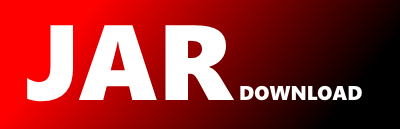
space.maxus.flare.ui.compose.SlideshowImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flare Show documentation
Show all versions of flare Show documentation
Reactive Spigot Inventory UI Library
The newest version!
package space.maxus.flare.ui.compose;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.ToString;
import org.bukkit.inventory.ItemStack;
import space.maxus.flare.Flare;
import space.maxus.flare.item.ItemProvider;
import space.maxus.flare.react.ReactiveState;
import space.maxus.flare.ui.ComposableReactiveState;
import space.maxus.flare.ui.Frame;
import space.maxus.flare.ui.space.Slot;
import space.maxus.flare.util.PausingTask;
import java.util.List;
import java.util.Objects;
import java.util.concurrent.atomic.AtomicInteger;
@ToString
@EqualsAndHashCode(callSuper = true)
final class SlideshowImpl extends RootReferencing implements Slideshow {
private final ReactiveState disabledState;
@Getter
private final List slides;
@Getter
private final int period;
private final AtomicInteger currentIdx;
private final ReactiveState currentSlide;
private PausingTask task;
public SlideshowImpl(List slides, int period, boolean isDisabled) {
this.slides = slides;
this.period = period;
this.disabledState = new ComposableReactiveState<>(isDisabled, this);
this.currentIdx = new AtomicInteger(0);
this.currentSlide = new ComposableReactiveState<>(slides.get(0), this);
this.task = new PausingTask(() -> {
int cur = this.currentIdx.get();
int newIdx = cur + 1 >= slides.size() ? 0 : cur + 1;
this.currentIdx.setRelease(newIdx);
this.currentSlide.set(slides.get(newIdx));
});
this.disabledState.subscribe(disabled -> {
if (Objects.requireNonNullElse(disabled, false))
this.task.pause();
else
this.task.resume();
});
}
@Override
public void injectRoot(Frame root) {
super.injectRoot(root);
// starting task only on root injection
this.task.runTaskTimerAsynchronously(Flare.getInstance(), period, period);
}
@Override
public Slideshow configure(Configurator configurator) {
configurator.configure(this);
return this;
}
@Override
public ReactiveState disabledState() {
return disabledState;
}
@Override
public ItemStack renderAt(Slot slot) {
return currentSlide.get().provide();
}
@Override
public ReactiveState itemState() {
return currentSlide;
}
@Override
public void destroy() {
this.task.cancel();
}
@Override
public void restore() {
this.task = this.task.copy();
this.task.runTaskTimerAsynchronously(Flare.getInstance(), period, period);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy