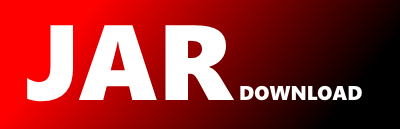
software.crldev.elrondspringbootstarterreactive.api.model.TransactionOnNetwork Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import software.crldev.elrondspringbootstarterreactive.domain.ApiModelToDomainConvertible;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.common.Nonce;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.*;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.TransactionStatus;
import java.math.BigInteger;
import java.util.List;
/**
* API response when querying for a transaction by hash
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = TransactionOnNetwork.TransactionOnNetworkBuilder.class)
public final class TransactionOnNetwork implements ApiModelToDomainConvertible {
@JsonProperty("type")
private final String type;
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("round")
private final Long round;
@JsonProperty("epoch")
private final Long epoch;
@JsonProperty("value")
private final BigInteger value;
@JsonProperty("receiver")
private final String receiver;
@JsonProperty("sender")
private final String sender;
@JsonProperty("gasPrice")
private final BigInteger gasPrice;
@JsonProperty("gasLimit")
private final BigInteger gasLimit;
@JsonProperty("data")
private final String data;
@JsonProperty("signature")
private final String signature;
@JsonProperty("sourceShard")
private final Long sourceShard;
@JsonProperty("destinationShard")
private final Long destinationShard;
@JsonProperty("blockNonce")
private final Integer blockNonce;
@JsonProperty("blockHash")
private final String blockHash;
@JsonProperty("notarizedAtSourceInMetaNonce")
private final Long notarizedAtSourceInMetaNonce;
@JsonProperty("NotarizedAtSourceInMetaHash")
private final String notarizedAtSourceInMetaHash;
@JsonProperty("notarizedAtDestinationInMetaNonce")
private final Long notarizedAtDestinationInMetaNonce;
@JsonProperty("notarizedAtDestinationInMetaHash")
private final String notarizedAtDestinationInMetaHash;
@JsonProperty("miniblockType")
private final String miniblockType;
@JsonProperty("miniblockHash")
private final String miniblockHash;
@JsonProperty("timestamp")
private final Long timestamp;
@JsonProperty("status")
private final String status;
@JsonProperty("hyperblockNonce")
private final Long hyperblockNonce;
@JsonProperty("hyperblockHash")
private final String hyperblockHash;
@JsonProperty("receipt")
private final Receipt receipt;
@JsonProperty("smartContractResults")
private final List smartContractResults;
@JsonProperty("logs")
private final Logs logs;
@Override
public Transaction toDomainObject() {
var transaction = new Transaction();
transaction.setReceiver(Address.fromBech32(this.getReceiver()));
transaction.setSender(Address.fromBech32(this.getSender()));
transaction.setValue(Balance.fromNumber(this.getValue()));
transaction.setNonce(Nonce.fromLong(this.getNonce()));
transaction.setGasPrice(GasPrice.fromNumber(this.getGasPrice()));
transaction.setGasLimit(GasLimit.fromNumber(this.getGasLimit()));
transaction.setHash(Hash.fromString(this.getBlockHash()));
transaction.setPayloadData(PayloadData.fromBase64Encoded(this.getData()));
transaction.applySignature(Signature.fromHex(this.getSignature()));
transaction.setStatus(TransactionStatus.fromString(this.getStatus()));
return transaction;
}
@JsonDeserialize(builder = Logs.LogsBuilder.class)
private static class Logs {
@JsonProperty("address")
String address;
@JsonProperty("events")
List events;
Logs(final String address, final List events) {
this.address = address;
this.events = events;
}
public static class LogsBuilder {
private String address;
private List events;
LogsBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("address")
public TransactionOnNetwork.Logs.LogsBuilder address(final String address) {
this.address = address;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("events")
public TransactionOnNetwork.Logs.LogsBuilder events(final List events) {
this.events = events;
return this;
}
public TransactionOnNetwork.Logs build() {
return new TransactionOnNetwork.Logs(this.address, this.events);
}
@Override
public String toString() {
return "TransactionOnNetwork.Logs.LogsBuilder(address=" + this.address + ", events=" + this.events + ")";
}
}
public static TransactionOnNetwork.Logs.LogsBuilder builder() {
return new TransactionOnNetwork.Logs.LogsBuilder();
}
}
@JsonDeserialize(builder = Event.EventBuilder.class)
private static class Event {
@JsonProperty("address")
String address;
@JsonProperty("identifier")
String identifier;
@JsonProperty("topics")
List topics;
Event(final String address, final String identifier, final List topics) {
this.address = address;
this.identifier = identifier;
this.topics = topics;
}
public static class EventBuilder {
private String address;
private String identifier;
private List topics;
EventBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("address")
public TransactionOnNetwork.Event.EventBuilder address(final String address) {
this.address = address;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("identifier")
public TransactionOnNetwork.Event.EventBuilder identifier(final String identifier) {
this.identifier = identifier;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("topics")
public TransactionOnNetwork.Event.EventBuilder topics(final List topics) {
this.topics = topics;
return this;
}
public TransactionOnNetwork.Event build() {
return new TransactionOnNetwork.Event(this.address, this.identifier, this.topics);
}
@Override
public String toString() {
return "TransactionOnNetwork.Event.EventBuilder(address=" + this.address + ", identifier=" + this.identifier + ", topics=" + this.topics + ")";
}
}
public static TransactionOnNetwork.Event.EventBuilder builder() {
return new TransactionOnNetwork.Event.EventBuilder();
}
}
TransactionOnNetwork(final String type, final Long nonce, final Long round, final Long epoch, final BigInteger value, final String receiver, final String sender, final BigInteger gasPrice, final BigInteger gasLimit, final String data, final String signature, final Long sourceShard, final Long destinationShard, final Integer blockNonce, final String blockHash, final Long notarizedAtSourceInMetaNonce, final String notarizedAtSourceInMetaHash, final Long notarizedAtDestinationInMetaNonce, final String notarizedAtDestinationInMetaHash, final String miniblockType, final String miniblockHash, final Long timestamp, final String status, final Long hyperblockNonce, final String hyperblockHash, final Receipt receipt, final List smartContractResults, final Logs logs) {
this.type = type;
this.nonce = nonce;
this.round = round;
this.epoch = epoch;
this.value = value;
this.receiver = receiver;
this.sender = sender;
this.gasPrice = gasPrice;
this.gasLimit = gasLimit;
this.data = data;
this.signature = signature;
this.sourceShard = sourceShard;
this.destinationShard = destinationShard;
this.blockNonce = blockNonce;
this.blockHash = blockHash;
this.notarizedAtSourceInMetaNonce = notarizedAtSourceInMetaNonce;
this.notarizedAtSourceInMetaHash = notarizedAtSourceInMetaHash;
this.notarizedAtDestinationInMetaNonce = notarizedAtDestinationInMetaNonce;
this.notarizedAtDestinationInMetaHash = notarizedAtDestinationInMetaHash;
this.miniblockType = miniblockType;
this.miniblockHash = miniblockHash;
this.timestamp = timestamp;
this.status = status;
this.hyperblockNonce = hyperblockNonce;
this.hyperblockHash = hyperblockHash;
this.receipt = receipt;
this.smartContractResults = smartContractResults;
this.logs = logs;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class TransactionOnNetworkBuilder {
private String type;
private Long nonce;
private Long round;
private Long epoch;
private BigInteger value;
private String receiver;
private String sender;
private BigInteger gasPrice;
private BigInteger gasLimit;
private String data;
private String signature;
private Long sourceShard;
private Long destinationShard;
private Integer blockNonce;
private String blockHash;
private Long notarizedAtSourceInMetaNonce;
private String notarizedAtSourceInMetaHash;
private Long notarizedAtDestinationInMetaNonce;
private String notarizedAtDestinationInMetaHash;
private String miniblockType;
private String miniblockHash;
private Long timestamp;
private String status;
private Long hyperblockNonce;
private String hyperblockHash;
private Receipt receipt;
private List smartContractResults;
private Logs logs;
TransactionOnNetworkBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("type")
public TransactionOnNetwork.TransactionOnNetworkBuilder type(final String type) {
this.type = type;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
public TransactionOnNetwork.TransactionOnNetworkBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("round")
public TransactionOnNetwork.TransactionOnNetworkBuilder round(final Long round) {
this.round = round;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("epoch")
public TransactionOnNetwork.TransactionOnNetworkBuilder epoch(final Long epoch) {
this.epoch = epoch;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("value")
public TransactionOnNetwork.TransactionOnNetworkBuilder value(final BigInteger value) {
this.value = value;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("receiver")
public TransactionOnNetwork.TransactionOnNetworkBuilder receiver(final String receiver) {
this.receiver = receiver;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("sender")
public TransactionOnNetwork.TransactionOnNetworkBuilder sender(final String sender) {
this.sender = sender;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasPrice")
public TransactionOnNetwork.TransactionOnNetworkBuilder gasPrice(final BigInteger gasPrice) {
this.gasPrice = gasPrice;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasLimit")
public TransactionOnNetwork.TransactionOnNetworkBuilder gasLimit(final BigInteger gasLimit) {
this.gasLimit = gasLimit;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("data")
public TransactionOnNetwork.TransactionOnNetworkBuilder data(final String data) {
this.data = data;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("signature")
public TransactionOnNetwork.TransactionOnNetworkBuilder signature(final String signature) {
this.signature = signature;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("sourceShard")
public TransactionOnNetwork.TransactionOnNetworkBuilder sourceShard(final Long sourceShard) {
this.sourceShard = sourceShard;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("destinationShard")
public TransactionOnNetwork.TransactionOnNetworkBuilder destinationShard(final Long destinationShard) {
this.destinationShard = destinationShard;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("blockNonce")
public TransactionOnNetwork.TransactionOnNetworkBuilder blockNonce(final Integer blockNonce) {
this.blockNonce = blockNonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("blockHash")
public TransactionOnNetwork.TransactionOnNetworkBuilder blockHash(final String blockHash) {
this.blockHash = blockHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("notarizedAtSourceInMetaNonce")
public TransactionOnNetwork.TransactionOnNetworkBuilder notarizedAtSourceInMetaNonce(final Long notarizedAtSourceInMetaNonce) {
this.notarizedAtSourceInMetaNonce = notarizedAtSourceInMetaNonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("NotarizedAtSourceInMetaHash")
public TransactionOnNetwork.TransactionOnNetworkBuilder notarizedAtSourceInMetaHash(final String notarizedAtSourceInMetaHash) {
this.notarizedAtSourceInMetaHash = notarizedAtSourceInMetaHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("notarizedAtDestinationInMetaNonce")
public TransactionOnNetwork.TransactionOnNetworkBuilder notarizedAtDestinationInMetaNonce(final Long notarizedAtDestinationInMetaNonce) {
this.notarizedAtDestinationInMetaNonce = notarizedAtDestinationInMetaNonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("notarizedAtDestinationInMetaHash")
public TransactionOnNetwork.TransactionOnNetworkBuilder notarizedAtDestinationInMetaHash(final String notarizedAtDestinationInMetaHash) {
this.notarizedAtDestinationInMetaHash = notarizedAtDestinationInMetaHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("miniblockType")
public TransactionOnNetwork.TransactionOnNetworkBuilder miniblockType(final String miniblockType) {
this.miniblockType = miniblockType;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("miniblockHash")
public TransactionOnNetwork.TransactionOnNetworkBuilder miniblockHash(final String miniblockHash) {
this.miniblockHash = miniblockHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("timestamp")
public TransactionOnNetwork.TransactionOnNetworkBuilder timestamp(final Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("status")
public TransactionOnNetwork.TransactionOnNetworkBuilder status(final String status) {
this.status = status;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("hyperblockNonce")
public TransactionOnNetwork.TransactionOnNetworkBuilder hyperblockNonce(final Long hyperblockNonce) {
this.hyperblockNonce = hyperblockNonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("hyperblockHash")
public TransactionOnNetwork.TransactionOnNetworkBuilder hyperblockHash(final String hyperblockHash) {
this.hyperblockHash = hyperblockHash;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("receipt")
public TransactionOnNetwork.TransactionOnNetworkBuilder receipt(final Receipt receipt) {
this.receipt = receipt;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("smartContractResults")
public TransactionOnNetwork.TransactionOnNetworkBuilder smartContractResults(final List smartContractResults) {
this.smartContractResults = smartContractResults;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("logs")
public TransactionOnNetwork.TransactionOnNetworkBuilder logs(final Logs logs) {
this.logs = logs;
return this;
}
public TransactionOnNetwork build() {
return new TransactionOnNetwork(this.type, this.nonce, this.round, this.epoch, this.value, this.receiver, this.sender, this.gasPrice, this.gasLimit, this.data, this.signature, this.sourceShard, this.destinationShard, this.blockNonce, this.blockHash, this.notarizedAtSourceInMetaNonce, this.notarizedAtSourceInMetaHash, this.notarizedAtDestinationInMetaNonce, this.notarizedAtDestinationInMetaHash, this.miniblockType, this.miniblockHash, this.timestamp, this.status, this.hyperblockNonce, this.hyperblockHash, this.receipt, this.smartContractResults, this.logs);
}
@Override
public String toString() {
return "TransactionOnNetwork.TransactionOnNetworkBuilder(type=" + this.type + ", nonce=" + this.nonce + ", round=" + this.round + ", epoch=" + this.epoch + ", value=" + this.value + ", receiver=" + this.receiver + ", sender=" + this.sender + ", gasPrice=" + this.gasPrice + ", gasLimit=" + this.gasLimit + ", data=" + this.data + ", signature=" + this.signature + ", sourceShard=" + this.sourceShard + ", destinationShard=" + this.destinationShard + ", blockNonce=" + this.blockNonce + ", blockHash=" + this.blockHash + ", notarizedAtSourceInMetaNonce=" + this.notarizedAtSourceInMetaNonce + ", notarizedAtSourceInMetaHash=" + this.notarizedAtSourceInMetaHash + ", notarizedAtDestinationInMetaNonce=" + this.notarizedAtDestinationInMetaNonce + ", notarizedAtDestinationInMetaHash=" + this.notarizedAtDestinationInMetaHash + ", miniblockType=" + this.miniblockType + ", miniblockHash=" + this.miniblockHash + ", timestamp=" + this.timestamp + ", status=" + this.status + ", hyperblockNonce=" + this.hyperblockNonce + ", hyperblockHash=" + this.hyperblockHash + ", receipt=" + this.receipt + ", smartContractResults=" + this.smartContractResults + ", logs=" + this.logs + ")";
}
}
public static TransactionOnNetwork.TransactionOnNetworkBuilder builder() {
return new TransactionOnNetwork.TransactionOnNetworkBuilder();
}
public String getType() {
return this.type;
}
public Long getNonce() {
return this.nonce;
}
public Long getRound() {
return this.round;
}
public Long getEpoch() {
return this.epoch;
}
public BigInteger getValue() {
return this.value;
}
public String getReceiver() {
return this.receiver;
}
public String getSender() {
return this.sender;
}
public BigInteger getGasPrice() {
return this.gasPrice;
}
public BigInteger getGasLimit() {
return this.gasLimit;
}
public String getData() {
return this.data;
}
public String getSignature() {
return this.signature;
}
public Long getSourceShard() {
return this.sourceShard;
}
public Long getDestinationShard() {
return this.destinationShard;
}
public Integer getBlockNonce() {
return this.blockNonce;
}
public String getBlockHash() {
return this.blockHash;
}
public Long getNotarizedAtSourceInMetaNonce() {
return this.notarizedAtSourceInMetaNonce;
}
public String getNotarizedAtSourceInMetaHash() {
return this.notarizedAtSourceInMetaHash;
}
public Long getNotarizedAtDestinationInMetaNonce() {
return this.notarizedAtDestinationInMetaNonce;
}
public String getNotarizedAtDestinationInMetaHash() {
return this.notarizedAtDestinationInMetaHash;
}
public String getMiniblockType() {
return this.miniblockType;
}
public String getMiniblockHash() {
return this.miniblockHash;
}
public Long getTimestamp() {
return this.timestamp;
}
public String getStatus() {
return this.status;
}
public Long getHyperblockNonce() {
return this.hyperblockNonce;
}
public String getHyperblockHash() {
return this.hyperblockHash;
}
public Receipt getReceipt() {
return this.receipt;
}
public List getSmartContractResults() {
return this.smartContractResults;
}
public Logs getLogs() {
return this.logs;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof TransactionOnNetwork)) return false;
final TransactionOnNetwork other = (TransactionOnNetwork) o;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$round = this.getRound();
final Object other$round = other.getRound();
if (this$round == null ? other$round != null : !this$round.equals(other$round)) return false;
final Object this$epoch = this.getEpoch();
final Object other$epoch = other.getEpoch();
if (this$epoch == null ? other$epoch != null : !this$epoch.equals(other$epoch)) return false;
final Object this$sourceShard = this.getSourceShard();
final Object other$sourceShard = other.getSourceShard();
if (this$sourceShard == null ? other$sourceShard != null : !this$sourceShard.equals(other$sourceShard)) return false;
final Object this$destinationShard = this.getDestinationShard();
final Object other$destinationShard = other.getDestinationShard();
if (this$destinationShard == null ? other$destinationShard != null : !this$destinationShard.equals(other$destinationShard)) return false;
final Object this$blockNonce = this.getBlockNonce();
final Object other$blockNonce = other.getBlockNonce();
if (this$blockNonce == null ? other$blockNonce != null : !this$blockNonce.equals(other$blockNonce)) return false;
final Object this$notarizedAtSourceInMetaNonce = this.getNotarizedAtSourceInMetaNonce();
final Object other$notarizedAtSourceInMetaNonce = other.getNotarizedAtSourceInMetaNonce();
if (this$notarizedAtSourceInMetaNonce == null ? other$notarizedAtSourceInMetaNonce != null : !this$notarizedAtSourceInMetaNonce.equals(other$notarizedAtSourceInMetaNonce)) return false;
final Object this$notarizedAtDestinationInMetaNonce = this.getNotarizedAtDestinationInMetaNonce();
final Object other$notarizedAtDestinationInMetaNonce = other.getNotarizedAtDestinationInMetaNonce();
if (this$notarizedAtDestinationInMetaNonce == null ? other$notarizedAtDestinationInMetaNonce != null : !this$notarizedAtDestinationInMetaNonce.equals(other$notarizedAtDestinationInMetaNonce)) return false;
final Object this$timestamp = this.getTimestamp();
final Object other$timestamp = other.getTimestamp();
if (this$timestamp == null ? other$timestamp != null : !this$timestamp.equals(other$timestamp)) return false;
final Object this$hyperblockNonce = this.getHyperblockNonce();
final Object other$hyperblockNonce = other.getHyperblockNonce();
if (this$hyperblockNonce == null ? other$hyperblockNonce != null : !this$hyperblockNonce.equals(other$hyperblockNonce)) return false;
final Object this$type = this.getType();
final Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final Object this$receiver = this.getReceiver();
final Object other$receiver = other.getReceiver();
if (this$receiver == null ? other$receiver != null : !this$receiver.equals(other$receiver)) return false;
final Object this$sender = this.getSender();
final Object other$sender = other.getSender();
if (this$sender == null ? other$sender != null : !this$sender.equals(other$sender)) return false;
final Object this$gasPrice = this.getGasPrice();
final Object other$gasPrice = other.getGasPrice();
if (this$gasPrice == null ? other$gasPrice != null : !this$gasPrice.equals(other$gasPrice)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
final Object this$data = this.getData();
final Object other$data = other.getData();
if (this$data == null ? other$data != null : !this$data.equals(other$data)) return false;
final Object this$signature = this.getSignature();
final Object other$signature = other.getSignature();
if (this$signature == null ? other$signature != null : !this$signature.equals(other$signature)) return false;
final Object this$blockHash = this.getBlockHash();
final Object other$blockHash = other.getBlockHash();
if (this$blockHash == null ? other$blockHash != null : !this$blockHash.equals(other$blockHash)) return false;
final Object this$notarizedAtSourceInMetaHash = this.getNotarizedAtSourceInMetaHash();
final Object other$notarizedAtSourceInMetaHash = other.getNotarizedAtSourceInMetaHash();
if (this$notarizedAtSourceInMetaHash == null ? other$notarizedAtSourceInMetaHash != null : !this$notarizedAtSourceInMetaHash.equals(other$notarizedAtSourceInMetaHash)) return false;
final Object this$notarizedAtDestinationInMetaHash = this.getNotarizedAtDestinationInMetaHash();
final Object other$notarizedAtDestinationInMetaHash = other.getNotarizedAtDestinationInMetaHash();
if (this$notarizedAtDestinationInMetaHash == null ? other$notarizedAtDestinationInMetaHash != null : !this$notarizedAtDestinationInMetaHash.equals(other$notarizedAtDestinationInMetaHash)) return false;
final Object this$miniblockType = this.getMiniblockType();
final Object other$miniblockType = other.getMiniblockType();
if (this$miniblockType == null ? other$miniblockType != null : !this$miniblockType.equals(other$miniblockType)) return false;
final Object this$miniblockHash = this.getMiniblockHash();
final Object other$miniblockHash = other.getMiniblockHash();
if (this$miniblockHash == null ? other$miniblockHash != null : !this$miniblockHash.equals(other$miniblockHash)) return false;
final Object this$status = this.getStatus();
final Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final Object this$hyperblockHash = this.getHyperblockHash();
final Object other$hyperblockHash = other.getHyperblockHash();
if (this$hyperblockHash == null ? other$hyperblockHash != null : !this$hyperblockHash.equals(other$hyperblockHash)) return false;
final Object this$receipt = this.getReceipt();
final Object other$receipt = other.getReceipt();
if (this$receipt == null ? other$receipt != null : !this$receipt.equals(other$receipt)) return false;
final Object this$smartContractResults = this.getSmartContractResults();
final Object other$smartContractResults = other.getSmartContractResults();
if (this$smartContractResults == null ? other$smartContractResults != null : !this$smartContractResults.equals(other$smartContractResults)) return false;
final Object this$logs = this.getLogs();
final Object other$logs = other.getLogs();
if (this$logs == null ? other$logs != null : !this$logs.equals(other$logs)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $round = this.getRound();
result = result * PRIME + ($round == null ? 43 : $round.hashCode());
final Object $epoch = this.getEpoch();
result = result * PRIME + ($epoch == null ? 43 : $epoch.hashCode());
final Object $sourceShard = this.getSourceShard();
result = result * PRIME + ($sourceShard == null ? 43 : $sourceShard.hashCode());
final Object $destinationShard = this.getDestinationShard();
result = result * PRIME + ($destinationShard == null ? 43 : $destinationShard.hashCode());
final Object $blockNonce = this.getBlockNonce();
result = result * PRIME + ($blockNonce == null ? 43 : $blockNonce.hashCode());
final Object $notarizedAtSourceInMetaNonce = this.getNotarizedAtSourceInMetaNonce();
result = result * PRIME + ($notarizedAtSourceInMetaNonce == null ? 43 : $notarizedAtSourceInMetaNonce.hashCode());
final Object $notarizedAtDestinationInMetaNonce = this.getNotarizedAtDestinationInMetaNonce();
result = result * PRIME + ($notarizedAtDestinationInMetaNonce == null ? 43 : $notarizedAtDestinationInMetaNonce.hashCode());
final Object $timestamp = this.getTimestamp();
result = result * PRIME + ($timestamp == null ? 43 : $timestamp.hashCode());
final Object $hyperblockNonce = this.getHyperblockNonce();
result = result * PRIME + ($hyperblockNonce == null ? 43 : $hyperblockNonce.hashCode());
final Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final Object $receiver = this.getReceiver();
result = result * PRIME + ($receiver == null ? 43 : $receiver.hashCode());
final Object $sender = this.getSender();
result = result * PRIME + ($sender == null ? 43 : $sender.hashCode());
final Object $gasPrice = this.getGasPrice();
result = result * PRIME + ($gasPrice == null ? 43 : $gasPrice.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
final Object $data = this.getData();
result = result * PRIME + ($data == null ? 43 : $data.hashCode());
final Object $signature = this.getSignature();
result = result * PRIME + ($signature == null ? 43 : $signature.hashCode());
final Object $blockHash = this.getBlockHash();
result = result * PRIME + ($blockHash == null ? 43 : $blockHash.hashCode());
final Object $notarizedAtSourceInMetaHash = this.getNotarizedAtSourceInMetaHash();
result = result * PRIME + ($notarizedAtSourceInMetaHash == null ? 43 : $notarizedAtSourceInMetaHash.hashCode());
final Object $notarizedAtDestinationInMetaHash = this.getNotarizedAtDestinationInMetaHash();
result = result * PRIME + ($notarizedAtDestinationInMetaHash == null ? 43 : $notarizedAtDestinationInMetaHash.hashCode());
final Object $miniblockType = this.getMiniblockType();
result = result * PRIME + ($miniblockType == null ? 43 : $miniblockType.hashCode());
final Object $miniblockHash = this.getMiniblockHash();
result = result * PRIME + ($miniblockHash == null ? 43 : $miniblockHash.hashCode());
final Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final Object $hyperblockHash = this.getHyperblockHash();
result = result * PRIME + ($hyperblockHash == null ? 43 : $hyperblockHash.hashCode());
final Object $receipt = this.getReceipt();
result = result * PRIME + ($receipt == null ? 43 : $receipt.hashCode());
final Object $smartContractResults = this.getSmartContractResults();
result = result * PRIME + ($smartContractResults == null ? 43 : $smartContractResults.hashCode());
final Object $logs = this.getLogs();
result = result * PRIME + ($logs == null ? 43 : $logs.hashCode());
return result;
}
@Override
public String toString() {
return "TransactionOnNetwork(type=" + this.getType() + ", nonce=" + this.getNonce() + ", round=" + this.getRound() + ", epoch=" + this.getEpoch() + ", value=" + this.getValue() + ", receiver=" + this.getReceiver() + ", sender=" + this.getSender() + ", gasPrice=" + this.getGasPrice() + ", gasLimit=" + this.getGasLimit() + ", data=" + this.getData() + ", signature=" + this.getSignature() + ", sourceShard=" + this.getSourceShard() + ", destinationShard=" + this.getDestinationShard() + ", blockNonce=" + this.getBlockNonce() + ", blockHash=" + this.getBlockHash() + ", notarizedAtSourceInMetaNonce=" + this.getNotarizedAtSourceInMetaNonce() + ", notarizedAtSourceInMetaHash=" + this.getNotarizedAtSourceInMetaHash() + ", notarizedAtDestinationInMetaNonce=" + this.getNotarizedAtDestinationInMetaNonce() + ", notarizedAtDestinationInMetaHash=" + this.getNotarizedAtDestinationInMetaHash() + ", miniblockType=" + this.getMiniblockType() + ", miniblockHash=" + this.getMiniblockHash() + ", timestamp=" + this.getTimestamp() + ", status=" + this.getStatus() + ", hyperblockNonce=" + this.getHyperblockNonce() + ", hyperblockHash=" + this.getHyperblockHash() + ", receipt=" + this.getReceipt() + ", smartContractResults=" + this.getSmartContractResults() + ", logs=" + this.getLogs() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy