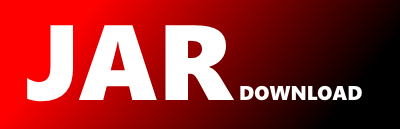
software.crldev.elrondspringbootstarterreactive.interactor.transaction.TransactionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elrond-spring-boot-starter-reactive Show documentation
Show all versions of elrond-spring-boot-starter-reactive Show documentation
A SpringBoot Starter solution designed to ensure easy and efficient integration with the Elrond Network using a Reactive API layer.
The newest version!
package software.crldev.elrondspringbootstarterreactive.interactor.transaction;
import lombok.NonNull;
import software.crldev.elrondspringbootstarterreactive.domain.account.Address;
import software.crldev.elrondspringbootstarterreactive.domain.common.Balance;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.GasLimit;
import software.crldev.elrondspringbootstarterreactive.domain.transaction.PayloadData;
/**
* Object used as a payload in the TransactionSender's methods
*
* @author carlo_stanciu
*/
public final class TransactionRequest {
@NonNull
private final Address receiverAddress;
@NonNull
private final Balance value;
@NonNull
private final PayloadData data;
@NonNull
private final GasLimit gasLimit;
private static GasLimit $default$gasLimit() {
return GasLimit.zero();
}
TransactionRequest(@NonNull final Address receiverAddress, @NonNull final Balance value, @NonNull final PayloadData data, @NonNull final GasLimit gasLimit) {
if (receiverAddress == null) {
throw new NullPointerException("receiverAddress is marked non-null but is null");
}
if (value == null) {
throw new NullPointerException("value is marked non-null but is null");
}
if (data == null) {
throw new NullPointerException("data is marked non-null but is null");
}
if (gasLimit == null) {
throw new NullPointerException("gasLimit is marked non-null but is null");
}
this.receiverAddress = receiverAddress;
this.value = value;
this.data = data;
this.gasLimit = gasLimit;
}
public static class TransactionRequestBuilder {
private Address receiverAddress;
private Balance value;
private PayloadData data;
private boolean gasLimit$set;
private GasLimit gasLimit$value;
TransactionRequestBuilder() {
}
/**
* @return {@code this}.
*/
public TransactionRequest.TransactionRequestBuilder receiverAddress(@NonNull final Address receiverAddress) {
if (receiverAddress == null) {
throw new NullPointerException("receiverAddress is marked non-null but is null");
}
this.receiverAddress = receiverAddress;
return this;
}
/**
* @return {@code this}.
*/
public TransactionRequest.TransactionRequestBuilder value(@NonNull final Balance value) {
if (value == null) {
throw new NullPointerException("value is marked non-null but is null");
}
this.value = value;
return this;
}
/**
* @return {@code this}.
*/
public TransactionRequest.TransactionRequestBuilder data(@NonNull final PayloadData data) {
if (data == null) {
throw new NullPointerException("data is marked non-null but is null");
}
this.data = data;
return this;
}
/**
* @return {@code this}.
*/
public TransactionRequest.TransactionRequestBuilder gasLimit(@NonNull final GasLimit gasLimit) {
if (gasLimit == null) {
throw new NullPointerException("gasLimit is marked non-null but is null");
}
this.gasLimit$value = gasLimit;
gasLimit$set = true;
return this;
}
public TransactionRequest build() {
GasLimit gasLimit$value = this.gasLimit$value;
if (!this.gasLimit$set) gasLimit$value = TransactionRequest.$default$gasLimit();
return new TransactionRequest(this.receiverAddress, this.value, this.data, gasLimit$value);
}
@Override
public String toString() {
return "TransactionRequest.TransactionRequestBuilder(receiverAddress=" + this.receiverAddress + ", value=" + this.value + ", data=" + this.data + ", gasLimit$value=" + this.gasLimit$value + ")";
}
}
public static TransactionRequest.TransactionRequestBuilder builder() {
return new TransactionRequest.TransactionRequestBuilder();
}
@NonNull
public Address getReceiverAddress() {
return this.receiverAddress;
}
@NonNull
public Balance getValue() {
return this.value;
}
@NonNull
public PayloadData getData() {
return this.data;
}
@NonNull
public GasLimit getGasLimit() {
return this.gasLimit;
}
@Override
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof TransactionRequest)) return false;
final TransactionRequest other = (TransactionRequest) o;
final Object this$receiverAddress = this.getReceiverAddress();
final Object other$receiverAddress = other.getReceiverAddress();
if (this$receiverAddress == null ? other$receiverAddress != null : !this$receiverAddress.equals(other$receiverAddress)) return false;
final Object this$value = this.getValue();
final Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
final Object this$data = this.getData();
final Object other$data = other.getData();
if (this$data == null ? other$data != null : !this$data.equals(other$data)) return false;
final Object this$gasLimit = this.getGasLimit();
final Object other$gasLimit = other.getGasLimit();
if (this$gasLimit == null ? other$gasLimit != null : !this$gasLimit.equals(other$gasLimit)) return false;
return true;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $receiverAddress = this.getReceiverAddress();
result = result * PRIME + ($receiverAddress == null ? 43 : $receiverAddress.hashCode());
final Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
final Object $data = this.getData();
result = result * PRIME + ($data == null ? 43 : $data.hashCode());
final Object $gasLimit = this.getGasLimit();
result = result * PRIME + ($gasLimit == null ? 43 : $gasLimit.hashCode());
return result;
}
@Override
public String toString() {
return "TransactionRequest(receiverAddress=" + this.getReceiverAddress() + ", value=" + this.getValue() + ", data=" + this.getData() + ", gasLimit=" + this.getGasLimit() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy