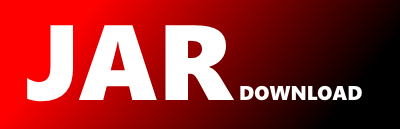
software.crldev.elrondspringbootstarterreactive.domain.esdt.NFTAttributesUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.esdt;
import lombok.NonNull;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.common.Nonce;
import software.crldev.elrondspringbootstarterreactive.domain.esdt.common.*;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.GasLimit;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.PayloadData;
import software.crldev.elrondspringbootstarterreactive.domain.wallet.Wallet;
import software.crldev.elrondspringbootstarterreactive.interactor.transaction.TransactionRequest;
import java.util.stream.Collectors;
import static java.lang.String.format;
import static software.crldev.elrondspringbootstarterreactive.config.constants.ESDTConstants.ESDT_NFT_CREATE_CALL;
import static software.crldev.elrondspringbootstarterreactive.config.constants.ESDTConstants.ESDT_NFT_UPDATE_ATTRIBUTES_CALL;
/**
* Value object for NFT attributes update
*
* @author carlo_stanciu
*/
public final class NFTAttributesUpdate implements ESDTTransaction {
@NonNull
private final TokenIdentifier tokenIdentifier;
@NonNull
private final Nonce nonce;
@NonNull
private final TokenAttributes tokenAttributes;
@NonNull
private final GasLimit gasLimit;
private PayloadData processPayloadData() {
return PayloadData.fromString(format("%s@%s@%s@%s", ESDT_NFT_UPDATE_ATTRIBUTES_CALL, tokenIdentifier.getHex(), nonce.getHex(), tokenAttributes.getHex()));
}
@Override
public TransactionRequest toTransactionRequest(Wallet wallet) {
return TransactionRequest.builder().receiverAddress(wallet.getAddress()).data(processPayloadData()).value(Balance.zero()).gasLimit(gasLimit).build();
}
private static GasLimit $default$gasLimit() {
return GasLimit.defaultNftCreate();
}
NFTAttributesUpdate(@NonNull final TokenIdentifier tokenIdentifier, @NonNull final Nonce nonce, @NonNull final TokenAttributes tokenAttributes, @NonNull final GasLimit gasLimit) {
if (tokenIdentifier == null) {
throw new NullPointerException("tokenIdentifier is marked non-null but is null");
}
if (nonce == null) {
throw new NullPointerException("nonce is marked non-null but is null");
}
if (tokenAttributes == null) {
throw new NullPointerException("tokenAttributes is marked non-null but is null");
}
if (gasLimit == null) {
throw new NullPointerException("gasLimit is marked non-null but is null");
}
this.tokenIdentifier = tokenIdentifier;
this.nonce = nonce;
this.tokenAttributes = tokenAttributes;
this.gasLimit = gasLimit;
}
public static class NFTAttributesUpdateBuilder {
private TokenIdentifier tokenIdentifier;
private Nonce nonce;
private TokenAttributes tokenAttributes;
private boolean gasLimit$set;
private GasLimit gasLimit$value;
NFTAttributesUpdateBuilder() {
}
/**
* @return {@code this}.
*/
public NFTAttributesUpdate.NFTAttributesUpdateBuilder tokenIdentifier(@NonNull final TokenIdentifier tokenIdentifier) {
if (tokenIdentifier == null) {
throw new NullPointerException("tokenIdentifier is marked non-null but is null");
}
this.tokenIdentifier = tokenIdentifier;
return this;
}
/**
* @return {@code this}.
*/
public NFTAttributesUpdate.NFTAttributesUpdateBuilder nonce(@NonNull final Nonce nonce) {
if (nonce == null) {
throw new NullPointerException("nonce is marked non-null but is null");
}
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
public NFTAttributesUpdate.NFTAttributesUpdateBuilder tokenAttributes(@NonNull final TokenAttributes tokenAttributes) {
if (tokenAttributes == null) {
throw new NullPointerException("tokenAttributes is marked non-null but is null");
}
this.tokenAttributes = tokenAttributes;
return this;
}
/**
* @return {@code this}.
*/
public NFTAttributesUpdate.NFTAttributesUpdateBuilder gasLimit(@NonNull final GasLimit gasLimit) {
if (gasLimit == null) {
throw new NullPointerException("gasLimit is marked non-null but is null");
}
this.gasLimit$value = gasLimit;
gasLimit$set = true;
return this;
}
public NFTAttributesUpdate build() {
GasLimit gasLimit$value = this.gasLimit$value;
if (!this.gasLimit$set) gasLimit$value = NFTAttributesUpdate.$default$gasLimit();
return new NFTAttributesUpdate(this.tokenIdentifier, this.nonce, this.tokenAttributes, gasLimit$value);
}
@Override
public String toString() {
return "NFTAttributesUpdate.NFTAttributesUpdateBuilder(tokenIdentifier=" + this.tokenIdentifier + ", nonce=" + this.nonce + ", tokenAttributes=" + this.tokenAttributes + ", gasLimit$value=" + this.gasLimit$value + ")";
}
}
public static NFTAttributesUpdate.NFTAttributesUpdateBuilder builder() {
return new NFTAttributesUpdate.NFTAttributesUpdateBuilder();
}
@NonNull
public TokenIdentifier getTokenIdentifier() {
return this.tokenIdentifier;
}
@NonNull
public Nonce getNonce() {
return this.nonce;
}
@NonNull
public TokenAttributes getTokenAttributes() {
return this.tokenAttributes;
}
@NonNull
public GasLimit getGasLimit() {
return this.gasLimit;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof NFTAttributesUpdate)) return false;
final NFTAttributesUpdate other = (NFTAttributesUpdate) o;
final Object this$tokenIdentifier = this.getTokenIdentifier();
final Object other$tokenIdentifier = other.getTokenIdentifier();
if (this$tokenIdentifier == null ? other$tokenIdentifier != null : !this$tokenIdentifier.equals(other$tokenIdentifier)) return false;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$tokenAttributes = this.getTokenAttributes();
final Object other$tokenAttributes = other.getTokenAttributes();
if (this$tokenAttributes == null ? other$tokenAttributes != null : !this$tokenAttributes.equals(other$tokenAttributes)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $tokenIdentifier = this.getTokenIdentifier();
result = result * PRIME + ($tokenIdentifier == null ? 43 : $tokenIdentifier.hashCode());
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $tokenAttributes = this.getTokenAttributes();
result = result * PRIME + ($tokenAttributes == null ? 43 : $tokenAttributes.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
return result;
}
@Override
public String toString() {
return "NFTAttributesUpdate(tokenIdentifier=" + this.getTokenIdentifier() + ", nonce=" + this.getNonce() + ", tokenAttributes=" + this.getTokenAttributes() + ", gasLimit=" + this.getGasLimit() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy