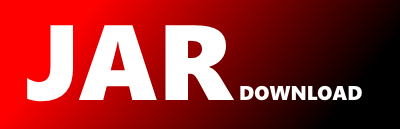
software.xdev.chartjs.model.options.LegendLabels Maven / Gradle / Ivy
/*
* Copyright © 2023 XDEV Software (https://xdev.software)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package software.xdev.chartjs.model.options;
import software.xdev.chartjs.model.enums.FontStyle;
import software.xdev.chartjs.model.javascript.JavaScriptFunction;
public class LegendLabels
{
protected Integer boxWidth;
protected Integer fontSize;
protected FontStyle fontStyle;
protected Object fontColor;
protected String fontFamily;
protected Integer padding;
protected JavaScriptFunction generateLabels;
protected Boolean usePointStyle;
/**
* @see #setBoxWidth(Integer)
*/
public Integer getBoxWidth()
{
return this.boxWidth;
}
/**
*
* Width of coloured box
*
*
*
* Default {@code 40}
*
*/
public LegendLabels setBoxWidth(final Integer boxWidth)
{
this.boxWidth = boxWidth;
return this;
}
/**
* @see #setFontSize(Integer)
*/
public Integer getFontSize()
{
return this.fontSize;
}
/**
*
* Font size inherited from global configuration
*
*
*
* Default {@code 12}
*
*/
public LegendLabels setFontSize(final Integer fontSize)
{
this.fontSize = fontSize;
return this;
}
/**
* @see #setFontStyle(FontStyle)
*/
public FontStyle getFontStyle()
{
return this.fontStyle;
}
/**
*
* Font style inherited from global configuration
*
*
*
* Default {@code "normal"}
*
*/
public LegendLabels setFontStyle(final FontStyle fontStyle)
{
this.fontStyle = fontStyle;
return this;
}
/**
* @see #setFontColor(Object)
*/
public Object getFontColor()
{
return this.fontColor;
}
/**
*
* Font color inherited from global configuration
*
*
*
* Default {@code "#666"}
*
*/
public LegendLabels setFontColor(final Object fontColor)
{
this.fontColor = fontColor;
return this;
}
/**
* @see #setFontFamily(String)
*/
public String getFontFamily()
{
return this.fontFamily;
}
/**
*
* Font family inherited from global configuration
*
*
*
* Default {@code "'Helvetica Neue', 'Helvetica', 'Arial', sans-serif"}
*
*/
public LegendLabels setFontFamily(final String fontFamily)
{
this.fontFamily = fontFamily;
return this;
}
/**
* @see #setPadding(Integer)
*/
public Integer getPadding()
{
return this.padding;
}
/**
*
* Padding between rows of colored boxes
*
*
*
* Default {@code 10}
*
*/
public LegendLabels setPadding(final Integer padding)
{
this.padding = padding;
return this;
}
/**
* @see #setGenerateLabels(JavaScriptFunction)
*/
public JavaScriptFunction getGenerateLabels()
{
return this.generateLabels;
}
/**
*
* Generates legend items for each thing in the legend. Default implementation returns the text + styling for the
* color box. See Legend Item for details.
*
*
*
* Default {@code function(chart) { }}
*
*/
public LegendLabels setGenerateLabels(final JavaScriptFunction generateLabels)
{
this.generateLabels = generateLabels;
return this;
}
/**
* @see #setUsePointStyle(Boolean)
*/
public Boolean getUsePointStyle()
{
return this.usePointStyle;
}
/**
*
* Label style will match corresponding point style (size is based on fontSize, boxWidth is not used in this case).
*
*
*
* Default {@code false}
*
*/
public LegendLabels setUsePointStyle(final Boolean usePointStyle)
{
this.usePointStyle = usePointStyle;
return this;
}
}