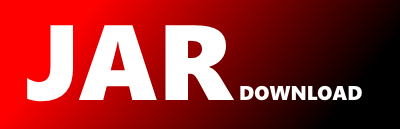
software.crldev.elrondspringbootstarterreactive.domain.esdt.common.TransferToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.esdt.common;
import lombok.NonNull;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.common.Nonce;
import software.crldev.elrondspringbootstarterreactive.domain.esdt.common.TokenIdentifier;
/**
* Value object for ESDT token data for transfer
*
* @author carlo_stanciu
*/
public final class TransferToken {
@NonNull
private final TokenIdentifier identifier;
@NonNull
private final Nonce nonce;
@NonNull
private final Balance amount;
private static Nonce $default$nonce() {
return Nonce.fromLong(0L);
}
TransferToken(@NonNull final TokenIdentifier identifier, @NonNull final Nonce nonce, @NonNull final Balance amount) {
if (identifier == null) {
throw new NullPointerException("identifier is marked non-null but is null");
}
if (nonce == null) {
throw new NullPointerException("nonce is marked non-null but is null");
}
if (amount == null) {
throw new NullPointerException("amount is marked non-null but is null");
}
this.identifier = identifier;
this.nonce = nonce;
this.amount = amount;
}
public static class TransferTokenBuilder {
private TokenIdentifier identifier;
private boolean nonce$set;
private Nonce nonce$value;
private Balance amount;
TransferTokenBuilder() {
}
/**
* @return {@code this}.
*/
public TransferToken.TransferTokenBuilder identifier(@NonNull final TokenIdentifier identifier) {
if (identifier == null) {
throw new NullPointerException("identifier is marked non-null but is null");
}
this.identifier = identifier;
return this;
}
/**
* @return {@code this}.
*/
public TransferToken.TransferTokenBuilder nonce(@NonNull final Nonce nonce) {
if (nonce == null) {
throw new NullPointerException("nonce is marked non-null but is null");
}
this.nonce$value = nonce;
nonce$set = true;
return this;
}
/**
* @return {@code this}.
*/
public TransferToken.TransferTokenBuilder amount(@NonNull final Balance amount) {
if (amount == null) {
throw new NullPointerException("amount is marked non-null but is null");
}
this.amount = amount;
return this;
}
public TransferToken build() {
Nonce nonce$value = this.nonce$value;
if (!this.nonce$set) nonce$value = TransferToken.$default$nonce();
return new TransferToken(this.identifier, nonce$value, this.amount);
}
@Override
public String toString() {
return "TransferToken.TransferTokenBuilder(identifier=" + this.identifier + ", nonce$value=" + this.nonce$value + ", amount=" + this.amount + ")";
}
}
public static TransferToken.TransferTokenBuilder builder() {
return new TransferToken.TransferTokenBuilder();
}
@NonNull
public TokenIdentifier getIdentifier() {
return this.identifier;
}
@NonNull
public Nonce getNonce() {
return this.nonce;
}
@NonNull
public Balance getAmount() {
return this.amount;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof TransferToken)) return false;
final TransferToken other = (TransferToken) o;
final Object this$identifier = this.getIdentifier();
final Object other$identifier = other.getIdentifier();
if (this$identifier == null ? other$identifier != null : !this$identifier.equals(other$identifier)) return false;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$amount = this.getAmount();
final Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $identifier = this.getIdentifier();
result = result * PRIME + ($identifier == null ? 43 : $identifier.hashCode());
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
return result;
}
@Override
public String toString() {
return "TransferToken(identifier=" + this.getIdentifier() + ", nonce=" + this.getNonce() + ", amount=" + this.getAmount() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy