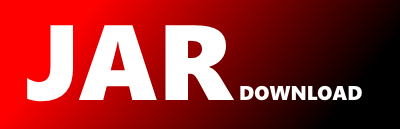
software.crldev.elrondspringbootstarterreactive.domain.transaction.Signature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.transaction;
import software.crldev.elrondspringbootstarterreactive.error.exception.CannotCreateSignatureException;
import software.crldev.elrondspringbootstarterreactive.error.exception.SignatureEmptyException;
import software.crldev.elrondspringbootstarterreactive.util.HexValidator;
import org.bouncycastle.util.encoders.Hex;
import java.util.Arrays;
import static software.crldev.elrondspringbootstarterreactive.config.constants.TransactionConstants.SIGNATURE_LENGTH;
import static software.crldev.elrondspringbootstarterreactive.config.constants.TransactionConstants.SIGNATURE_LENGTH_HEX;
/**
* Value object for Signature
*
* @author carlo_stanciu
*/
public final class Signature {
private final String hex;
private Signature(String hex) {
this.hex = hex;
}
/**
* used for creating an Signature using
* a hex representation of an Signable object
*
* @param hexValue - hex value of Signable object buffer
* @return - an instance of Signature
*/
public static Signature fromHex(String hexValue) {
if (!HexValidator.isHexValid(hexValue, SIGNATURE_LENGTH_HEX)) throw new CannotCreateSignatureException(hexValue);
return new Signature(hexValue);
}
/**
* used for creating an Signature using
* a buffer representation of an Signable object
*
* @param buffer - buffer of Signable object buffer
* @return - an instance of Signature
*/
public static Signature fromBuffer(byte[] buffer) {
if (buffer.length != SIGNATURE_LENGTH) throw new CannotCreateSignatureException(Arrays.toString(buffer));
return new Signature(Hex.toHexString(buffer));
}
/**
* Creates an empty Signature
*
* @return - an instance of Signature
*/
public static Signature empty() {
return new Signature("");
}
/**
* Getter
* Throws exception if empty
*
* @return - hex value of the Signature
*/
public String getHex() {
if (isEmpty()) throw new SignatureEmptyException();
return hex;
}
/**
* Checks if Signature is empty
*
* @return - boolean
*/
public boolean isEmpty() {
return hex.isEmpty();
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Signature)) return false;
final Signature other = (Signature) o;
final Object this$hex = this.getHex();
final Object other$hex = other.getHex();
if (this$hex == null ? other$hex != null : !this$hex.equals(other$hex)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $hex = this.getHex();
result = result * PRIME + ($hex == null ? 43 : $hex.hashCode());
return result;
}
@Override
public String toString() {
return "Signature(hex=" + this.getHex() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy