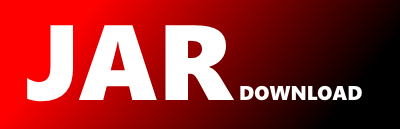
software.crldev.elrondspringbootstarterreactive.client.ErdProxyClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.client;
import software.crldev.elrondspringbootstarterreactive.api.ApiResponse;
import software.crldev.elrondspringbootstarterreactive.config.ErdClientConfig;
import software.crldev.elrondspringbootstarterreactive.config.JsonMapper;
import software.crldev.elrondspringbootstarterreactive.error.exception.EmptyPayloadException;
import software.crldev.elrondspringbootstarterreactive.error.exception.ProxyRequestException;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Mono;
import static java.util.Objects.nonNull;
public class ErdProxyClientImpl implements ErdProxyClient {
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(ErdProxyClientImpl.class);
private final WebClient erdClient;
public ErdProxyClientImpl(ErdClientConfig config) {
this.erdClient = config.getErdClientBuilder().build();
}
public Mono get(String uri, Class responseType) {
log.debug("[ProxyClient] executing GET {}", uri);
return processRequest(uri, null, responseType, HttpMethod.GET);
}
public Mono post(String uri, P payload, Class responseType) {
log.debug("[ProxyClient] executing POST {}", uri);
return processRequest(uri, payload, responseType, HttpMethod.POST);
}
private Mono processRequest(String uri, P payload, Class responseType, HttpMethod method) {
var requestBuilder = erdClient.method(method).uri(uri);
if (method == HttpMethod.POST) {
if (nonNull(payload)) {
requestBuilder.contentType(MediaType.APPLICATION_JSON).bodyValue(payload);
} else {
throw new EmptyPayloadException();
}
}
return requestBuilder.exchangeToMono(r -> r.bodyToMono(String.class)).map(r -> JsonMapper.deserializeApiResponse(r, responseType)).doOnSuccess(this::onSuccess).doOnError(this::onError).map(ApiResponse::getData);
}
private void onSuccess(ApiResponse response) {
response.throwIfError();
}
private void onError(Throwable t) {
var errorMessage = t.getMessage();
throw new ProxyRequestException(errorMessage);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy