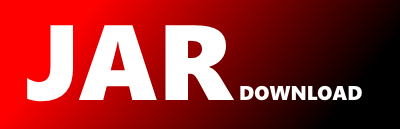
software.crldev.elrondspringbootstarterreactive.domain.esdt.ESDTLocalOp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.esdt;
import lombok.NonNull;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.esdt.ESDTTransaction;
import software.crldev.elrondspringbootstarterreactive.domain.esdt.common.TokenIdentifier;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.GasLimit;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.PayloadData;
import software.crldev.elrondspringbootstarterreactive.domain.wallet.Wallet;
import software.crldev.elrondspringbootstarterreactive.interactor.transaction.TransactionRequest;
import static java.lang.String.format;
import static software.crldev.elrondspringbootstarterreactive.config.constants.ESDTConstants.ESDT_BURN_CALL;
import static software.crldev.elrondspringbootstarterreactive.config.constants.ESDTConstants.ESDT_MINT_CALL;
/**
* Value object for ESDT Local operations minting
*
* @author carlo_stanciu
*/
public final class ESDTLocalOp implements ESDTTransaction {
@NonNull
private final Type type;
@NonNull
private final TokenIdentifier tokenIdentifier;
@NonNull
private final Balance amount;
@NonNull
private final GasLimit gasLimit;
private PayloadData processPayloadData() {
return PayloadData.fromString(format("%s@%s@%s", processType(), tokenIdentifier.getHex(), amount.getHex()));
}
@Override
public TransactionRequest toTransactionRequest(Wallet wallet) {
return TransactionRequest.builder().receiverAddress(wallet.getAddress()).data(processPayloadData()).value(Balance.zero()).gasLimit(gasLimit).build();
}
private String processType() {
return type.equals(Type.MINT) ? ESDT_MINT_CALL : ESDT_BURN_CALL;
}
public enum Type {
MINT, BURN;
}
private static GasLimit $default$gasLimit() {
return GasLimit.defaultEsdtLocalOp();
}
ESDTLocalOp(@NonNull final Type type, @NonNull final TokenIdentifier tokenIdentifier, @NonNull final Balance amount, @NonNull final GasLimit gasLimit) {
if (type == null) {
throw new NullPointerException("type is marked non-null but is null");
}
if (tokenIdentifier == null) {
throw new NullPointerException("tokenIdentifier is marked non-null but is null");
}
if (amount == null) {
throw new NullPointerException("amount is marked non-null but is null");
}
if (gasLimit == null) {
throw new NullPointerException("gasLimit is marked non-null but is null");
}
this.type = type;
this.tokenIdentifier = tokenIdentifier;
this.amount = amount;
this.gasLimit = gasLimit;
}
public static class ESDTLocalOpBuilder {
private Type type;
private TokenIdentifier tokenIdentifier;
private Balance amount;
private boolean gasLimit$set;
private GasLimit gasLimit$value;
ESDTLocalOpBuilder() {
}
/**
* @return {@code this}.
*/
public ESDTLocalOp.ESDTLocalOpBuilder type(@NonNull final Type type) {
if (type == null) {
throw new NullPointerException("type is marked non-null but is null");
}
this.type = type;
return this;
}
/**
* @return {@code this}.
*/
public ESDTLocalOp.ESDTLocalOpBuilder tokenIdentifier(@NonNull final TokenIdentifier tokenIdentifier) {
if (tokenIdentifier == null) {
throw new NullPointerException("tokenIdentifier is marked non-null but is null");
}
this.tokenIdentifier = tokenIdentifier;
return this;
}
/**
* @return {@code this}.
*/
public ESDTLocalOp.ESDTLocalOpBuilder amount(@NonNull final Balance amount) {
if (amount == null) {
throw new NullPointerException("amount is marked non-null but is null");
}
this.amount = amount;
return this;
}
/**
* @return {@code this}.
*/
public ESDTLocalOp.ESDTLocalOpBuilder gasLimit(@NonNull final GasLimit gasLimit) {
if (gasLimit == null) {
throw new NullPointerException("gasLimit is marked non-null but is null");
}
this.gasLimit$value = gasLimit;
gasLimit$set = true;
return this;
}
public ESDTLocalOp build() {
GasLimit gasLimit$value = this.gasLimit$value;
if (!this.gasLimit$set) gasLimit$value = ESDTLocalOp.$default$gasLimit();
return new ESDTLocalOp(this.type, this.tokenIdentifier, this.amount, gasLimit$value);
}
@Override
public String toString() {
return "ESDTLocalOp.ESDTLocalOpBuilder(type=" + this.type + ", tokenIdentifier=" + this.tokenIdentifier + ", amount=" + this.amount + ", gasLimit$value=" + this.gasLimit$value + ")";
}
}
public static ESDTLocalOp.ESDTLocalOpBuilder builder() {
return new ESDTLocalOp.ESDTLocalOpBuilder();
}
@NonNull
public Type getType() {
return this.type;
}
@NonNull
public TokenIdentifier getTokenIdentifier() {
return this.tokenIdentifier;
}
@NonNull
public Balance getAmount() {
return this.amount;
}
@NonNull
public GasLimit getGasLimit() {
return this.gasLimit;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ESDTLocalOp)) return false;
final ESDTLocalOp other = (ESDTLocalOp) o;
final Object this$type = this.getType();
final Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final Object this$tokenIdentifier = this.getTokenIdentifier();
final Object other$tokenIdentifier = other.getTokenIdentifier();
if (this$tokenIdentifier == null ? other$tokenIdentifier != null : !this$tokenIdentifier.equals(other$tokenIdentifier)) return false;
final Object this$amount = this.getAmount();
final Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final Object $tokenIdentifier = this.getTokenIdentifier();
result = result * PRIME + ($tokenIdentifier == null ? 43 : $tokenIdentifier.hashCode());
final Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
return result;
}
@Override
public String toString() {
return "ESDTLocalOp(type=" + this.getType() + ", tokenIdentifier=" + this.getTokenIdentifier() + ", amount=" + this.getAmount() + ", gasLimit=" + this.getGasLimit() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy