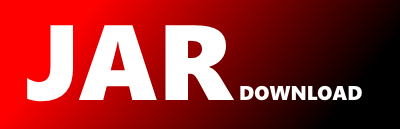
space.vectrix.flare.fastutil.Short2ObjectSyncMapSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flare-fastutil Show documentation
Show all versions of flare-fastutil Show documentation
Useful thread-safe collections with performance in mind.
/*
* This file is part of flare, licensed under the MIT License (MIT).
*
* Copyright (c) vectrix.space
* Copyright (c) contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package space.vectrix.flare.fastutil;
import it.unimi.dsi.fastutil.shorts.AbstractShortSet;
import it.unimi.dsi.fastutil.shorts.ShortCollection;
import it.unimi.dsi.fastutil.shorts.ShortIterator;
import it.unimi.dsi.fastutil.shorts.ShortSet;
import it.unimi.dsi.fastutil.shorts.ShortSpliterator;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.checkerframework.checker.nullness.qual.Nullable;
/* package */ final class Short2ObjectSyncMapSet extends AbstractShortSet implements ShortSet {
private static final long serialVersionUID = 1;
private final Short2ObjectSyncMap map;
private final ShortSet set;
/* package */ Short2ObjectSyncMapSet(final @NonNull Short2ObjectSyncMap map) {
this.map = map;
this.set = map.keySet();
}
@Override
public void clear() {
this.map.clear();
}
@Override
public int size() {
return this.map.size();
}
@Override
public boolean isEmpty() {
return this.map.isEmpty();
}
@Override
public boolean contains(final short key) {
return this.map.containsKey(key);
}
@Override
public boolean remove(final short key) {
return this.map.remove(key) != null;
}
@Override
public boolean add(final short key) {
return this.map.put(key, Boolean.TRUE) == null;
}
@Override
public boolean containsAll(final @NonNull ShortCollection collection) {
return this.set.containsAll(collection);
}
@Override
public boolean removeAll(final @NonNull ShortCollection collection) {
return this.set.removeAll(collection);
}
@Override
public boolean retainAll(final @NonNull ShortCollection collection) {
return this.set.retainAll(collection);
}
@Override
public @NonNull ShortIterator iterator() {
return this.set.iterator();
}
@Override
public @NonNull ShortSpliterator spliterator() {
return this.set.spliterator();
}
@Override
public short[] toArray(short[] original) {
return this.set.toArray(original);
}
@Override
public short[] toShortArray() {
return this.set.toShortArray();
}
@Override
public boolean equals(final @Nullable Object other) {
return other == this || this.set.equals(other);
}
@Override
public @NonNull String toString() {
return this.set.toString();
}
@Override
public int hashCode() {
return this.set.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy