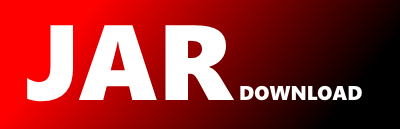
software.crldev.elrondspringbootstarterreactive.domain.esdt.ESDTIssuance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.esdt;
import lombok.NonNull;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.esdt.common.*;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.GasLimit;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.PayloadData;
import software.crldev.elrondspringbootstarterreactive.domain.wallet.Wallet;
import software.crldev.elrondspringbootstarterreactive.error.ErrorMessage;
import software.crldev.elrondspringbootstarterreactive.interactor.transaction.TransactionRequest;
import java.util.HashSet;
import java.util.Set;
import java.util.stream.Collectors;
import static io.netty.util.internal.StringUtil.EMPTY_STRING;
import static java.lang.String.format;
import static java.util.Objects.nonNull;
import static software.crldev.elrondspringbootstarterreactive.config.constants.ESDTConstants.*;
/**
* Value object for ESDT issuance (FUNGIBLE, SEMI-FUNGIBLE, NON-FUNGIBLE, META)
*
* @author carlo_stanciu
*/
public final class ESDTIssuance implements ESDTTransaction {
@NonNull
private final Type type;
@NonNull
private final TokenName tokenName;
@NonNull
private final TokenTicker tokenTicker;
private final TokenInitialSupply initialSupply;
private final TokenDecimals decimals;
private final Balance value;
private final GasLimit gasLimit;
private final Set properties;
private PayloadData processPayloadData() {
return PayloadData.fromString(format("%s@%s@%s%s%s%s", processType(), tokenName.getHex(), tokenTicker.getHex(), processSupplyArg(), processDecimalsArg(), processPropsArg()));
}
@Override
public TransactionRequest toTransactionRequest(Wallet wallet) {
return TransactionRequest.builder().receiverAddress(Address.fromBech32(ESDT_ISSUER_BECH32_ADDRESS)).data(processPayloadData()).value(value).gasLimit(gasLimit).build();
}
private String processSupplyArg() {
if (!type.equals(Type.FUNGIBLE)) return EMPTY_STRING;
return "@" + initialSupply.getHex();
}
private String processDecimalsArg() {
if (!(type.equals(Type.FUNGIBLE) || type.equals(Type.META))) return EMPTY_STRING;
return "@" + decimals.getHex();
}
private String processPropsArg() {
properties.removeIf(p -> type.equals(Type.FUNGIBLE) && p.getName().equals(TokenPropertyName.CAN_TRANSFER_NFT_CREATE_ROLE));
properties.removeIf(p -> (type.equals(Type.SEMI_FUNGIBLE) || type.equals(Type.NON_FUNGIBLE) || type.equals(Type.META)) && (p.getName().equals(TokenPropertyName.CAN_UPGRADE) || p.getName().equals(TokenPropertyName.CAN_ADD_SPECIAL_ROLES) || p.getName().equals(TokenPropertyName.CAN_CHANGE_OWNER)));
return properties.stream().map(p -> "@" + p.getNameHex() + "@" + p.getValueHex()).collect(Collectors.joining());
}
private String processType() {
switch (type) {
case FUNGIBLE:
throwIfNull(initialSupply, ErrorMessage.INITIAL_SUPPLY.getValue());
throwIfNull(decimals, ErrorMessage.TOKEN_DECIMALS.getValue());
return ESDT_FUNGIBLE_ISSUANCE_CALL;
case SEMI_FUNGIBLE:
return ESDT_SEMI_FUNGIBLE_ISSUE_CALL;
case NON_FUNGIBLE:
return ESDT_NON_FUNGIBLE_ISSUE_CALL;
case META:
throwIfNull(decimals, ErrorMessage.TOKEN_DECIMALS.getValue());
return META_ESDT_ISSUE_CALL;
default:
throw new IllegalArgumentException();
}
}
private void throwIfNull(Object target, String message) {
if (!nonNull(target)) throw new IllegalArgumentException(message);
}
public enum Type {
FUNGIBLE, SEMI_FUNGIBLE, NON_FUNGIBLE, META;
}
private static Balance $default$value() {
return Balance.fromNumber(ESDT_ISSUANCE_EGLD_COST);
}
private static GasLimit $default$gasLimit() {
return GasLimit.defaultEsdtIssuance();
}
private static Set $default$properties() {
return new HashSet<>();
}
ESDTIssuance(@NonNull final Type type, @NonNull final TokenName tokenName, @NonNull final TokenTicker tokenTicker, final TokenInitialSupply initialSupply, final TokenDecimals decimals, final Balance value, final GasLimit gasLimit, final Set properties) {
if (type == null) {
throw new NullPointerException("type is marked non-null but is null");
}
if (tokenName == null) {
throw new NullPointerException("tokenName is marked non-null but is null");
}
if (tokenTicker == null) {
throw new NullPointerException("tokenTicker is marked non-null but is null");
}
this.type = type;
this.tokenName = tokenName;
this.tokenTicker = tokenTicker;
this.initialSupply = initialSupply;
this.decimals = decimals;
this.value = value;
this.gasLimit = gasLimit;
this.properties = properties;
}
public static class ESDTIssuanceBuilder {
private Type type;
private TokenName tokenName;
private TokenTicker tokenTicker;
private TokenInitialSupply initialSupply;
private TokenDecimals decimals;
private boolean value$set;
private Balance value$value;
private boolean gasLimit$set;
private GasLimit gasLimit$value;
private boolean properties$set;
private Set properties$value;
ESDTIssuanceBuilder() {
}
/**
* @return {@code this}.
*/
public ESDTIssuance.ESDTIssuanceBuilder type(@NonNull final Type type) {
if (type == null) {
throw new NullPointerException("type is marked non-null but is null");
}
this.type = type;
return this;
}
/**
* @return {@code this}.
*/
public ESDTIssuance.ESDTIssuanceBuilder tokenName(@NonNull final TokenName tokenName) {
if (tokenName == null) {
throw new NullPointerException("tokenName is marked non-null but is null");
}
this.tokenName = tokenName;
return this;
}
/**
* @return {@code this}.
*/
public ESDTIssuance.ESDTIssuanceBuilder tokenTicker(@NonNull final TokenTicker tokenTicker) {
if (tokenTicker == null) {
throw new NullPointerException("tokenTicker is marked non-null but is null");
}
this.tokenTicker = tokenTicker;
return this;
}
/**
* @return {@code this}.
*/
public ESDTIssuance.ESDTIssuanceBuilder initialSupply(final TokenInitialSupply initialSupply) {
this.initialSupply = initialSupply;
return this;
}
/**
* @return {@code this}.
*/
public ESDTIssuance.ESDTIssuanceBuilder decimals(final TokenDecimals decimals) {
this.decimals = decimals;
return this;
}
/**
* @return {@code this}.
*/
public ESDTIssuance.ESDTIssuanceBuilder value(final Balance value) {
this.value$value = value;
value$set = true;
return this;
}
/**
* @return {@code this}.
*/
public ESDTIssuance.ESDTIssuanceBuilder gasLimit(final GasLimit gasLimit) {
this.gasLimit$value = gasLimit;
gasLimit$set = true;
return this;
}
/**
* @return {@code this}.
*/
public ESDTIssuance.ESDTIssuanceBuilder properties(final Set properties) {
this.properties$value = properties;
properties$set = true;
return this;
}
public ESDTIssuance build() {
Balance value$value = this.value$value;
if (!this.value$set) value$value = ESDTIssuance.$default$value();
GasLimit gasLimit$value = this.gasLimit$value;
if (!this.gasLimit$set) gasLimit$value = ESDTIssuance.$default$gasLimit();
Set properties$value = this.properties$value;
if (!this.properties$set) properties$value = ESDTIssuance.$default$properties();
return new ESDTIssuance(this.type, this.tokenName, this.tokenTicker, this.initialSupply, this.decimals, value$value, gasLimit$value, properties$value);
}
@Override
public String toString() {
return "ESDTIssuance.ESDTIssuanceBuilder(type=" + this.type + ", tokenName=" + this.tokenName + ", tokenTicker=" + this.tokenTicker + ", initialSupply=" + this.initialSupply + ", decimals=" + this.decimals + ", value$value=" + this.value$value + ", gasLimit$value=" + this.gasLimit$value + ", properties$value=" + this.properties$value + ")";
}
}
public static ESDTIssuance.ESDTIssuanceBuilder builder() {
return new ESDTIssuance.ESDTIssuanceBuilder();
}
@NonNull
public Type getType() {
return this.type;
}
@NonNull
public TokenName getTokenName() {
return this.tokenName;
}
@NonNull
public TokenTicker getTokenTicker() {
return this.tokenTicker;
}
public TokenInitialSupply getInitialSupply() {
return this.initialSupply;
}
public TokenDecimals getDecimals() {
return this.decimals;
}
public Balance getValue() {
return this.value;
}
public GasLimit getGasLimit() {
return this.gasLimit;
}
public Set getProperties() {
return this.properties;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ESDTIssuance)) return false;
final ESDTIssuance other = (ESDTIssuance) o;
final Object this$type = this.getType();
final Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final Object this$tokenName = this.getTokenName();
final Object other$tokenName = other.getTokenName();
if (this$tokenName == null ? other$tokenName != null : !this$tokenName.equals(other$tokenName)) return false;
final Object this$tokenTicker = this.getTokenTicker();
final Object other$tokenTicker = other.getTokenTicker();
if (this$tokenTicker == null ? other$tokenTicker != null : !this$tokenTicker.equals(other$tokenTicker)) return false;
final Object this$initialSupply = this.getInitialSupply();
final Object other$initialSupply = other.getInitialSupply();
if (this$initialSupply == null ? other$initialSupply != null : !this$initialSupply.equals(other$initialSupply)) return false;
final Object this$decimals = this.getDecimals();
final Object other$decimals = other.getDecimals();
if (this$decimals == null ? other$decimals != null : !this$decimals.equals(other$decimals)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
final Object this$properties = this.getProperties();
final Object other$properties = other.getProperties();
if (this$properties == null ? other$properties != null : !this$properties.equals(other$properties)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final Object $tokenName = this.getTokenName();
result = result * PRIME + ($tokenName == null ? 43 : $tokenName.hashCode());
final Object $tokenTicker = this.getTokenTicker();
result = result * PRIME + ($tokenTicker == null ? 43 : $tokenTicker.hashCode());
final Object $initialSupply = this.getInitialSupply();
result = result * PRIME + ($initialSupply == null ? 43 : $initialSupply.hashCode());
final Object $decimals = this.getDecimals();
result = result * PRIME + ($decimals == null ? 43 : $decimals.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
final Object $properties = this.getProperties();
result = result * PRIME + ($properties == null ? 43 : $properties.hashCode());
return result;
}
@Override
public String toString() {
return "ESDTIssuance(type=" + this.getType() + ", tokenName=" + this.getTokenName() + ", tokenTicker=" + this.getTokenTicker() + ", initialSupply=" + this.getInitialSupply() + ", decimals=" + this.getDecimals() + ", value=" + this.getValue() + ", gasLimit=" + this.getGasLimit() + ", properties=" + this.getProperties() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy