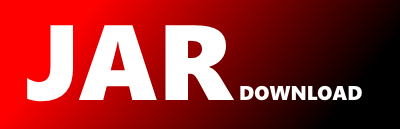
grpc.vectorindex.VectorindexGrpcKt.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of client-protos-jvm Show documentation
Show all versions of client-protos-jvm Show documentation
Kotlin protobuf protocols for the JVM that define the Momento gRPC wire format
package grpc.vectorindex
import grpc.vectorindex.VectorIndexGrpc.getServiceDescriptor
import io.grpc.CallOptions
import io.grpc.CallOptions.DEFAULT
import io.grpc.Channel
import io.grpc.Metadata
import io.grpc.MethodDescriptor
import io.grpc.ServerServiceDefinition
import io.grpc.ServerServiceDefinition.builder
import io.grpc.ServiceDescriptor
import io.grpc.Status.UNIMPLEMENTED
import io.grpc.StatusException
import io.grpc.kotlin.AbstractCoroutineServerImpl
import io.grpc.kotlin.AbstractCoroutineStub
import io.grpc.kotlin.ClientCalls.unaryRpc
import io.grpc.kotlin.ServerCalls.unaryServerMethodDefinition
import io.grpc.kotlin.StubFor
import kotlin.String
import kotlin.coroutines.CoroutineContext
import kotlin.coroutines.EmptyCoroutineContext
import kotlin.jvm.JvmOverloads
import kotlin.jvm.JvmStatic
/**
* Holder for Kotlin coroutine-based client and server APIs for vectorindex.VectorIndex.
*/
public object VectorIndexGrpcKt {
public const val SERVICE_NAME: String = VectorIndexGrpc.SERVICE_NAME
@JvmStatic
public val serviceDescriptor: ServiceDescriptor
get() = getServiceDescriptor()
public val upsertItemBatchMethod:
MethodDescriptor<_UpsertItemBatchRequest, _UpsertItemBatchResponse>
@JvmStatic
get() = VectorIndexGrpc.getUpsertItemBatchMethod()
public val deleteItemBatchMethod:
MethodDescriptor<_DeleteItemBatchRequest, _DeleteItemBatchResponse>
@JvmStatic
get() = VectorIndexGrpc.getDeleteItemBatchMethod()
public val searchMethod: MethodDescriptor<_SearchRequest, _SearchResponse>
@JvmStatic
get() = VectorIndexGrpc.getSearchMethod()
public val searchAndFetchVectorsMethod:
MethodDescriptor<_SearchAndFetchVectorsRequest, _SearchAndFetchVectorsResponse>
@JvmStatic
get() = VectorIndexGrpc.getSearchAndFetchVectorsMethod()
public val getItemMetadataBatchMethod:
MethodDescriptor<_GetItemMetadataBatchRequest, _GetItemMetadataBatchResponse>
@JvmStatic
get() = VectorIndexGrpc.getGetItemMetadataBatchMethod()
public val getItemBatchMethod: MethodDescriptor<_GetItemBatchRequest, _GetItemBatchResponse>
@JvmStatic
get() = VectorIndexGrpc.getGetItemBatchMethod()
public val countItemsMethod: MethodDescriptor<_CountItemsRequest, _CountItemsResponse>
@JvmStatic
get() = VectorIndexGrpc.getCountItemsMethod()
/**
* A stub for issuing RPCs to a(n) vectorindex.VectorIndex service as suspending coroutines.
*/
@StubFor(VectorIndexGrpc::class)
public class VectorIndexCoroutineStub @JvmOverloads constructor(
channel: Channel,
callOptions: CallOptions = DEFAULT,
) : AbstractCoroutineStub(channel, callOptions) {
override fun build(channel: Channel, callOptions: CallOptions): VectorIndexCoroutineStub =
VectorIndexCoroutineStub(channel, callOptions)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun upsertItemBatch(request: _UpsertItemBatchRequest, headers: Metadata =
Metadata()): _UpsertItemBatchResponse = unaryRpc(
channel,
VectorIndexGrpc.getUpsertItemBatchMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun deleteItemBatch(request: _DeleteItemBatchRequest, headers: Metadata =
Metadata()): _DeleteItemBatchResponse = unaryRpc(
channel,
VectorIndexGrpc.getDeleteItemBatchMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun search(request: _SearchRequest, headers: Metadata = Metadata()):
_SearchResponse = unaryRpc(
channel,
VectorIndexGrpc.getSearchMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun searchAndFetchVectors(request: _SearchAndFetchVectorsRequest,
headers: Metadata = Metadata()): _SearchAndFetchVectorsResponse = unaryRpc(
channel,
VectorIndexGrpc.getSearchAndFetchVectorsMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun getItemMetadataBatch(request: _GetItemMetadataBatchRequest, headers: Metadata
= Metadata()): _GetItemMetadataBatchResponse = unaryRpc(
channel,
VectorIndexGrpc.getGetItemMetadataBatchMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun getItemBatch(request: _GetItemBatchRequest, headers: Metadata = Metadata()):
_GetItemBatchResponse = unaryRpc(
channel,
VectorIndexGrpc.getGetItemBatchMethod(),
request,
callOptions,
headers
)
/**
* Executes this RPC and returns the response message, suspending until the RPC completes
* with [`Status.OK`][io.grpc.Status]. If the RPC completes with another status, a
* corresponding
* [StatusException] is thrown. If this coroutine is cancelled, the RPC is also cancelled
* with the corresponding exception as a cause.
*
* @param request The request message to send to the server.
*
* @param headers Metadata to attach to the request. Most users will not need this.
*
* @return The single response from the server.
*/
public suspend fun countItems(request: _CountItemsRequest, headers: Metadata = Metadata()):
_CountItemsResponse = unaryRpc(
channel,
VectorIndexGrpc.getCountItemsMethod(),
request,
callOptions,
headers
)
}
/**
* Skeletal implementation of the vectorindex.VectorIndex service based on Kotlin coroutines.
*/
public abstract class VectorIndexCoroutineImplBase(
coroutineContext: CoroutineContext = EmptyCoroutineContext,
) : AbstractCoroutineServerImpl(coroutineContext) {
/**
* Returns the response to an RPC for vectorindex.VectorIndex.UpsertItemBatch.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun upsertItemBatch(request: _UpsertItemBatchRequest):
_UpsertItemBatchResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method vectorindex.VectorIndex.UpsertItemBatch is unimplemented"))
/**
* Returns the response to an RPC for vectorindex.VectorIndex.DeleteItemBatch.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun deleteItemBatch(request: _DeleteItemBatchRequest):
_DeleteItemBatchResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method vectorindex.VectorIndex.DeleteItemBatch is unimplemented"))
/**
* Returns the response to an RPC for vectorindex.VectorIndex.Search.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun search(request: _SearchRequest): _SearchResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method vectorindex.VectorIndex.Search is unimplemented"))
/**
* Returns the response to an RPC for vectorindex.VectorIndex.SearchAndFetchVectors.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun searchAndFetchVectors(request: _SearchAndFetchVectorsRequest):
_SearchAndFetchVectorsResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method vectorindex.VectorIndex.SearchAndFetchVectors is unimplemented"))
/**
* Returns the response to an RPC for vectorindex.VectorIndex.GetItemMetadataBatch.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun getItemMetadataBatch(request: _GetItemMetadataBatchRequest):
_GetItemMetadataBatchResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method vectorindex.VectorIndex.GetItemMetadataBatch is unimplemented"))
/**
* Returns the response to an RPC for vectorindex.VectorIndex.GetItemBatch.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun getItemBatch(request: _GetItemBatchRequest): _GetItemBatchResponse =
throw
StatusException(UNIMPLEMENTED.withDescription("Method vectorindex.VectorIndex.GetItemBatch is unimplemented"))
/**
* Returns the response to an RPC for vectorindex.VectorIndex.CountItems.
*
* If this method fails with a [StatusException], the RPC will fail with the corresponding
* [io.grpc.Status]. If this method fails with a [java.util.concurrent.CancellationException],
* the RPC will fail
* with status `Status.CANCELLED`. If this method fails for any other reason, the RPC will
* fail with `Status.UNKNOWN` with the exception as a cause.
*
* @param request The request from the client.
*/
public open suspend fun countItems(request: _CountItemsRequest): _CountItemsResponse = throw
StatusException(UNIMPLEMENTED.withDescription("Method vectorindex.VectorIndex.CountItems is unimplemented"))
final override fun bindService(): ServerServiceDefinition = builder(getServiceDescriptor())
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = VectorIndexGrpc.getUpsertItemBatchMethod(),
implementation = ::upsertItemBatch
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = VectorIndexGrpc.getDeleteItemBatchMethod(),
implementation = ::deleteItemBatch
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = VectorIndexGrpc.getSearchMethod(),
implementation = ::search
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = VectorIndexGrpc.getSearchAndFetchVectorsMethod(),
implementation = ::searchAndFetchVectors
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = VectorIndexGrpc.getGetItemMetadataBatchMethod(),
implementation = ::getItemMetadataBatch
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = VectorIndexGrpc.getGetItemBatchMethod(),
implementation = ::getItemBatch
))
.addMethod(unaryServerMethodDefinition(
context = this.context,
descriptor = VectorIndexGrpc.getCountItemsMethod(),
implementation = ::countItems
)).build()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy