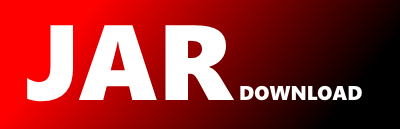
software.crldev.elrondspringbootstarterreactive.domain.wallet.Wallet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.domain.wallet;
import com.fasterxml.jackson.core.JsonProcessingException;
import org.bouncycastle.crypto.params.Ed25519PrivateKeyParameters;
import org.bouncycastle.crypto.signers.Ed25519Signer;
import org.bouncycastle.util.encoders.Hex;
import org.springframework.http.codec.multipart.FilePart;
import reactor.core.publisher.Mono;
import software.crldev.elrondspringbootstarterreactive.config.constants.WalletConstants;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.Signable;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.Signature;
import software.crldev.elrondspringbootstarterreactive.error.exception.CannotCreateSignatureException;
import software.crldev.elrondspringbootstarterreactive.error.exception.CannotSignTransactionException;
import software.crldev.elrondspringbootstarterreactive.error.exception.PrivateKeyHexSizeException;
import software.crldev.elrondspringbootstarterreactive.util.MnemonicsUtils;
import software.crldev.elrondspringbootstarterreactive.util.PemUtils;
import java.io.File;
import java.util.List;
/**
* Value object for Wallet
*/
public final class Wallet {
private final byte[] publicKey;
private final byte[] privateKey;
private Wallet(byte[] publicKey, byte[] privateKey) {
this.publicKey = publicKey;
this.privateKey = privateKey;
}
/**
* Method used for creating a Wallet using a private key buffer
*
* @param privateKey - private key in buffer format
* @return - an instance of Wallet
*/
public static Wallet fromPrivateKeyBuffer(byte[] privateKey) {
var privateKeyParameters = new Ed25519PrivateKeyParameters(privateKey, 0);
var publicKeyParameters = privateKeyParameters.generatePublicKey();
return new Wallet(publicKeyParameters.getEncoded(), privateKey);
}
/**
* Method used for creating Wallet instance
* using private key from PEM file (File format)
*
* @param pem - required PEM file
* @return - an instance of Wallet
*/
public static Wallet fromPemFile(File pem) {
return fromPrivateKeyHex(PemUtils.extractKeyHex(pem));
}
/**
* Method used for creating Wallet instance
* using private key from PEM file (FilePart format)
* Used in reactive file input
*
* @param pem - required PEM file
* @return - an instance of Wallet
*/
public static Mono fromPemFile(FilePart pem) {
return PemUtils.extractKeyHex(pem).map(Wallet::fromPrivateKeyHex);
}
/**
* Method used for creating Wallet instance
* using mnemonics
*
* @param mnemonics - mnemonic phrase in a List
* @param accountIndex - long value of index
* @return - an instance of Wallet
*/
public static Wallet fromMnemonic(List mnemonics, long accountIndex) {
var privateKey = MnemonicsUtils.privateKeyFromMnemonic(mnemonics, accountIndex);
return Wallet.fromPrivateKeyBuffer(privateKey);
}
/**
* Method used for creating a Wallet using a private key hex
*
* @param privateKeyHex - private key in hex representation
* @return - an instance of Wallet
*/
public static Wallet fromPrivateKeyHex(String privateKeyHex) {
if (privateKeyHex.length() == WalletConstants.PRIVATE_KEY_LENGTH) return fromPrivateKeyBuffer(Hex.decode(privateKeyHex));
throw new PrivateKeyHexSizeException(privateKeyHex.length());
}
/**
* Getter
*
* @return - an instance of Address owning the Wallet
*/
public Address getAddress() {
return Address.fromHex(getPublicKeyHex());
}
/**
* Method used for signing a Signable object
* using Ed25519Signer
*
* @param signable - object to sign
*/
public void sign(Signable signable) {
try {
var data = signable.serializeForSigning();
var signer = createEd25519Signer();
signer.update(data, 0, data.length);
signable.applySignature(Signature.fromBuffer(signer.generateSignature()));
} catch (JsonProcessingException | CannotCreateSignatureException e) {
throw new CannotSignTransactionException(e);
}
}
/**
* Getter
*
* @return - hex value of public key
*/
public String getPublicKeyHex() {
return Hex.toHexString(publicKey);
}
private Ed25519Signer createEd25519Signer() {
var parameters = new Ed25519PrivateKeyParameters(this.privateKey, 0);
var signer = new Ed25519Signer();
signer.init(true, parameters);
return signer;
}
public byte[] getPublicKey() {
return this.publicKey;
}
public byte[] getPrivateKey() {
return this.privateKey;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Wallet)) return false;
final Wallet other = (Wallet) o;
if (!java.util.Arrays.equals(this.getPublicKey(), other.getPublicKey())) return false;
if (!java.util.Arrays.equals(this.getPrivateKey(), other.getPrivateKey())) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + java.util.Arrays.hashCode(this.getPublicKey());
result = result * PRIME + java.util.Arrays.hashCode(this.getPrivateKey());
return result;
}
@Override
public String toString() {
return "Wallet(publicKey=" + java.util.Arrays.toString(this.getPublicKey()) + ", privateKey=" + java.util.Arrays.toString(this.getPrivateKey()) + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy