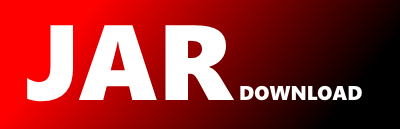
software.crldev.elrondspringbootstarterreactive.domain.smartcontract.ContractFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.smartcontract;
import lombok.NonNull;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.GasLimit;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.PayloadData;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
/**
* Value object for Smart Contract function
*
* @author carlo_stanciu
*/
public final class ContractFunction {
@NonNull
private final Address smartContractAddress;
@NonNull
private final FunctionName functionName;
@NonNull
private final List args;
@NonNull
private final Balance value;
@NonNull
private final GasLimit gasLimit;
/**
* Retrieves an instance of Contract Function
* containing the function name and arguments in the required format
*
* @return - an instance of PayloadData
*/
public PayloadData getPayloadData() {
return PayloadData.fromString(String.format("%s%s", functionName.getValue(), args.stream().map(p -> "@" + p.getHex()).collect(Collectors.joining())));
}
private static List $default$args() {
return Collections.emptyList();
}
private static Balance $default$value() {
return Balance.zero();
}
private static GasLimit $default$gasLimit() {
return GasLimit.defaultSmartContractCall();
}
ContractFunction(@NonNull final Address smartContractAddress, @NonNull final FunctionName functionName, @NonNull final List args, @NonNull final Balance value, @NonNull final GasLimit gasLimit) {
if (smartContractAddress == null) {
throw new NullPointerException("smartContractAddress is marked non-null but is null");
}
if (functionName == null) {
throw new NullPointerException("functionName is marked non-null but is null");
}
if (args == null) {
throw new NullPointerException("args is marked non-null but is null");
}
if (value == null) {
throw new NullPointerException("value is marked non-null but is null");
}
if (gasLimit == null) {
throw new NullPointerException("gasLimit is marked non-null but is null");
}
this.smartContractAddress = smartContractAddress;
this.functionName = functionName;
this.args = args;
this.value = value;
this.gasLimit = gasLimit;
}
public static class ContractFunctionBuilder {
private Address smartContractAddress;
private FunctionName functionName;
private boolean args$set;
private List args$value;
private boolean value$set;
private Balance value$value;
private boolean gasLimit$set;
private GasLimit gasLimit$value;
ContractFunctionBuilder() {
}
/**
* @return {@code this}.
*/
public ContractFunction.ContractFunctionBuilder smartContractAddress(@NonNull final Address smartContractAddress) {
if (smartContractAddress == null) {
throw new NullPointerException("smartContractAddress is marked non-null but is null");
}
this.smartContractAddress = smartContractAddress;
return this;
}
/**
* @return {@code this}.
*/
public ContractFunction.ContractFunctionBuilder functionName(@NonNull final FunctionName functionName) {
if (functionName == null) {
throw new NullPointerException("functionName is marked non-null but is null");
}
this.functionName = functionName;
return this;
}
/**
* @return {@code this}.
*/
public ContractFunction.ContractFunctionBuilder args(@NonNull final List args) {
if (args == null) {
throw new NullPointerException("args is marked non-null but is null");
}
this.args$value = args;
args$set = true;
return this;
}
/**
* @return {@code this}.
*/
public ContractFunction.ContractFunctionBuilder value(@NonNull final Balance value) {
if (value == null) {
throw new NullPointerException("value is marked non-null but is null");
}
this.value$value = value;
value$set = true;
return this;
}
/**
* @return {@code this}.
*/
public ContractFunction.ContractFunctionBuilder gasLimit(@NonNull final GasLimit gasLimit) {
if (gasLimit == null) {
throw new NullPointerException("gasLimit is marked non-null but is null");
}
this.gasLimit$value = gasLimit;
gasLimit$set = true;
return this;
}
public ContractFunction build() {
List args$value = this.args$value;
if (!this.args$set) args$value = ContractFunction.$default$args();
Balance value$value = this.value$value;
if (!this.value$set) value$value = ContractFunction.$default$value();
GasLimit gasLimit$value = this.gasLimit$value;
if (!this.gasLimit$set) gasLimit$value = ContractFunction.$default$gasLimit();
return new ContractFunction(this.smartContractAddress, this.functionName, args$value, value$value, gasLimit$value);
}
@Override
public String toString() {
return "ContractFunction.ContractFunctionBuilder(smartContractAddress=" + this.smartContractAddress + ", functionName=" + this.functionName + ", args$value=" + this.args$value + ", value$value=" + this.value$value + ", gasLimit$value=" + this.gasLimit$value + ")";
}
}
public static ContractFunction.ContractFunctionBuilder builder() {
return new ContractFunction.ContractFunctionBuilder();
}
public ContractFunction.ContractFunctionBuilder toBuilder() {
return new ContractFunction.ContractFunctionBuilder().smartContractAddress(this.smartContractAddress).functionName(this.functionName).args(this.args).value(this.value).gasLimit(this.gasLimit);
}
@NonNull
public Address getSmartContractAddress() {
return this.smartContractAddress;
}
@NonNull
public FunctionName getFunctionName() {
return this.functionName;
}
@NonNull
public List getArgs() {
return this.args;
}
@NonNull
public Balance getValue() {
return this.value;
}
@NonNull
public GasLimit getGasLimit() {
return this.gasLimit;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ContractFunction)) return false;
final ContractFunction other = (ContractFunction) o;
final Object this$smartContractAddress = this.getSmartContractAddress();
final Object other$smartContractAddress = other.getSmartContractAddress();
if (this$smartContractAddress == null ? other$smartContractAddress != null : !this$smartContractAddress.equals(other$smartContractAddress)) return false;
final Object this$functionName = this.getFunctionName();
final Object other$functionName = other.getFunctionName();
if (this$functionName == null ? other$functionName != null : !this$functionName.equals(other$functionName)) return false;
final Object this$args = this.getArgs();
final Object other$args = other.getArgs();
if (this$args == null ? other$args != null : !this$args.equals(other$args)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $smartContractAddress = this.getSmartContractAddress();
result = result * PRIME + ($smartContractAddress == null ? 43 : $smartContractAddress.hashCode());
final Object $functionName = this.getFunctionName();
result = result * PRIME + ($functionName == null ? 43 : $functionName.hashCode());
final Object $args = this.getArgs();
result = result * PRIME + ($args == null ? 43 : $args.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
return result;
}
@Override
public String toString() {
return "ContractFunction(smartContractAddress=" + this.getSmartContractAddress() + ", functionName=" + this.getFunctionName() + ", args=" + this.getArgs() + ", value=" + this.getValue() + ", gasLimit=" + this.getGasLimit() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy