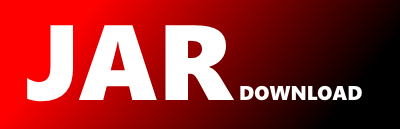
software.crldev.elrondspringbootstarterreactive.domain.transaction.Transaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.transaction;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonProcessingException;
import lombok.AccessLevel;
import software.crldev.elrondspringbootstarterreactive.config.ErdNetworkConfigSupplier;
import software.crldev.elrondspringbootstarterreactive.config.JsonMapper;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.common.Nonce;
import java.math.BigInteger;
import static software.crldev.elrondspringbootstarterreactive.util.GasUtils.computeGasCost;
/**
* Domain object for Transaction representation, which is Signable
* Contains all the required fields to validate & construct a transaction
*
* @author carlo_stanciu
*/
public class Transaction implements Signable {
private Boolean isEstimation = Boolean.FALSE;
private Nonce nonce = Nonce.fromLong(0L);
private ChainID chainID = ChainID.fromString(ErdNetworkConfigSupplier.config.getChainId());
private Balance value = Balance.zero();
private Address sender = Address.zero();
private Address receiver = Address.zero();
private GasPrice gasPrice = GasPrice.fromNumber(ErdNetworkConfigSupplier.config.getMinGasPrice());
private GasLimit gasLimit = GasLimit.fromNumber(ErdNetworkConfigSupplier.config.getMinGasLimit());
private PayloadData payloadData = PayloadData.empty();
private TransactionVersion version = TransactionVersion.withDefaultVersion();
private Signature signature = Signature.empty();
private Hash hash = Hash.empty();
private TransactionStatus status = TransactionStatus.UNKNOWN;
@Override
public byte[] serializeForSigning() throws JsonProcessingException {
return JsonMapper.serializeToJsonBuffer(toSendable());
}
@Override
public void applySignature(Signature signature) {
setSignature(signature);
}
/**
* Method used to create a Sendable representation of the transaction
* used for submitting send/sendMultiple/simulate requests to the TransactionInteractor
*
* @return - instance of SendableTransaction
*/
public Sendable toSendable() {
var sendableBuilder = Sendable.builder().chainId(chainID.getValue()).nonce(nonce.getValue()).value(value.toString()).receiver(receiver.getBech32()).sender(sender.getBech32()).data(payloadData.isEmpty() ? null : payloadData.encoded()).signature(signature.isEmpty() ? null : signature.getHex()).version(version.getValue());
if (!isEstimation) {
sendableBuilder.gasLimit(gasLimit.getValue());
sendableBuilder.gasPrice(gasPrice.getValue());
}
return sendableBuilder.build();
}
/**
* Setter
* Used for setting PayloadData
* and also computing GasCost for the data
*
* @param payloadData - data input value
*/
public void setPayloadData(PayloadData payloadData) {
this.payloadData = payloadData;
this.gasLimit = GasLimit.fromNumber(computeGasCost(payloadData));
}
/**
* This inner class represents a Transaction in a sendable format for API calls
* used for the TransactionInteractor
*/
public static final class Sendable {
@JsonProperty("nonce")
private final Long nonce;
@JsonProperty("value")
private final String value;
@JsonProperty("receiver")
private final String receiver;
@JsonProperty("sender")
private final String sender;
@JsonProperty("gasPrice")
private final BigInteger gasPrice;
@JsonProperty("gasLimit")
private final BigInteger gasLimit;
@JsonProperty("data")
private final String data;
@JsonProperty("chainID")
private final String chainId;
@JsonProperty("signature")
private final String signature;
@JsonProperty("version")
private final Integer version;
Sendable(final Long nonce, final String value, final String receiver, final String sender, final BigInteger gasPrice, final BigInteger gasLimit, final String data, final String chainId, final String signature, final Integer version) {
this.nonce = nonce;
this.value = value;
this.receiver = receiver;
this.sender = sender;
this.gasPrice = gasPrice;
this.gasLimit = gasLimit;
this.data = data;
this.chainId = chainId;
this.signature = signature;
this.version = version;
}
private static class SendableBuilder {
private Long nonce;
private String value;
private String receiver;
private String sender;
private BigInteger gasPrice;
private BigInteger gasLimit;
private String data;
private String chainId;
private String signature;
private Integer version;
SendableBuilder() {
}
/**
* @return {@code this}.
*/
@JsonProperty("nonce")
private Transaction.Sendable.SendableBuilder nonce(final Long nonce) {
this.nonce = nonce;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("value")
private Transaction.Sendable.SendableBuilder value(final String value) {
this.value = value;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("receiver")
private Transaction.Sendable.SendableBuilder receiver(final String receiver) {
this.receiver = receiver;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("sender")
private Transaction.Sendable.SendableBuilder sender(final String sender) {
this.sender = sender;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasPrice")
private Transaction.Sendable.SendableBuilder gasPrice(final BigInteger gasPrice) {
this.gasPrice = gasPrice;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("gasLimit")
private Transaction.Sendable.SendableBuilder gasLimit(final BigInteger gasLimit) {
this.gasLimit = gasLimit;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("data")
private Transaction.Sendable.SendableBuilder data(final String data) {
this.data = data;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("chainID")
private Transaction.Sendable.SendableBuilder chainId(final String chainId) {
this.chainId = chainId;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("signature")
private Transaction.Sendable.SendableBuilder signature(final String signature) {
this.signature = signature;
return this;
}
/**
* @return {@code this}.
*/
@JsonProperty("version")
private Transaction.Sendable.SendableBuilder version(final Integer version) {
this.version = version;
return this;
}
private Transaction.Sendable build() {
return new Transaction.Sendable(this.nonce, this.value, this.receiver, this.sender, this.gasPrice, this.gasLimit, this.data, this.chainId, this.signature, this.version);
}
@Override
public String toString() {
return "Transaction.Sendable.SendableBuilder(nonce=" + this.nonce + ", value=" + this.value + ", receiver=" + this.receiver + ", sender=" + this.sender + ", gasPrice=" + this.gasPrice + ", gasLimit=" + this.gasLimit + ", data=" + this.data + ", chainId=" + this.chainId + ", signature=" + this.signature + ", version=" + this.version + ")";
}
}
private static Transaction.Sendable.SendableBuilder builder() {
return new Transaction.Sendable.SendableBuilder();
}
public Long getNonce() {
return this.nonce;
}
public String getValue() {
return this.value;
}
public String getReceiver() {
return this.receiver;
}
public String getSender() {
return this.sender;
}
public BigInteger getGasPrice() {
return this.gasPrice;
}
public BigInteger getGasLimit() {
return this.gasLimit;
}
public String getData() {
return this.data;
}
public String getChainId() {
return this.chainId;
}
public String getSignature() {
return this.signature;
}
public Integer getVersion() {
return this.version;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Transaction.Sendable)) return false;
final Transaction.Sendable other = (Transaction.Sendable) o;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$version = this.getVersion();
final Object other$version = other.getVersion();
if (this$version == null ? other$version != null : !this$version.equals(other$version)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final Object this$receiver = this.getReceiver();
final Object other$receiver = other.getReceiver();
if (this$receiver == null ? other$receiver != null : !this$receiver.equals(other$receiver)) return false;
final Object this$sender = this.getSender();
final Object other$sender = other.getSender();
if (this$sender == null ? other$sender != null : !this$sender.equals(other$sender)) return false;
final Object this$gasPrice = this.getGasPrice();
final Object other$gasPrice = other.getGasPrice();
if (this$gasPrice == null ? other$gasPrice != null : !this$gasPrice.equals(other$gasPrice)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
final Object this$data = this.getData();
final Object other$data = other.getData();
if (this$data == null ? other$data != null : !this$data.equals(other$data)) return false;
final Object this$chainId = this.getChainId();
final Object other$chainId = other.getChainId();
if (this$chainId == null ? other$chainId != null : !this$chainId.equals(other$chainId)) return false;
final Object this$signature = this.getSignature();
final Object other$signature = other.getSignature();
if (this$signature == null ? other$signature != null : !this$signature.equals(other$signature)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $version = this.getVersion();
result = result * PRIME + ($version == null ? 43 : $version.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final Object $receiver = this.getReceiver();
result = result * PRIME + ($receiver == null ? 43 : $receiver.hashCode());
final Object $sender = this.getSender();
result = result * PRIME + ($sender == null ? 43 : $sender.hashCode());
final Object $gasPrice = this.getGasPrice();
result = result * PRIME + ($gasPrice == null ? 43 : $gasPrice.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
final Object $data = this.getData();
result = result * PRIME + ($data == null ? 43 : $data.hashCode());
final Object $chainId = this.getChainId();
result = result * PRIME + ($chainId == null ? 43 : $chainId.hashCode());
final Object $signature = this.getSignature();
result = result * PRIME + ($signature == null ? 43 : $signature.hashCode());
return result;
}
@Override
public String toString() {
return "Transaction.Sendable(nonce=" + this.getNonce() + ", value=" + this.getValue() + ", receiver=" + this.getReceiver() + ", sender=" + this.getSender() + ", gasPrice=" + this.getGasPrice() + ", gasLimit=" + this.getGasLimit() + ", data=" + this.getData() + ", chainId=" + this.getChainId() + ", signature=" + this.getSignature() + ", version=" + this.getVersion() + ")";
}
}
public Transaction() {
}
public Boolean getIsEstimation() {
return this.isEstimation;
}
public Nonce getNonce() {
return this.nonce;
}
public ChainID getChainID() {
return this.chainID;
}
public Balance getValue() {
return this.value;
}
public Address getSender() {
return this.sender;
}
public Address getReceiver() {
return this.receiver;
}
public GasPrice getGasPrice() {
return this.gasPrice;
}
public GasLimit getGasLimit() {
return this.gasLimit;
}
public PayloadData getPayloadData() {
return this.payloadData;
}
public TransactionVersion getVersion() {
return this.version;
}
public Signature getSignature() {
return this.signature;
}
public Hash getHash() {
return this.hash;
}
public TransactionStatus getStatus() {
return this.status;
}
public void setIsEstimation(final Boolean isEstimation) {
this.isEstimation = isEstimation;
}
public void setNonce(final Nonce nonce) {
this.nonce = nonce;
}
public void setChainID(final ChainID chainID) {
this.chainID = chainID;
}
public void setValue(final Balance value) {
this.value = value;
}
public void setSender(final Address sender) {
this.sender = sender;
}
public void setReceiver(final Address receiver) {
this.receiver = receiver;
}
public void setGasPrice(final GasPrice gasPrice) {
this.gasPrice = gasPrice;
}
public void setGasLimit(final GasLimit gasLimit) {
this.gasLimit = gasLimit;
}
public void setVersion(final TransactionVersion version) {
this.version = version;
}
public void setSignature(final Signature signature) {
this.signature = signature;
}
public void setHash(final Hash hash) {
this.hash = hash;
}
public void setStatus(final TransactionStatus status) {
this.status = status;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Transaction)) return false;
final Transaction other = (Transaction) o;
if (!other.canEqual((Object) this)) return false;
final Object this$isEstimation = this.getIsEstimation();
final Object other$isEstimation = other.getIsEstimation();
if (this$isEstimation == null ? other$isEstimation != null : !this$isEstimation.equals(other$isEstimation)) return false;
final Object this$nonce = this.getNonce();
final Object other$nonce = other.getNonce();
if (this$nonce == null ? other$nonce != null : !this$nonce.equals(other$nonce)) return false;
final Object this$chainID = this.getChainID();
final Object other$chainID = other.getChainID();
if (this$chainID == null ? other$chainID != null : !this$chainID.equals(other$chainID)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final Object this$sender = this.getSender();
final Object other$sender = other.getSender();
if (this$sender == null ? other$sender != null : !this$sender.equals(other$sender)) return false;
final Object this$receiver = this.getReceiver();
final Object other$receiver = other.getReceiver();
if (this$receiver == null ? other$receiver != null : !this$receiver.equals(other$receiver)) return false;
final Object this$gasPrice = this.getGasPrice();
final Object other$gasPrice = other.getGasPrice();
if (this$gasPrice == null ? other$gasPrice != null : !this$gasPrice.equals(other$gasPrice)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
final Object this$payloadData = this.getPayloadData();
final Object other$payloadData = other.getPayloadData();
if (this$payloadData == null ? other$payloadData != null : !this$payloadData.equals(other$payloadData)) return false;
final Object this$version = this.getVersion();
final Object other$version = other.getVersion();
if (this$version == null ? other$version != null : !this$version.equals(other$version)) return false;
final Object this$signature = this.getSignature();
final Object other$signature = other.getSignature();
if (this$signature == null ? other$signature != null : !this$signature.equals(other$signature)) return false;
final Object this$hash = this.getHash();
final Object other$hash = other.getHash();
if (this$hash == null ? other$hash != null : !this$hash.equals(other$hash)) return false;
final Object this$status = this.getStatus();
final Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
protected boolean canEqual(final Object other) {
return other instanceof Transaction;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $isEstimation = this.getIsEstimation();
result = result * PRIME + ($isEstimation == null ? 43 : $isEstimation.hashCode());
final Object $nonce = this.getNonce();
result = result * PRIME + ($nonce == null ? 43 : $nonce.hashCode());
final Object $chainID = this.getChainID();
result = result * PRIME + ($chainID == null ? 43 : $chainID.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final Object $sender = this.getSender();
result = result * PRIME + ($sender == null ? 43 : $sender.hashCode());
final Object $receiver = this.getReceiver();
result = result * PRIME + ($receiver == null ? 43 : $receiver.hashCode());
final Object $gasPrice = this.getGasPrice();
result = result * PRIME + ($gasPrice == null ? 43 : $gasPrice.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
final Object $payloadData = this.getPayloadData();
result = result * PRIME + ($payloadData == null ? 43 : $payloadData.hashCode());
final Object $version = this.getVersion();
result = result * PRIME + ($version == null ? 43 : $version.hashCode());
final Object $signature = this.getSignature();
result = result * PRIME + ($signature == null ? 43 : $signature.hashCode());
final Object $hash = this.getHash();
result = result * PRIME + ($hash == null ? 43 : $hash.hashCode());
final Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
@Override
public String toString() {
return "Transaction(isEstimation=" + this.getIsEstimation() + ", nonce=" + this.getNonce() + ", chainID=" + this.getChainID() + ", value=" + this.getValue() + ", sender=" + this.getSender() + ", receiver=" + this.getReceiver() + ", gasPrice=" + this.getGasPrice() + ", gasLimit=" + this.getGasLimit() + ", payloadData=" + this.getPayloadData() + ", version=" + this.getVersion() + ", signature=" + this.getSignature() + ", hash=" + this.getHash() + ", status=" + this.getStatus() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy