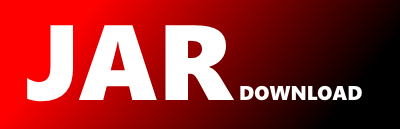
grpc.vectorindex.VectorIndexGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of client-protos-jvm Show documentation
Show all versions of client-protos-jvm Show documentation
Kotlin protobuf protocols for the JVM that define the Momento gRPC wire format
package grpc.vectorindex;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.57.2)",
comments = "Source: vectorindex.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class VectorIndexGrpc {
private VectorIndexGrpc() {}
public static final java.lang.String SERVICE_NAME = "vectorindex.VectorIndex";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getUpsertItemBatchMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "UpsertItemBatch",
requestType = grpc.vectorindex._UpsertItemBatchRequest.class,
responseType = grpc.vectorindex._UpsertItemBatchResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUpsertItemBatchMethod() {
io.grpc.MethodDescriptor getUpsertItemBatchMethod;
if ((getUpsertItemBatchMethod = VectorIndexGrpc.getUpsertItemBatchMethod) == null) {
synchronized (VectorIndexGrpc.class) {
if ((getUpsertItemBatchMethod = VectorIndexGrpc.getUpsertItemBatchMethod) == null) {
VectorIndexGrpc.getUpsertItemBatchMethod = getUpsertItemBatchMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "UpsertItemBatch"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._UpsertItemBatchRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._UpsertItemBatchResponse.getDefaultInstance()))
.setSchemaDescriptor(new VectorIndexMethodDescriptorSupplier("UpsertItemBatch"))
.build();
}
}
}
return getUpsertItemBatchMethod;
}
private static volatile io.grpc.MethodDescriptor getDeleteItemBatchMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DeleteItemBatch",
requestType = grpc.vectorindex._DeleteItemBatchRequest.class,
responseType = grpc.vectorindex._DeleteItemBatchResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeleteItemBatchMethod() {
io.grpc.MethodDescriptor getDeleteItemBatchMethod;
if ((getDeleteItemBatchMethod = VectorIndexGrpc.getDeleteItemBatchMethod) == null) {
synchronized (VectorIndexGrpc.class) {
if ((getDeleteItemBatchMethod = VectorIndexGrpc.getDeleteItemBatchMethod) == null) {
VectorIndexGrpc.getDeleteItemBatchMethod = getDeleteItemBatchMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DeleteItemBatch"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._DeleteItemBatchRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._DeleteItemBatchResponse.getDefaultInstance()))
.setSchemaDescriptor(new VectorIndexMethodDescriptorSupplier("DeleteItemBatch"))
.build();
}
}
}
return getDeleteItemBatchMethod;
}
private static volatile io.grpc.MethodDescriptor getSearchMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Search",
requestType = grpc.vectorindex._SearchRequest.class,
responseType = grpc.vectorindex._SearchResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSearchMethod() {
io.grpc.MethodDescriptor getSearchMethod;
if ((getSearchMethod = VectorIndexGrpc.getSearchMethod) == null) {
synchronized (VectorIndexGrpc.class) {
if ((getSearchMethod = VectorIndexGrpc.getSearchMethod) == null) {
VectorIndexGrpc.getSearchMethod = getSearchMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Search"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._SearchRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._SearchResponse.getDefaultInstance()))
.setSchemaDescriptor(new VectorIndexMethodDescriptorSupplier("Search"))
.build();
}
}
}
return getSearchMethod;
}
private static volatile io.grpc.MethodDescriptor getSearchAndFetchVectorsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "SearchAndFetchVectors",
requestType = grpc.vectorindex._SearchAndFetchVectorsRequest.class,
responseType = grpc.vectorindex._SearchAndFetchVectorsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSearchAndFetchVectorsMethod() {
io.grpc.MethodDescriptor getSearchAndFetchVectorsMethod;
if ((getSearchAndFetchVectorsMethod = VectorIndexGrpc.getSearchAndFetchVectorsMethod) == null) {
synchronized (VectorIndexGrpc.class) {
if ((getSearchAndFetchVectorsMethod = VectorIndexGrpc.getSearchAndFetchVectorsMethod) == null) {
VectorIndexGrpc.getSearchAndFetchVectorsMethod = getSearchAndFetchVectorsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "SearchAndFetchVectors"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._SearchAndFetchVectorsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._SearchAndFetchVectorsResponse.getDefaultInstance()))
.setSchemaDescriptor(new VectorIndexMethodDescriptorSupplier("SearchAndFetchVectors"))
.build();
}
}
}
return getSearchAndFetchVectorsMethod;
}
private static volatile io.grpc.MethodDescriptor getGetItemMetadataBatchMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetItemMetadataBatch",
requestType = grpc.vectorindex._GetItemMetadataBatchRequest.class,
responseType = grpc.vectorindex._GetItemMetadataBatchResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetItemMetadataBatchMethod() {
io.grpc.MethodDescriptor getGetItemMetadataBatchMethod;
if ((getGetItemMetadataBatchMethod = VectorIndexGrpc.getGetItemMetadataBatchMethod) == null) {
synchronized (VectorIndexGrpc.class) {
if ((getGetItemMetadataBatchMethod = VectorIndexGrpc.getGetItemMetadataBatchMethod) == null) {
VectorIndexGrpc.getGetItemMetadataBatchMethod = getGetItemMetadataBatchMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetItemMetadataBatch"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._GetItemMetadataBatchRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._GetItemMetadataBatchResponse.getDefaultInstance()))
.setSchemaDescriptor(new VectorIndexMethodDescriptorSupplier("GetItemMetadataBatch"))
.build();
}
}
}
return getGetItemMetadataBatchMethod;
}
private static volatile io.grpc.MethodDescriptor getGetItemBatchMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetItemBatch",
requestType = grpc.vectorindex._GetItemBatchRequest.class,
responseType = grpc.vectorindex._GetItemBatchResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetItemBatchMethod() {
io.grpc.MethodDescriptor getGetItemBatchMethod;
if ((getGetItemBatchMethod = VectorIndexGrpc.getGetItemBatchMethod) == null) {
synchronized (VectorIndexGrpc.class) {
if ((getGetItemBatchMethod = VectorIndexGrpc.getGetItemBatchMethod) == null) {
VectorIndexGrpc.getGetItemBatchMethod = getGetItemBatchMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetItemBatch"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._GetItemBatchRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._GetItemBatchResponse.getDefaultInstance()))
.setSchemaDescriptor(new VectorIndexMethodDescriptorSupplier("GetItemBatch"))
.build();
}
}
}
return getGetItemBatchMethod;
}
private static volatile io.grpc.MethodDescriptor getCountItemsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CountItems",
requestType = grpc.vectorindex._CountItemsRequest.class,
responseType = grpc.vectorindex._CountItemsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCountItemsMethod() {
io.grpc.MethodDescriptor getCountItemsMethod;
if ((getCountItemsMethod = VectorIndexGrpc.getCountItemsMethod) == null) {
synchronized (VectorIndexGrpc.class) {
if ((getCountItemsMethod = VectorIndexGrpc.getCountItemsMethod) == null) {
VectorIndexGrpc.getCountItemsMethod = getCountItemsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CountItems"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._CountItemsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
grpc.vectorindex._CountItemsResponse.getDefaultInstance()))
.setSchemaDescriptor(new VectorIndexMethodDescriptorSupplier("CountItems"))
.build();
}
}
}
return getCountItemsMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static VectorIndexStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public VectorIndexStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new VectorIndexStub(channel, callOptions);
}
};
return VectorIndexStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static VectorIndexBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public VectorIndexBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new VectorIndexBlockingStub(channel, callOptions);
}
};
return VectorIndexBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static VectorIndexFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public VectorIndexFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new VectorIndexFutureStub(channel, callOptions);
}
};
return VectorIndexFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*/
default void upsertItemBatch(grpc.vectorindex._UpsertItemBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getUpsertItemBatchMethod(), responseObserver);
}
/**
*/
default void deleteItemBatch(grpc.vectorindex._DeleteItemBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDeleteItemBatchMethod(), responseObserver);
}
/**
*/
default void search(grpc.vectorindex._SearchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSearchMethod(), responseObserver);
}
/**
*/
default void searchAndFetchVectors(grpc.vectorindex._SearchAndFetchVectorsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getSearchAndFetchVectorsMethod(), responseObserver);
}
/**
*/
default void getItemMetadataBatch(grpc.vectorindex._GetItemMetadataBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetItemMetadataBatchMethod(), responseObserver);
}
/**
*/
default void getItemBatch(grpc.vectorindex._GetItemBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetItemBatchMethod(), responseObserver);
}
/**
*/
default void countItems(grpc.vectorindex._CountItemsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCountItemsMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service VectorIndex.
*/
public static abstract class VectorIndexImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return VectorIndexGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service VectorIndex.
*/
public static final class VectorIndexStub
extends io.grpc.stub.AbstractAsyncStub {
private VectorIndexStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected VectorIndexStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new VectorIndexStub(channel, callOptions);
}
/**
*/
public void upsertItemBatch(grpc.vectorindex._UpsertItemBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getUpsertItemBatchMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void deleteItemBatch(grpc.vectorindex._DeleteItemBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDeleteItemBatchMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void search(grpc.vectorindex._SearchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSearchMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void searchAndFetchVectors(grpc.vectorindex._SearchAndFetchVectorsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getSearchAndFetchVectorsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getItemMetadataBatch(grpc.vectorindex._GetItemMetadataBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetItemMetadataBatchMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getItemBatch(grpc.vectorindex._GetItemBatchRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetItemBatchMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void countItems(grpc.vectorindex._CountItemsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCountItemsMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service VectorIndex.
*/
public static final class VectorIndexBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private VectorIndexBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected VectorIndexBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new VectorIndexBlockingStub(channel, callOptions);
}
/**
*/
public grpc.vectorindex._UpsertItemBatchResponse upsertItemBatch(grpc.vectorindex._UpsertItemBatchRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getUpsertItemBatchMethod(), getCallOptions(), request);
}
/**
*/
public grpc.vectorindex._DeleteItemBatchResponse deleteItemBatch(grpc.vectorindex._DeleteItemBatchRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDeleteItemBatchMethod(), getCallOptions(), request);
}
/**
*/
public grpc.vectorindex._SearchResponse search(grpc.vectorindex._SearchRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSearchMethod(), getCallOptions(), request);
}
/**
*/
public grpc.vectorindex._SearchAndFetchVectorsResponse searchAndFetchVectors(grpc.vectorindex._SearchAndFetchVectorsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getSearchAndFetchVectorsMethod(), getCallOptions(), request);
}
/**
*/
public grpc.vectorindex._GetItemMetadataBatchResponse getItemMetadataBatch(grpc.vectorindex._GetItemMetadataBatchRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetItemMetadataBatchMethod(), getCallOptions(), request);
}
/**
*/
public grpc.vectorindex._GetItemBatchResponse getItemBatch(grpc.vectorindex._GetItemBatchRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetItemBatchMethod(), getCallOptions(), request);
}
/**
*/
public grpc.vectorindex._CountItemsResponse countItems(grpc.vectorindex._CountItemsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCountItemsMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service VectorIndex.
*/
public static final class VectorIndexFutureStub
extends io.grpc.stub.AbstractFutureStub {
private VectorIndexFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected VectorIndexFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new VectorIndexFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture upsertItemBatch(
grpc.vectorindex._UpsertItemBatchRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getUpsertItemBatchMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture deleteItemBatch(
grpc.vectorindex._DeleteItemBatchRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDeleteItemBatchMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture search(
grpc.vectorindex._SearchRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSearchMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture searchAndFetchVectors(
grpc.vectorindex._SearchAndFetchVectorsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getSearchAndFetchVectorsMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture getItemMetadataBatch(
grpc.vectorindex._GetItemMetadataBatchRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetItemMetadataBatchMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture getItemBatch(
grpc.vectorindex._GetItemBatchRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetItemBatchMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture countItems(
grpc.vectorindex._CountItemsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCountItemsMethod(), getCallOptions()), request);
}
}
private static final int METHODID_UPSERT_ITEM_BATCH = 0;
private static final int METHODID_DELETE_ITEM_BATCH = 1;
private static final int METHODID_SEARCH = 2;
private static final int METHODID_SEARCH_AND_FETCH_VECTORS = 3;
private static final int METHODID_GET_ITEM_METADATA_BATCH = 4;
private static final int METHODID_GET_ITEM_BATCH = 5;
private static final int METHODID_COUNT_ITEMS = 6;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_UPSERT_ITEM_BATCH:
serviceImpl.upsertItemBatch((grpc.vectorindex._UpsertItemBatchRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DELETE_ITEM_BATCH:
serviceImpl.deleteItemBatch((grpc.vectorindex._DeleteItemBatchRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SEARCH:
serviceImpl.search((grpc.vectorindex._SearchRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SEARCH_AND_FETCH_VECTORS:
serviceImpl.searchAndFetchVectors((grpc.vectorindex._SearchAndFetchVectorsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_ITEM_METADATA_BATCH:
serviceImpl.getItemMetadataBatch((grpc.vectorindex._GetItemMetadataBatchRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_ITEM_BATCH:
serviceImpl.getItemBatch((grpc.vectorindex._GetItemBatchRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_COUNT_ITEMS:
serviceImpl.countItems((grpc.vectorindex._CountItemsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getUpsertItemBatchMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
grpc.vectorindex._UpsertItemBatchRequest,
grpc.vectorindex._UpsertItemBatchResponse>(
service, METHODID_UPSERT_ITEM_BATCH)))
.addMethod(
getDeleteItemBatchMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
grpc.vectorindex._DeleteItemBatchRequest,
grpc.vectorindex._DeleteItemBatchResponse>(
service, METHODID_DELETE_ITEM_BATCH)))
.addMethod(
getSearchMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
grpc.vectorindex._SearchRequest,
grpc.vectorindex._SearchResponse>(
service, METHODID_SEARCH)))
.addMethod(
getSearchAndFetchVectorsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
grpc.vectorindex._SearchAndFetchVectorsRequest,
grpc.vectorindex._SearchAndFetchVectorsResponse>(
service, METHODID_SEARCH_AND_FETCH_VECTORS)))
.addMethod(
getGetItemMetadataBatchMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
grpc.vectorindex._GetItemMetadataBatchRequest,
grpc.vectorindex._GetItemMetadataBatchResponse>(
service, METHODID_GET_ITEM_METADATA_BATCH)))
.addMethod(
getGetItemBatchMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
grpc.vectorindex._GetItemBatchRequest,
grpc.vectorindex._GetItemBatchResponse>(
service, METHODID_GET_ITEM_BATCH)))
.addMethod(
getCountItemsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
grpc.vectorindex._CountItemsRequest,
grpc.vectorindex._CountItemsResponse>(
service, METHODID_COUNT_ITEMS)))
.build();
}
private static abstract class VectorIndexBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
VectorIndexBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return grpc.vectorindex.Vectorindex.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("VectorIndex");
}
}
private static final class VectorIndexFileDescriptorSupplier
extends VectorIndexBaseDescriptorSupplier {
VectorIndexFileDescriptorSupplier() {}
}
private static final class VectorIndexMethodDescriptorSupplier
extends VectorIndexBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
VectorIndexMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (VectorIndexGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new VectorIndexFileDescriptorSupplier())
.addMethod(getUpsertItemBatchMethod())
.addMethod(getDeleteItemBatchMethod())
.addMethod(getSearchMethod())
.addMethod(getSearchAndFetchVectorsMethod())
.addMethod(getGetItemMetadataBatchMethod())
.addMethod(getGetItemBatchMethod())
.addMethod(getCountItemsMethod())
.build();
}
}
}
return result;
}
}