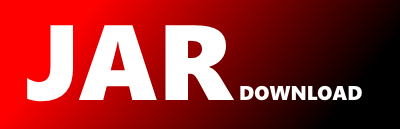
software.crldev.elrondspringbootstarterreactive.domain.esdt.common.TokenName Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.esdt.common;
import org.bouncycastle.util.encoders.Hex;
import software.crldev.elrondspringbootstarterreactive.error.ErrorMessage;
import static io.netty.util.internal.StringUtil.isNullOrEmpty;
/**
* Value object for ESDT token name
*
* @author carlo_stanciu
*/
public final class TokenName {
private final String value;
private TokenName(String name) {
this.value = name;
}
/**
* Creates a TokenName object from String input
*
* @return - an instance of TokenName
*/
public static TokenName fromString(String name) {
if (isNullOrEmpty(name)) throw new IllegalArgumentException(ErrorMessage.TOKEN_NAME.getValue());
var value = name.replaceAll("\\s+", "");
verifyFormat(value);
return new TokenName(value);
}
/**
* Getter
*
* @return - hex value of the Token Name
*/
public String getHex() {
return Hex.toHexString(value.getBytes());
}
/**
* Returns the value
*
* @return - value in String format
*/
@Override
public String toString() {
return value;
}
private static void verifyFormat(String value) {
if (!value.matches("^[a-zA-Z0-9]{3,20}+$")) throw new IllegalArgumentException(ErrorMessage.TOKEN_NAME.getValue());
}
public String getValue() {
return this.value;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof TokenName)) return false;
final TokenName other = (TokenName) o;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy