other$sender != null : !this$sender.equals(other$sender)) return false;
final Object this$gasPrice = this.getGasPrice();
final Object other$gasPrice = other.getGasPrice();
if (this$gasPrice == null ? other$gasPrice != null : !this$gasPrice.equals(other$gasPrice)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
final Object this$gasUsed = this.getGasUsed();
final Object other$gasUsed = other.getGasUsed();
if (this$gasUsed == null ? other$gasUsed != null : !this$gasUsed.equals(other$gasUsed)) return false;
final Object this$data = this.getData();
final Object other$data = other.getData();
if (this$data == null ? other$data != null : !this$data.equals(other$data)) return false;
final Object this$signature = this.getSignature();
final Object other$signature = other.getSignature();
if (this$signature == null ? other$signature != null : !this$signature.equals(other$signature)) return false;
final Object this$status = this.getStatus();
final Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final Object this$scResults = this.getScResults();
final Object other$scResults = other.getScResults();
if (this$scResults == null ? other$scResults != null : !this$scResults.equals(other$scResults)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $round = this.getRound();
result = result * PRIME + ($round == null ? 43 : $round.hashCode());
final Object $receiverShard = this.getReceiverShard();
result = result * PRIME + ($receiverShard == null ? 43 : $receiverShard.hashCode());
final Object $senderShard = this.getSenderShard();
result = result * PRIME + ($senderShard == null ? 43 : $senderShard.hashCode());
final Object $timestamp = this.getTimestamp();
result = result * PRIME + ($timestamp == null ? 43 : $timestamp.hashCode());
final Object $searchOrder = this.getSearchOrder();
result = result * PRIME + ($searchOrder == null ? 43 : $searchOrder.hashCode());
final Object $hash = this.getHash();
result = result * PRIME + ($hash == null ? 43 : $hash.hashCode());
final Object $fee = this.getFee();
result = result * PRIME + ($fee == null ? 43 : $fee.hashCode());
final Object $miniBlockHash = this.getMiniBlockHash();
result = result * PRIME + ($miniBlockHash == null ? 43 : $miniBlockHash.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final Object $receiver = this.getReceiver();
result = result * PRIME + ($receiver == null ? 43 : $receiver.hashCode());
final Object $sender = this.getSender();
result = result * PRIME + ($sender == null ? 43 : $sender.hashCode());
final Object $gasPrice = this.getGasPrice();
result = result * PRIME + ($gasPrice == null ? 43 : $gasPrice.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
final Object $gasUsed = this.getGasUsed();
result = result * PRIME + ($gasUsed == null ? 43 : $gasUsed.hashCode());
final Object $data = this.getData();
result = result * PRIME + ($data == null ? 43 : $data.hashCode());
final Object $signature = this.getSignature();
result = result * PRIME + ($signature == null ? 43 : $signature.hashCode());
final Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final Object $scResults = this.getScResults();
result = result * PRIME + ($scResults == null ? 43 : $scResults.hashCode());
return result;
}
@Override
public String toString() {
return "TransactionForAddress(hash=" + this.getHash() + ", fee=" + this.getFee() + ", miniBlockHash=" + this.getMiniBlockHash() + ", nonce=" + this.getNonce() + ", round=" + this.getRound() + ", value=" + this.getValue() + ", receiver=" + this.getReceiver() + ", sender=" + this.getSender() + ", receiverShard=" + this.getReceiverShard() + ", senderShard=" + this.getSenderShard() + ", gasPrice=" + this.getGasPrice() + ", gasLimit=" + this.getGasLimit() + ", gasUsed=" + this.getGasUsed() + ", data=" + this.getData() + ", signature=" + this.getSignature() + ", timestamp=" + this.getTimestamp() + ", status=" + this.getStatus() + ", searchOrder=" + this.getSearchOrder() + ", scResults=" + this.getScResults() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy