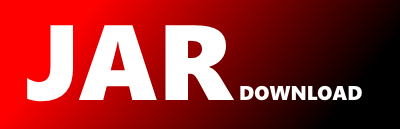
software.crldev.elrondspringbootstarterreactive.domain.esdt.common.ESDTUri Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.esdt.common;
import org.bouncycastle.util.encoders.Hex;
import org.springframework.web.util.UriUtils;
import software.crldev.elrondspringbootstarterreactive.error.ErrorMessage;
import java.net.URI;
import java.net.URISyntaxException;
import static io.netty.util.internal.StringUtil.isNullOrEmpty;
/**
* Value object for ESDT URI
*
* @author carlo_stanciu
*/
public final class ESDTUri {
private final String value;
private ESDTUri(String value) {
this.value = value;
}
/**
* Creates a ESDTUri object from String and Type inputs
*
* @return - an instance of ESDTUri
*/
public static ESDTUri fromString(String value, Type type) {
verifyNullEmpty(value, type);
var valueW = removeWhitespace(value);
try {
new URI(value);
} catch (URISyntaxException e) {
throw new IllegalArgumentException("Invalid URI syntax.");
}
verifyExtension(UriUtils.extractFileExtension(valueW), type);
return new ESDTUri(valueW);
}
/**
* Getter
*
* @return - hex value of the URI
*/
public String getHex() {
return Hex.toHexString(value.getBytes());
}
private static void verifyExtension(String extension, Type type) {
verifyNullEmpty(extension, type);
if (type.equals(Type.METADATA)) {
if (!extension.equalsIgnoreCase("json")) throw new IllegalArgumentException(ErrorMessage.NFT_METADATA_URI.getValue());
} else {
switch (extension.toLowerCase()) {
case "png":
case "jpeg":
case "jpg":
case "gif":
case "acc":
case "flac":
case "m4a":
case "mp3":
case "wav":
case "mov":
case "quicktime":
case "mp4":
case "webm":
break;
default:
throw new IllegalArgumentException(ErrorMessage.NFT_MEDIA_URI.getValue());
}
}
}
private static String removeWhitespace(String value) {
return value.replaceAll("\\s+", "");
}
private static void verifyNullEmpty(String value, Type type) {
if (isNullOrEmpty(value)) {
if (type.equals(Type.METADATA)) throw new IllegalArgumentException(ErrorMessage.NFT_METADATA_URI.getValue());
throw new IllegalArgumentException(ErrorMessage.NFT_MEDIA_URI.getValue());
}
}
public enum Type {
METADATA, MEDIA;
}
public static class ESDTUriBuilder {
private String value;
ESDTUriBuilder() {
}
/**
* @return {@code this}.
*/
public ESDTUri.ESDTUriBuilder value(final String value) {
this.value = value;
return this;
}
public ESDTUri build() {
return new ESDTUri(this.value);
}
@Override
public String toString() {
return "ESDTUri.ESDTUriBuilder(value=" + this.value + ")";
}
}
public static ESDTUri.ESDTUriBuilder builder() {
return new ESDTUri.ESDTUriBuilder();
}
public String getValue() {
return this.value;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ESDTUri)) return false;
final ESDTUri other = (ESDTUri) o;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
return result;
}
@Override
public String toString() {
return "ESDTUri(value=" + this.getValue() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy