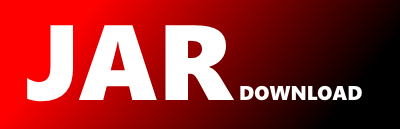
space.vectrix.flare.fastutil.Short2ObjectSyncMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flare-fastutil Show documentation
Show all versions of flare-fastutil Show documentation
Useful thread-safe collections with performance in mind.
/*
* This file is part of flare, licensed under the MIT License (MIT).
*
* Copyright (c) vectrix.space
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package space.vectrix.flare.fastutil;
import it.unimi.dsi.fastutil.shorts.Short2ObjectMap;
import it.unimi.dsi.fastutil.shorts.Short2ObjectOpenHashMap;
import it.unimi.dsi.fastutil.shorts.ShortSet;
import it.unimi.dsi.fastutil.objects.ObjectSet;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.checkerframework.checker.nullness.qual.Nullable;
import java.util.Collections;
import java.util.Map;
import java.util.function.IntFunction;
/**
* A concurrent map, internally backed by a non-thread-safe map but carefully
* managed in a matter such that any changes are thread-safe. Internally, the
* map is split into a {@code read} and a {@code dirty} map. The read map only
* satisfies read requests, while the dirty map satisfies all other requests.
*
* The map is optimized for two common use cases:
*
*
* - The entry for the given map is only written once but read many
* times, as in a cache that only grows.
*
* - Heavy concurrent modification of entries for a disjoint set of
* keys.
*
*
* In both cases, this map significantly reduces lock contention compared
* to a traditional map paired with a read and write lock, along with maps
* with an exclusive lock (such as using {@link Collections#synchronizedMap(Map)}.
*
* Null values are not accepted. Null keys are supported if the backing collection
* supports them.
*
* Based on: https://golang.org/src/sync/map.go
*
* @param the value type
* @since 0.2.0
*/
public interface Short2ObjectSyncMap extends Short2ObjectMap {
/**
* Returns a new sync map, backed by a {@link Short2ObjectOpenHashMap}.
*
* @param the value type
* @return a sync map
* @since 0.2.0
*/
@SuppressWarnings("RedundantTypeArguments")
static @NonNull Short2ObjectSyncMap hashmap() {
return of(Short2ObjectOpenHashMap>::new, 16);
}
/**
* Returns a new sync map, backed by a {@link Short2ObjectOpenHashMap} with a provided initial
* capacity.
*
* @param initialCapacity the initial capacity of the hash map
* @param the value type
* @return a sync map
* @since 0.2.0
*/
@SuppressWarnings("RedundantTypeArguments")
static @NonNull Short2ObjectSyncMap hashmap(final int initialCapacity) {
return of(Short2ObjectOpenHashMap>::new, initialCapacity);
}
/**
* Returns a new sync map, backed by a {@link Short2ObjectOpenHashMap} with a provided initial
* capacity and promotion factor.
*
* @param initialCapacity the initial capacity of the hash map
* @param promotionFactor the promotion factor of the sync map
* @param the value type
* @return a sync map
* @since 0.3.0
*/
@SuppressWarnings("RedundantTypeArguments")
static @NonNull Short2ObjectSyncMap hashmap(final int initialCapacity, final float promotionFactor) {
return of(Short2ObjectOpenHashMap>::new, initialCapacity, promotionFactor);
}
/**
* Returns a new mutable set view of a sync map, backed by a {@link Short2ObjectOpenHashMap}.
*
* @return a mutable set view of a sync map
* @since 0.2.0
*/
@SuppressWarnings("RedundantTypeArguments")
static @NonNull ShortSet hashset() {
return setOf(Short2ObjectOpenHashMap>::new, 16);
}
/**
* Returns a new mutable set view of a sync map, backed by a {@link Short2ObjectOpenHashMap}
* with a provided initial capacity.
*
* @param initialCapacity the initial capacity of the hash map
* @return a mutable set view of a sync map
* @since 0.2.0
*/
@SuppressWarnings("RedundantTypeArguments")
static @NonNull ShortSet hashset(final int initialCapacity) {
return setOf(Short2ObjectOpenHashMap>::new, initialCapacity);
}
/**
* Returns a new mutable set view of a sync map, backed by a {@link Short2ObjectOpenHashMap} with
* a provided initial capacity and promotion factor.
*
* @param initialCapacity the initial capacity of the hash map
* @param promotionFactor the promotion factor of the sync map
* @return a mutable set view of a sync map
* @since 0.3.0
*/
@SuppressWarnings("RedundantTypeArguments")
static @NonNull ShortSet hashset(final int initialCapacity, final float promotionFactor) {
return setOf(Short2ObjectOpenHashMap>::new, initialCapacity, promotionFactor);
}
/**
* Returns a new sync map, backed by the provided {@link Short2ObjectMap}
* implementation with a provided initial capacity.
*
* @param function the map creation function
* @param initialCapacity the map initial capacity
* @param the value type
* @return a sync map
* @since 0.2.0
*/
static @NonNull Short2ObjectSyncMap of(final @NonNull IntFunction>> function, final int initialCapacity) {
return new Short2ObjectSyncMapImpl<>(function, initialCapacity);
}
/**
* Returns a new sync map, backed by the provided {@link Short2ObjectMap}
* implementation with a provided initial capacity and promotion factor.
*
* @param function the map creation function
* @param initialCapacity the map initial capacity
* @param promotionFactor the map promotion factor
* @param the value type
* @return a sync map
* @since 0.3.0
*/
static @NonNull Short2ObjectSyncMap of(final @NonNull IntFunction>> function, final int initialCapacity, final float promotionFactor) {
return new Short2ObjectSyncMapImpl<>(function, initialCapacity, promotionFactor);
}
/**
* Returns a new mutable set view of a sync map, backed by the provided
* {@link Short2ObjectMap} implementation with a provided initial capacity.
*
* @param function the map creation function
* @param initialCapacity the map initial capacity
* @return a mutable set view of a sync map
* @since 0.2.0
*/
static @NonNull ShortSet setOf(final @NonNull IntFunction>> function, final int initialCapacity) {
return new Short2ObjectSyncMapSet(new Short2ObjectSyncMapImpl<>(function, initialCapacity));
}
/**
* Returns a new mutable set view of a sync map, backed by the provided
* {@link Short2ObjectMap} implementation with a provided initial capacity
* and promotion factor.
*
* @param function the map creation function
* @param initialCapacity the map initial capacity
* @param promotionFactor the map promotion factor
* @return a mutable set view of a sync map
* @since 0.3.0
*/
static @NonNull ShortSet setOf(final @NonNull IntFunction>> function, final int initialCapacity, final float promotionFactor) {
return new Short2ObjectSyncMapSet(new Short2ObjectSyncMapImpl<>(function, initialCapacity, promotionFactor));
}
/**
* {@inheritDoc}
*
* Iterations over a sync map are thread-safe, and the keys iterated over will not change for a single iteration
* attempt, however they may not necessarily reflect the state of the map at the time the iterator was created.
*
* Performance Note: If entries have been appended to the map, iterating over the entry set will automatically
* promote them to the read map.
*/
@Override
@NonNull ObjectSet> short2ObjectEntrySet();
/**
* {@inheritDoc}
*
* This implementation is {@code O(n)} in nature due to the need to check for any expunged entries. Likewise, as
* with other concurrent collections, the value obtained by this method may be out of date by the time this method
* returns.
*
* @return the size of all the mappings contained in this map
*/
@Override
int size();
/**
* {@inheritDoc}
*
* This method clears the map by resetting the internal state to a state similar to as if a new map had been created.
* If there are concurrent iterations in progress, they will reflect the state of the map prior to being cleared.
*/
@Override
void clear();
/**
* The expunging value the backing map wraps for its values.
*
* @param the backing value type
* @since 0.2.0
*/
interface ExpungingValue {
/**
* Returns the backing value, which may be {@code null} if it has been expunged.
*
* @return the backing value if it has not been expunged
* @since 0.2.0
*/
@Nullable V get();
/**
* Attempts to place the value into the map if it is absent.
*
* @param value the value to place in the map
* @return {@link Map.Entry} with a {@code false} key and {@code null} value if the element
* was expunged, otherwise a {@code true} key and value from the map
* @since 0.2.0
*/
Map.@NonNull Entry putIfAbsent(final @NonNull V value);
/**
* Returns {@code true} if this element has a value (it is neither expunged nor {@code null}).
*
* @return {@code true} if this entry exists, otherwise {@code false}
* @since 0.2.0
*/
boolean exists();
/**
* Sets the backing value.
*
* @param value the value
* @return the value to be stored
* @since 0.3.0
*/
@NonNull V set(final @NonNull V value);
/**
* Tries to replace the backing value, which can be set to {@code null}. This operation has no effect
* if the entry was expunged.
*
* @param compare the value to compare against
* @param value the new value to be stored
* @return {@code true} if successful, otherwise {@code false}
* @since 0.2.0
*/
boolean replace(final @NonNull Object compare, final @Nullable V value);
/**
* Clears the value stored in this entry. Has no effect if {@code null} is stored in the map or
* the entry was expunged.
*
* @return the previous element stored, or {@code null} if the entry had been expunged
* @since 0.2.0
*/
@Nullable V clear();
/**
* Tries to set the backing element. If the entry is expunged, this operation
* will fail.
*
* @param value the new value
* @return {@code true} if the entry was not expunged, {@code false} otherwise
* @since 0.2.0
*/
boolean trySet(final @NonNull V value);
/**
* Tries to mark the entry as expunged if its value is {@code null}.
*
* @return whether or not the item has been expunged
* @since 0.2.0
*/
boolean tryMarkExpunged();
/**
* Tries to set the backing value, which can be set to {@code null}, if the entry was expunged.
*
* @param value the new value
* @return {@code true} if the entry was unexpunged, otherwise {@code false}
* @since 0.2.0
*/
boolean tryUnexpungeAndSet(final @Nullable V value);
/**
* Tries to unexpunge the backing value, if the entry was previously expunged.
*
* @return {@code true} if the entry was unexpunged, otherwise {@code false}
* @since 0.3.0
*/
boolean tryUnexpunge();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy