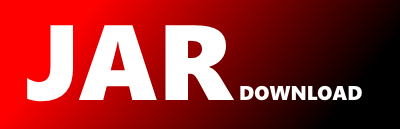
software.xdev.chartjs.model.options.scale.AbstractTickOptions Maven / Gradle / Ivy
/*
* Copyright © 2023 XDEV Software (https://xdev.software)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package software.xdev.chartjs.model.options.scale;
import software.xdev.chartjs.model.javascript.JavaScriptFunction;
import software.xdev.chartjs.model.options.Font;
/**
* Common Tick Options to all axes.
*
* @see ChartJS Docs
* @see ChartJS source
*/
public abstract class AbstractTickOptions>
{
protected Object backdropColor;
protected Object backdropPadding;
protected JavaScriptFunction callback;
protected Boolean display;
protected Object color;
protected Font font;
protected MajorTickConfiguration major;
protected Number padding;
protected Boolean showLabelBackdrop;
protected Object textStrokeColor;
protected Number textStrokeWidth;
protected Number z;
public Object getBackdropColor()
{
return this.backdropColor;
}
public T setBackdropColor(final Object backdropColor)
{
this.backdropColor = backdropColor;
return this.self();
}
public Object getBackdropPadding()
{
return this.backdropPadding;
}
public T setBackdropPadding(final Object backdropPadding)
{
this.backdropPadding = backdropPadding;
return this.self();
}
public JavaScriptFunction getCallback()
{
return this.callback;
}
public T setCallback(final JavaScriptFunction callback)
{
this.callback = callback;
return this.self();
}
public Boolean getDisplay()
{
return this.display;
}
public T setDisplay(final Boolean display)
{
this.display = display;
return this.self();
}
public Object getColor()
{
return this.color;
}
public T setColor(final Object color)
{
this.color = color;
return this.self();
}
public Font getFont()
{
return this.font;
}
public T setFont(final Font font)
{
this.font = font;
return this.self();
}
public MajorTickConfiguration getMajor()
{
return this.major;
}
public T setMajor(final MajorTickConfiguration major)
{
this.major = major;
return this.self();
}
public Number getPadding()
{
return this.padding;
}
public T setPadding(final Number padding)
{
this.padding = padding;
return this.self();
}
public Boolean getShowLabelBackdrop()
{
return this.showLabelBackdrop;
}
public T setShowLabelBackdrop(final Boolean showLabelBackdrop)
{
this.showLabelBackdrop = showLabelBackdrop;
return this.self();
}
public Object getTextStrokeColor()
{
return this.textStrokeColor;
}
public T setTextStrokeColor(final Object textStrokeColor)
{
this.textStrokeColor = textStrokeColor;
return this.self();
}
public Number getTextStrokeWidth()
{
return this.textStrokeWidth;
}
public T setTextStrokeWidth(final Number textStrokeWidth)
{
this.textStrokeWidth = textStrokeWidth;
return this.self();
}
public Number getZ()
{
return this.z;
}
public T setZ(final Number z)
{
this.z = z;
return this.self();
}
@SuppressWarnings("unchecked")
protected T self()
{
return (T)this;
}
/**
* Defines options for the major tick marks that are generated by the axis.
*
* @see ChartJS Docs
* @see ChasrtJS Source
*/
public static class MajorTickConfiguration
{
protected Boolean enabled;
public Boolean getEnabled()
{
return this.enabled;
}
public MajorTickConfiguration setEnabled(final Boolean enabled)
{
this.enabled = enabled;
return this;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy