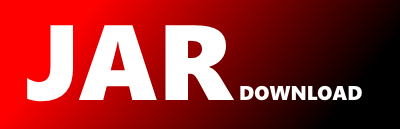
software.crldev.elrondspringbootstarterreactive.domain.account.Account Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.account;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.common.Nonce;
/**
* Domain object for Account representation
*
* @author carlo_stanciu
*/
public class Account {
private Address address = Address.zero();
private Nonce nonce = Nonce.zero();
private Balance balance = Balance.zero();
private String code;
private String username;
public Account() {
}
public Address getAddress() {
return this.address;
}
public Nonce getNonce() {
return this.nonce;
}
public Balance getBalance() {
return this.balance;
}
public String getCode() {
return this.code;
}
public String getUsername() {
return this.username;
}
public void setAddress(final Address address) {
this.address = address;
}
public void setNonce(final Nonce nonce) {
this.nonce = nonce;
}
public void setBalance(final Balance balance) {
this.balance = balance;
}
public void setCode(final String code) {
this.code = code;
}
public void setUsername(final String username) {
this.username = username;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Account)) return false;
final Account other = (Account) o;
if (!other.canEqual((Object) this)) return false;
final Object this$address = this.getAddress();
final Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$balance = this.getBalance();
final Object other$balance = other.getBalance();
if (this$balance == null ? other$balance != null : !this$balance.equals(other$balance)) return false;
final Object this$code = this.getCode();
final Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final Object this$username = this.getUsername();
final Object other$username = other.getUsername();
if (this$username == null ? other$username != null : !this$username.equals(other$username)) return false;
return true;
}
protected boolean canEqual(final Object other) {
return other instanceof Account;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $balance = this.getBalance();
result = result * PRIME + ($balance == null ? 43 : $balance.hashCode());
final Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final Object $username = this.getUsername();
result = result * PRIME + ($username == null ? 43 : $username.hashCode());
return result;
}
@Override
public String toString() {
return "Account(address=" + this.getAddress() + ", nonce=" + this.getNonce() + ", balance=" + this.getBalance() + ", code=" + this.getCode() + ", username=" + this.getUsername() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy