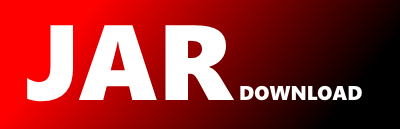
grpc.control_client.ControlclientGrpcKt.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of client-protos-jvm Show documentation
Show all versions of client-protos-jvm Show documentation
Kotlin protobuf protocols for the JVM that define the Momento gRPC wire format
package grpc.control_client
import grpc.control_client.ScsControlGrpc.getServiceDescriptor
import io.grpc.CallOptions
import io.grpc.CallOptions.DEFAULT
import io.grpc.Channel
import io.grpc.Metadata
import io.grpc.MethodDescriptor
import io.grpc.ServerServiceDefinition
import io.grpc.ServerServiceDefinition.builder
import io.grpc.ServiceDescriptor
import io.grpc.Status.UNIMPLEMENTED
import io.grpc.StatusException
import io.grpc.kotlin.AbstractCoroutineServerImpl
import io.grpc.kotlin.AbstractCoroutineStub
import io.grpc.kotlin.ClientCalls.unaryRpc
import io.grpc.kotlin.ServerCalls.unaryServerMethodDefinition
import io.grpc.kotlin.StubFor
import kotlin.String
import kotlin.coroutines.CoroutineContext
import kotlin.coroutines.EmptyCoroutineContext
import kotlin.jvm.JvmOverloads
import kotlin.jvm.JvmStatic
/**
* Holder for Kotlin coroutine-based client and server APIs for control_client.ScsControl.
*/
public object ScsControlGrpcKt {
public const val SERVICE_NAME: String = ScsControlGrpc.SERVICE_NAME
@JvmStatic
public val serviceDescriptor: ServiceDescriptor
get() = getServiceDescriptor()
public val createCacheMethod: MethodDescriptor<_CreateCacheRequest, _CreateCacheResponse>
@JvmStatic
get() = ScsControlGrpc.getCreateCacheMethod()
public val deleteCacheMethod: MethodDescriptor<_DeleteCacheRequest, _DeleteCacheResponse>
@JvmStatic
get() = ScsControlGrpc.getDeleteCacheMethod()
public val listCachesMethod: MethodDescriptor<_ListCachesRequest, _ListCachesResponse>
@JvmStatic
get() = ScsControlGrpc.getListCachesMethod()
public val flushCacheMethod: MethodDescriptor<_FlushCacheRequest, _FlushCacheResponse>
@JvmStatic
get() = ScsControlGrpc.getFlushCacheMethod()
public val createSigningKeyMethod:
MethodDescriptor<_CreateSigningKeyRequest, _CreateSigningKeyResponse>
@JvmStatic
get() = ScsControlGrpc.getCreateSigningKeyMethod()
public val revokeSigningKeyMethod:
MethodDescriptor<_RevokeSigningKeyRequest, _RevokeSigningKeyResponse>
@JvmStatic
get() = ScsControlGrpc.getRevokeSigningKeyMethod()
public val listSigningKeysMethod:
MethodDescriptor<_ListSigningKeysRequest, _ListSigningKeysResponse>
@JvmStatic
get() = ScsControlGrpc.getListSigningKeysMethod()
public val createIndexMethod: MethodDescriptor<_CreateIndexRequest, _CreateIndexResponse>
@JvmStatic
get() = ScsControlGrpc.getCreateIndexMethod()
public val deleteIndexMethod: MethodDescriptor<_DeleteIndexRequest, _DeleteIndexResponse>
@JvmStatic
get() = ScsControlGrpc.getDeleteIndexMethod()
public val listIndexesMethod: MethodDescriptor<_ListIndexesRequest, _ListIndexesResponse>
@JvmStatic
get() = ScsControlGrpc.getListIndexesMethod()
/**
* A stub for issuing RPCs to a(n) control_client.ScsControl service as suspending coroutines.
*/
@StubFor(ScsControlGrpc::class)
public class ScsControlCoroutineStub @JvmOverloads constructor(
channel: Channel,
callOptions: CallOptions = DEFAULT,
) : AbstractCoroutineStub(channel, callOptions) {
override fun build(channel: Channel, callOptions: CallOptions): ScsControlCoroutineStub =
ScsControlCoroutineStub(channel, callOptions)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun createCache(request: _CreateCacheRequest, headers: Metadata = Metadata()):
_CreateCacheResponse = unaryRpc(
channel,
ScsControlGrpc.getCreateCacheMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun deleteCache(request: _DeleteCacheRequest, headers: Metadata = Metadata()):
_DeleteCacheResponse = unaryRpc(
channel,
ScsControlGrpc.getDeleteCacheMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun listCaches(request: _ListCachesRequest, headers: Metadata = Metadata()):
_ListCachesResponse = unaryRpc(
channel,
ScsControlGrpc.getListCachesMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun flushCache(request: _FlushCacheRequest, headers: Metadata = Metadata()):
_FlushCacheResponse = unaryRpc(
channel,
ScsControlGrpc.getFlushCacheMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun createSigningKey(request: _CreateSigningKeyRequest, headers: Metadata =
Metadata()): _CreateSigningKeyResponse = unaryRpc(
channel,
ScsControlGrpc.getCreateSigningKeyMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun revokeSigningKey(request: _RevokeSigningKeyRequest, headers: Metadata =
Metadata()): _RevokeSigningKeyResponse = unaryRpc(
channel,
ScsControlGrpc.getRevokeSigningKeyMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun listSigningKeys(request: _ListSigningKeysRequest, headers: Metadata =
Metadata()): _ListSigningKeysResponse = unaryRpc(
channel,
ScsControlGrpc.getListSigningKeysMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun createIndex(request: _CreateIndexRequest, headers: Metadata = Metadata()):
_CreateIndexResponse = unaryRpc(
channel,
ScsControlGrpc.getCreateIndexMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun deleteIndex(request: _DeleteIndexRequest, headers: Metadata = Metadata()):
_DeleteIndexResponse = unaryRpc(
channel,
ScsControlGrpc.getDeleteIndexMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun listIndexes(request: _ListIndexesRequest, headers: Metadata = Metadata()):
_ListIndexesResponse = unaryRpc(
channel,
ScsControlGrpc.getListIndexesMethod(),
request,
callOptions,
headers
)
}
/**
* Skeletal implementation of the control_client.ScsControl service based on Kotlin coroutines.
*/
public abstract class ScsControlCoroutineImplBase(
coroutineContext: CoroutineContext = EmptyCoroutineContext,
) : AbstractCoroutineServerImpl(coroutineContext) {
/**
* Returns the response to an RPC for control_client.ScsControl.CreateCache.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun createCache(request: _CreateCacheRequest): _CreateCacheResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.CreateCache is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.DeleteCache.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun deleteCache(request: _DeleteCacheRequest): _DeleteCacheResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.DeleteCache is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.ListCaches.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun listCaches(request: _ListCachesRequest): _ListCachesResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.ListCaches is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.FlushCache.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun flushCache(request: _FlushCacheRequest): _FlushCacheResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.FlushCache is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.CreateSigningKey.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun createSigningKey(request: _CreateSigningKeyRequest):
_CreateSigningKeyResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.CreateSigningKey is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.RevokeSigningKey.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun revokeSigningKey(request: _RevokeSigningKeyRequest):
_RevokeSigningKeyResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.RevokeSigningKey is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.ListSigningKeys.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun listSigningKeys(request: _ListSigningKeysRequest):
_ListSigningKeysResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.ListSigningKeys is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.CreateIndex.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun createIndex(request: _CreateIndexRequest): _CreateIndexResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.CreateIndex is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.DeleteIndex.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun deleteIndex(request: _DeleteIndexRequest): _DeleteIndexResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.DeleteIndex is unimplemented"))
/**
* Returns the response to an RPC for control_client.ScsControl.ListIndexes.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun listIndexes(request: _ListIndexesRequest): _ListIndexesResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method control_client.ScsControl.ListIndexes is unimplemented"))
final override fun bindService(): ServerServiceDefinition = builder(getServiceDescriptor())
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getCreateCacheMethod(),
implementation = ::createCache
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getDeleteCacheMethod(),
implementation = ::deleteCache
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getListCachesMethod(),
implementation = ::listCaches
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getFlushCacheMethod(),
implementation = ::flushCache
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getCreateSigningKeyMethod(),
implementation = ::createSigningKey
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getRevokeSigningKeyMethod(),
implementation = ::revokeSigningKey
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getListSigningKeysMethod(),
implementation = ::listSigningKeys
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getCreateIndexMethod(),
implementation = ::createIndex
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getDeleteIndexMethod(),
implementation = ::deleteIndex
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = ScsControlGrpc.getListIndexesMethod(),
implementation = ::listIndexes
)).build()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy