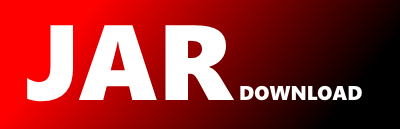
su.nlq.prometheus.jmx.Configuration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmx-prometheus-collector Show documentation
Show all versions of jmx-prometheus-collector Show documentation
Pipe for JMX MBeans to Prometheus
package su.nlq.prometheus.jmx;
import org.jetbrains.annotations.NotNull;
import su.nlq.prometheus.jmx.connection.Connection;
import su.nlq.prometheus.jmx.connection.local.LocalConnection;
import su.nlq.prometheus.jmx.connection.local.LocalConnectionConfiguration;
import su.nlq.prometheus.jmx.connection.remote.jmxmp.JMXMPConnectionConfiguration;
import su.nlq.prometheus.jmx.connection.remote.rmi.RMIConnectionConfiguration;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.annotation.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.function.Supplier;
import java.util.stream.Collectors;
@XmlAccessorOrder
@XmlRootElement(name = "configuration")
public final class Configuration {
@XmlElementWrapper(name = "connections")
@XmlElements({
@XmlElement(name = "local", type = LocalConnectionConfiguration.class),
@XmlElement(name = "jmxmp", type = JMXMPConnectionConfiguration.class),
@XmlElement(name = "rmi", type = RMIConnectionConfiguration.class)
})
private @NotNull List> connections = new ArrayList<>(Arrays.asList(LocalConnection::new));
@XmlElementWrapper(name = "whitelist")
@XmlElement(name = "name")
private @NotNull List whitelist = new ArrayList<>();
@XmlElementWrapper(name = "blacklist")
@XmlElement(name = "name")
private @NotNull List blacklist = new ArrayList<>();
public static @NotNull Configuration parse(@NotNull File file) throws IOException, JAXBException {
try (final InputStream input = new FileInputStream(file)) {
return (Configuration) unmarshaller(Configuration.class).unmarshal(input);
}
}
public @NotNull Iterable connections() {
return connections.stream().map(Supplier::get).collect(Collectors.toList());
}
public @NotNull List whitelist() {
return whitelist;
}
public @NotNull List blacklist() {
return blacklist;
}
private static @NotNull Unmarshaller unmarshaller(@NotNull Class aClass) throws JAXBException {
final JAXBContext context = JAXBContext.newInstance(aClass);
final Unmarshaller unmarshaller = context.createUnmarshaller();
SchemaGenerator.load(context).ifPresent(unmarshaller::setSchema);
return unmarshaller;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy