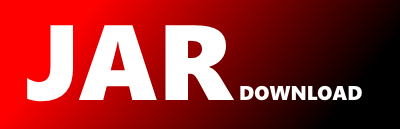
software.crldev.elrondspringbootstarterreactive.api.model.TransactionsSentResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Map;
/**
* API response sending multiple transactions for execution
*
* @author carlo_stanciu
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = TransactionsSentResult.TransactionsSentResultBuilder.class)
public final class TransactionsSentResult {
@JsonProperty("numOfSentTxs")
private final Integer numberOfSentTransactions;
@JsonProperty("txsHashes")
private final Map transactionsHashes;
TransactionsSentResult(final Integer numberOfSentTransactions, final Map transactionsHashes) {
this.numberOfSentTransactions = numberOfSentTransactions;
this.transactionsHashes = transactionsHashes;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class TransactionsSentResultBuilder {
private Integer numberOfSentTransactions;
private Map transactionsHashes;
TransactionsSentResultBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("numOfSentTxs")
public TransactionsSentResult.TransactionsSentResultBuilder numberOfSentTransactions(final Integer numberOfSentTransactions) {
this.numberOfSentTransactions = numberOfSentTransactions;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("txsHashes")
public TransactionsSentResult.TransactionsSentResultBuilder transactionsHashes(final Map transactionsHashes) {
this.transactionsHashes = transactionsHashes;
return this;
}
public TransactionsSentResult build() {
return new TransactionsSentResult(this.numberOfSentTransactions, this.transactionsHashes);
}
@Override
public String toString() {
return "TransactionsSentResult.TransactionsSentResultBuilder(numberOfSentTransactions=" + this.numberOfSentTransactions + ", transactionsHashes=" + this.transactionsHashes + ")";
}
}
public static TransactionsSentResult.TransactionsSentResultBuilder builder() {
return new TransactionsSentResult.TransactionsSentResultBuilder();
}
public Integer getNumberOfSentTransactions() {
return this.numberOfSentTransactions;
}
public Map getTransactionsHashes() {
return this.transactionsHashes;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof TransactionsSentResult)) return false;
final TransactionsSentResult other = (TransactionsSentResult) o;
final Object this$numberOfSentTransactions = this.getNumberOfSentTransactions();
final Object other$numberOfSentTransactions = other.getNumberOfSentTransactions();
if (this$numberOfSentTransactions == null ? other$numberOfSentTransactions != null : !this$numberOfSentTransactions.equals(other$numberOfSentTransactions)) return false;
final Object this$transactionsHashes = this.getTransactionsHashes();
final Object other$transactionsHashes = other.getTransactionsHashes();
if (this$transactionsHashes == null ? other$transactionsHashes != null : !this$transactionsHashes.equals(other$transactionsHashes)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $numberOfSentTransactions = this.getNumberOfSentTransactions();
result = result * PRIME + ($numberOfSentTransactions == null ? 43 : $numberOfSentTransactions.hashCode());
final Object $transactionsHashes = this.getTransactionsHashes();
result = result * PRIME + ($transactionsHashes == null ? 43 : $transactionsHashes.hashCode());
return result;
}
@Override
public String toString() {
return "TransactionsSentResult(numberOfSentTransactions=" + this.getNumberOfSentTransactions() + ", transactionsHashes=" + this.getTransactionsHashes() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy