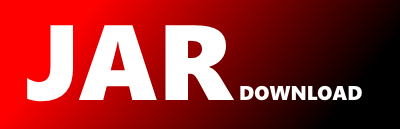
commonMain.space.kscience.dataforge.data.CachingAction.kt Maven / Gradle / Ivy
package space.kscience.dataforge.data
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.coroutineScope
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.collect
import space.kscience.dataforge.actions.Action
import space.kscience.dataforge.meta.Meta
import space.kscience.dataforge.names.Name
import space.kscience.dataforge.names.startsWith
import kotlin.reflect.KType
/**
* Remove all values with keys starting with [name]
*/
internal fun MutableMap.removeWhatStartsWith(name: Name) {
val toRemove = keys.filter { it.startsWith(name) }
toRemove.forEach(::remove)
}
/**
* An action that caches results on-demand and recalculates them on source push
*/
public abstract class CachingAction(
public val outputType: KType,
) : Action {
protected abstract fun CoroutineScope.transform(
set: DataSet,
meta: Meta,
key: Name = Name.EMPTY,
): Flow>
override suspend fun execute(
dataSet: DataSet,
meta: Meta,
scope: CoroutineScope?,
): DataSet = ActiveDataTree(outputType) {
coroutineScope {
populate(transform(dataSet, meta))
}
scope?.let {
dataSet.updates.collect {
//clear old nodes
remove(it)
//collect new items
populate(scope.transform(dataSet, meta, it))
//FIXME if the target is data, updates are fired twice
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy