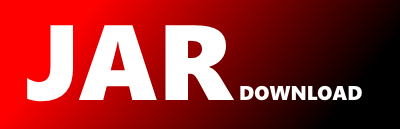
bionic.js.Lambda Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bionic-js Show documentation
Show all versions of bionic-js Show documentation
The library to integrate Bionic JS in Java applications
package bionic.js;
/**
* A wrapper interface for function types.
* */
public interface Lambda
{
/**
* A generic function.
* */
interface Function
{
}
/**
* A function which takes no arguments.
*
* @param the return type of the function
* */
interface F0 extends Function
{
O apply();
}
/**
* A function which takes 1 argument.
*
* @param the type of the first argument of the function
* @param the return type of the function
* */
interface F1 extends Function
{
O apply(I1 i1);
}
/**
* A function which takes 2 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the return type of the function
* */
interface F2 extends Function
{
O apply(I1 i1, I2 i2);
}
/**
* A function which takes 3 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the third argument of the function
* @param the return type of the function
* */
interface F3 extends Function
{
O apply(I1 i1, I2 i2, I3 i3);
}
/**
* A function which takes 4 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the third argument of the function
* @param the type of the forth argument of the function
* @param the return type of the function
* */
interface F4 extends Function
{
O apply(I1 i1, I2 i2, I3 i3, I4 i4);
}
/**
* A function which takes 5 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the third argument of the function
* @param the type of the forth argument of the function
* @param the type of the fifth argument of the function
* @param the return type of the function
* */
interface F5 extends Function
{
O apply(I1 i1, I2 i2, I3 i3, I4 i4, I5 i5);
}
/**
* A function which takes 6 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the third argument of the function
* @param the type of the forth argument of the function
* @param the type of the fifth argument of the function
* @param the type of the sixth argument of the function
* @param the return type of the function
* */
interface F6 extends Function
{
O apply(I1 i1, I2 i2, I3 i3, I4 i4, I5 i5, I6 i6);
}
/**
* A function which takes 7 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the third argument of the function
* @param the type of the forth argument of the function
* @param the type of the fifth argument of the function
* @param the type of the sixth argument of the function
* @param the type of the seventh argument of the function
* @param the return type of the function
* */
interface F7 extends Function
{
O apply(I1 i1, I2 i2, I3 i3, I4 i4, I5 i5, I6 i6, I7 i7);
}
/**
* A function which takes 8 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the third argument of the function
* @param the type of the forth argument of the function
* @param the type of the fifth argument of the function
* @param the type of the sixth argument of the function
* @param the type of the seventh argument of the function
* @param the type of the eighth argument of the function
* @param the return type of the function
* */
interface F8 extends Function
{
O apply(I1 i1, I2 i2, I3 i3, I4 i4, I5 i5, I6 i6, I7 i7, I8 i8);
}
/**
* A function which takes 9 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the third argument of the function
* @param the type of the forth argument of the function
* @param the type of the fifth argument of the function
* @param the type of the sixth argument of the function
* @param the type of the seventh argument of the function
* @param the type of the eighth argument of the function
* @param the type of the ninth argument of the function
* @param the return type of the function
* */
interface F9 extends Function
{
O apply(I1 i1, I2 i2, I3 i3, I4 i4, I5 i5, I6 i6, I7 i7, I8 i8, I9 i9);
}
/**
* A function which takes 10 arguments.
*
* @param the type of the first argument of the function
* @param the type of the second argument of the function
* @param the type of the third argument of the function
* @param the type of the forth argument of the function
* @param the type of the fifth argument of the function
* @param the type of the sixth argument of the function
* @param the type of the seventh argument of the function
* @param the type of the eighth argument of the function
* @param the type of the ninth argument of the function
* @param the type of the tenth argument of the function
* @param the return type of the function
* */
interface F10 extends Function
{
O apply(I1 i1, I2 i2, I3 i3, I4 i4, I5 i5, I6 i6, I7 i7, I8 i8, I9 i9, I10 i10);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy