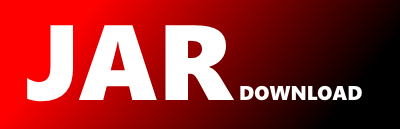
solutions.siren.join.action.coordinate.TransportCoordinateSearchAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of siren-join Show documentation
Show all versions of siren-join Show documentation
SIREn plugin that adds join capabilities to Elasticsearch
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy