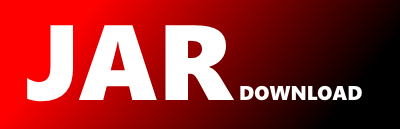
software.crldev.elrondspringbootstarterreactive.domain.esdt.common.TokenAttributes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.esdt.common;
import org.bouncycastle.util.encoders.Hex;
import software.crldev.elrondspringbootstarterreactive.error.ErrorMessage;
import java.util.Arrays;
import java.util.Set;
import java.util.stream.Collectors;
import static io.netty.util.internal.StringUtil.isNullOrEmpty;
/**
* Value object for ESDT token attributes
*
* @author carlo_stanciu
*/
public final class TokenAttributes {
private final ESDTUri metadataUri;
private final Set tags;
private TokenAttributes(ESDTUri metadataUri, Set tags) {
this.metadataUri = metadataUri;
this.tags = tags;
}
/**
* Creates a TokenName object from String inputs
*
* @return - an instance of TokenAttributes
*/
public static TokenAttributes fromString(String metadata, String[] tags) {
verifyNullEmpty(tags);
var tagsW = Arrays.stream(tags).map(TokenAttributes::removeWhitespace).collect(Collectors.toSet());
return new TokenAttributes(ESDTUri.fromString(metadata, ESDTUri.Type.METADATA), tagsW);
}
/**
* Getter
*
* @return - hex value of the Token Attributes
*/
public String getHex() {
return Hex.toHexString(toString().getBytes());
}
/**
* Returns the value
*
* @return - value in String format
*/
@Override
public String toString() {
return "metadata:" + metadataUri.getValue() + ";tags:" + String.join(",", tags);
}
private static String removeWhitespace(String value) {
return value.replaceAll("\\s+", "");
}
private static void verifyNullEmpty(String... value) {
if (value == null || value.length == 0) throw new IllegalArgumentException(ErrorMessage.TOKEN_ATTRIBUTES.getValue());
Arrays.stream(value).forEach(v -> {
if (isNullOrEmpty(v)) throw new IllegalArgumentException(ErrorMessage.TOKEN_ATTRIBUTES.getValue());
});
}
public ESDTUri getMetadataUri() {
return this.metadataUri;
}
public Set getTags() {
return this.tags;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof TokenAttributes)) return false;
final TokenAttributes other = (TokenAttributes) o;
final Object this$metadataUri = this.getMetadataUri();
final Object other$metadataUri = other.getMetadataUri();
if (this$metadataUri == null ? other$metadataUri != null : !this$metadataUri.equals(other$metadataUri)) return false;
final Object this$tags = this.getTags();
final Object other$tags = other.getTags();
if (this$tags == null ? other$tags != null : !this$tags.equals(other$tags)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $metadataUri = this.getMetadataUri();
result = result * PRIME + ($metadataUri == null ? 43 : $metadataUri.hashCode());
final Object $tags = this.getTags();
result = result * PRIME + ($tags == null ? 43 : $tags.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy