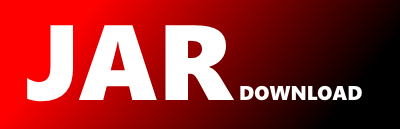
software.crldev.elrondspringbootstarterreactive.domain.smartcontract.ContractQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.smartcontract;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.AccessLevel;
import lombok.NonNull;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
/**
* Value object for Smart Contract query
*
* @author carlo_stanciu
*/
public final class ContractQuery {
@NonNull
private final Address smartContractAddress;
@NonNull
private final FunctionName functionName;
@NonNull
private final List args;
private final Address callerAddress;
private final Balance value;
/**
* Method used to create a Sendable representation of the query
* used for submitting to the ContractInteractor
*
* @return - instance of SendableTransaction
*/
public Sendable toSendable() {
return Sendable.builder().smartContractAddress(smartContractAddress.getBech32()).functionName(functionName.getValue()).args(args.stream().map(FunctionArg::getHex).collect(Collectors.toList()).toArray(String[]::new)).callerAddress(callerAddress.isZero() ? null : callerAddress.getBech32()).value(value.isZero() ? null : value.toString()).build();
}
public static final class Sendable {
@JsonProperty("scAddress")
private final String smartContractAddress;
@JsonProperty("funcName")
private final String functionName;
@JsonProperty("args")
private final String[] args;
@JsonProperty("caller")
private final String callerAddress;
@JsonProperty("value")
private final String value;
Sendable(final String smartContractAddress, final String functionName, final String[] args, final String callerAddress, final String value) {
this.smartContractAddress = smartContractAddress;
this.functionName = functionName;
this.args = args;
this.callerAddress = callerAddress;
this.value = value;
}
private static class SendableBuilder {
private String smartContractAddress;
private String functionName;
private String[] args;
private String callerAddress;
private String value;
SendableBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("scAddress")
private ContractQuery.Sendable.SendableBuilder smartContractAddress(final String smartContractAddress) {
this.smartContractAddress = smartContractAddress;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("funcName")
private ContractQuery.Sendable.SendableBuilder functionName(final String functionName) {
this.functionName = functionName;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("args")
private ContractQuery.Sendable.SendableBuilder args(final String[] args) {
this.args = args;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("caller")
private ContractQuery.Sendable.SendableBuilder callerAddress(final String callerAddress) {
this.callerAddress = callerAddress;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("value")
private ContractQuery.Sendable.SendableBuilder value(final String value) {
this.value = value;
return this;
}
private ContractQuery.Sendable build() {
return new ContractQuery.Sendable(this.smartContractAddress, this.functionName, this.args, this.callerAddress, this.value);
}
@Override
public String toString() {
return "ContractQuery.Sendable.SendableBuilder(smartContractAddress=" + this.smartContractAddress + ", functionName=" + this.functionName + ", args=" + java.util.Arrays.deepToString(this.args) + ", callerAddress=" + this.callerAddress + ", value=" + this.value + ")";
}
}
private static ContractQuery.Sendable.SendableBuilder builder() {
return new ContractQuery.Sendable.SendableBuilder();
}
public String getSmartContractAddress() {
return this.smartContractAddress;
}
public String getFunctionName() {
return this.functionName;
}
public String[] getArgs() {
return this.args;
}
public String getCallerAddress() {
return this.callerAddress;
}
public String getValue() {
return this.value;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ContractQuery.Sendable)) return false;
final ContractQuery.Sendable other = (ContractQuery.Sendable) o;
final Object this$smartContractAddress = this.getSmartContractAddress();
final Object other$smartContractAddress = other.getSmartContractAddress();
if (this$smartContractAddress == null ? other$smartContractAddress != null : !this$smartContractAddress.equals(other$smartContractAddress)) return false;
final Object this$functionName = this.getFunctionName();
final Object other$functionName = other.getFunctionName();
if (this$functionName == null ? other$functionName != null : !this$functionName.equals(other$functionName)) return false;
if (!java.util.Arrays.deepEquals(this.getArgs(), other.getArgs())) return false;
final Object this$callerAddress = this.getCallerAddress();
final Object other$callerAddress = other.getCallerAddress();
if (this$callerAddress == null ? other$callerAddress != null : !this$callerAddress.equals(other$callerAddress)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $smartContractAddress = this.getSmartContractAddress();
result = result * PRIME + ($smartContractAddress == null ? 43 : $smartContractAddress.hashCode());
final Object $functionName = this.getFunctionName();
result = result * PRIME + ($functionName == null ? 43 : $functionName.hashCode());
result = result * PRIME + java.util.Arrays.deepHashCode(this.getArgs());
final Object $callerAddress = this.getCallerAddress();
result = result * PRIME + ($callerAddress == null ? 43 : $callerAddress.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
return result;
}
@Override
public String toString() {
return "ContractQuery.Sendable(smartContractAddress=" + this.getSmartContractAddress() + ", functionName=" + this.getFunctionName() + ", args=" + java.util.Arrays.deepToString(this.getArgs()) + ", callerAddress=" + this.getCallerAddress() + ", value=" + this.getValue() + ")";
}
}
private static List $default$args() {
return Collections.emptyList();
}
private static Address $default$callerAddress() {
return Address.zero();
}
private static Balance $default$value() {
return Balance.zero();
}
ContractQuery(@NonNull final Address smartContractAddress, @NonNull final FunctionName functionName, @NonNull final List args, final Address callerAddress, final Balance value) {
if (smartContractAddress == null) {
throw new NullPointerException("smartContractAddress is marked non-null but is null");
}
if (functionName == null) {
throw new NullPointerException("functionName is marked non-null but is null");
}
if (args == null) {
throw new NullPointerException("args is marked non-null but is null");
}
this.smartContractAddress = smartContractAddress;
this.functionName = functionName;
this.args = args;
this.callerAddress = callerAddress;
this.value = value;
}
public static class ContractQueryBuilder {
private Address smartContractAddress;
private FunctionName functionName;
private boolean args$set;
private List args$value;
private boolean callerAddress$set;
private Address callerAddress$value;
private boolean value$set;
private Balance value$value;
ContractQueryBuilder() {
}
/**
* @return {@code this}.
*/
public ContractQuery.ContractQueryBuilder smartContractAddress(@NonNull final Address smartContractAddress) {
if (smartContractAddress == null) {
throw new NullPointerException("smartContractAddress is marked non-null but is null");
}
this.smartContractAddress = smartContractAddress;
return this;
}
/**
* @return {@code this}.
*/
public ContractQuery.ContractQueryBuilder functionName(@NonNull final FunctionName functionName) {
if (functionName == null) {
throw new NullPointerException("functionName is marked non-null but is null");
}
this.functionName = functionName;
return this;
}
/**
* @return {@code this}.
*/
public ContractQuery.ContractQueryBuilder args(@NonNull final List args) {
if (args == null) {
throw new NullPointerException("args is marked non-null but is null");
}
this.args$value = args;
args$set = true;
return this;
}
/**
* @return {@code this}.
*/
public ContractQuery.ContractQueryBuilder callerAddress(final Address callerAddress) {
this.callerAddress$value = callerAddress;
callerAddress$set = true;
return this;
}
/**
* @return {@code this}.
*/
public ContractQuery.ContractQueryBuilder value(final Balance value) {
this.value$value = value;
value$set = true;
return this;
}
public ContractQuery build() {
List args$value = this.args$value;
if (!this.args$set) args$value = ContractQuery.$default$args();
Address callerAddress$value = this.callerAddress$value;
if (!this.callerAddress$set) callerAddress$value = ContractQuery.$default$callerAddress();
Balance value$value = this.value$value;
if (!this.value$set) value$value = ContractQuery.$default$value();
return new ContractQuery(this.smartContractAddress, this.functionName, args$value, callerAddress$value, value$value);
}
@Override
public String toString() {
return "ContractQuery.ContractQueryBuilder(smartContractAddress=" + this.smartContractAddress + ", functionName=" + this.functionName + ", args$value=" + this.args$value + ", callerAddress$value=" + this.callerAddress$value + ", value$value=" + this.value$value + ")";
}
}
public static ContractQuery.ContractQueryBuilder builder() {
return new ContractQuery.ContractQueryBuilder();
}
@NonNull
public Address getSmartContractAddress() {
return this.smartContractAddress;
}
@NonNull
public FunctionName getFunctionName() {
return this.functionName;
}
@NonNull
public List getArgs() {
return this.args;
}
public Address getCallerAddress() {
return this.callerAddress;
}
public Balance getValue() {
return this.value;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ContractQuery)) return false;
final ContractQuery other = (ContractQuery) o;
final Object this$smartContractAddress = this.getSmartContractAddress();
final Object other$smartContractAddress = other.getSmartContractAddress();
if (this$smartContractAddress == null ? other$smartContractAddress != null : !this$smartContractAddress.equals(other$smartContractAddress)) return false;
final Object this$functionName = this.getFunctionName();
final Object other$functionName = other.getFunctionName();
if (this$functionName == null ? other$functionName != null : !this$functionName.equals(other$functionName)) return false;
final Object this$args = this.getArgs();
final Object other$args = other.getArgs();
if (this$args == null ? other$args != null : !this$args.equals(other$args)) return false;
final Object this$callerAddress = this.getCallerAddress();
final Object other$callerAddress = other.getCallerAddress();
if (this$callerAddress == null ? other$callerAddress != null : !this$callerAddress.equals(other$callerAddress)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $smartContractAddress = this.getSmartContractAddress();
result = result * PRIME + ($smartContractAddress == null ? 43 : $smartContractAddress.hashCode());
final Object $functionName = this.getFunctionName();
result = result * PRIME + ($functionName == null ? 43 : $functionName.hashCode());
final Object $args = this.getArgs();
result = result * PRIME + ($args == null ? 43 : $args.hashCode());
final Object $callerAddress = this.getCallerAddress();
result = result * PRIME + ($callerAddress == null ? 43 : $callerAddress.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
return result;
}
@Override
public String toString() {
return "ContractQuery(smartContractAddress=" + this.getSmartContractAddress() + ", functionName=" + this.getFunctionName() + ", args=" + this.getArgs() + ", callerAddress=" + this.getCallerAddress() + ", value=" + this.getValue() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy